
RoboCup Base Station
Dependencies: mbed mbed-rtos Wireless Drivers
main.cpp
00001 // RoboCup dual-frequency band base station 00002 00003 #include "BaseStation.h" 00004 00005 uint8_t channel; 00006 00007 // Function for writing a number to the 7-segment display 00008 void writeSegment(uint8_t val, DigitalOut& latch) 00009 { 00010 // Outputs used as input values to the 7-segment binary decoder - uses latching inputs 00011 DigitalOut signal[4] = { RJ_7_SEG_PINS }; 00012 00013 // write out the new value 00014 for (int i=0; i<4; i++) 00015 signal[i] = ((1<<i) & (val)) & 0x0F; 00016 00017 // latch the value 00018 for (int i=0; i<2; i++) 00019 latch = !latch; 00020 } 00021 00022 void seg_task(void const *arg) 00023 { 00024 // latch pin for 7-seg 00025 DigitalOut latch( RJ_7_SEG_LATCH_PIN, 0 ); 00026 00027 // Decimal point initialized to OFF 00028 DigitalOut decimal( RJ_7_SEG_DOT_PIN, 1 ); 00029 00030 channel = 8; 00031 writeSegment(channel, latch); 00032 00033 // wait to be signaled before beginning 00034 osSignalWait(0x01, osWaitForever); 00035 00036 // give a small delay to ensure the startup value stays lit for some time 00037 Thread::wait(500); 00038 00039 // turn the decimal point off 00040 decimal = 0; 00041 00042 while(1) { // loop forever 00043 // send numerical value to 7-segment & hold for a while 00044 writeSegment(channel, latch); 00045 // channel = (channel > 9) ? 0 : channel; // reset value if too high 00046 Thread::wait(1000); 00047 } 00048 } 00049 00050 int main() 00051 { 00052 // RGB Status LED 00053 PwmOut rgb_led[3] = { RJ_RGB_LED_PINS }; 00054 00055 // Primary radio status LEDs 00056 DigitalOut r1_led[3] = { RJ_PRIMARY_RADIO_LEDS }; 00057 00058 // Secondary radio status LEDs 00059 DigitalOut r2_led[3] = { RJ_SECONDARY_RADIO_LEDS }; 00060 00061 // Used for controlling power to the RGB LED's shared annode lead 00062 DigitalOut rgb_pwr( RJ_RGB_LED_ANNODE, 0 ); 00063 00064 // Start 7-segment task 00065 Thread thread_seg_task(seg_task); 00066 00067 // turn all LEDs off initially - values are inverted since LEDs are sinking the current 00068 for (int i=0; i<3; i++) { 00069 rgb_led[i] = 1; 00070 r1_led[i] = 1; 00071 r2_led[i] = 1; 00072 } 00073 00074 // =========== Cyle primary & secondary radio status LEDs =========== 00075 // turn on all radio status LEDs 00076 for (int i=0; i<3; i++) { 00077 00078 // initialze off at the start of every iteration 00079 for(int j=0; j<3; j++) { 00080 r1_led[j] = 1; 00081 r2_led[j] = 1; 00082 } 00083 00084 if (i != 2) { 00085 // cycle 2 LEDs 00086 for (int j=i; j<2; j++) { 00087 r1_led[j] = 0; 00088 r2_led[j] = 0; 00089 Thread::wait(50); 00090 } 00091 } else { 00092 r1_led[i] = 0; 00093 r2_led[i] = 0; 00094 Thread::wait(50); 00095 r1_led[i] = 1; 00096 r2_led[i] = 1; 00097 } 00098 } 00099 00100 // give power to all colors of the RGB LED and turn off decimal point 00101 rgb_pwr = 1; 00102 00103 // 915MHz band radio object 00104 CC1101 cc1101( 00105 RJ_SPI_PINS, 00106 RJ_PRIMARY_RADIO_CS_PIN, 00107 RJ_PRIMARY_RADIO_LED_TX, 00108 RJ_PRIMARY_RADIO_LED_RX, 00109 RJ_PRIMARY_RADIO_INT_PIN 00110 ); 00111 00112 // set pointer for base class calls 00113 Radio *radio_p = &cc1101; 00114 00115 channel = radio_p->channel(); 00116 // tell the segment thread to begin its task 00117 thread_seg_task.signal_set(0x01); 00118 00119 uint8_t color; 00120 if (radio_p->hasError()) { 00121 color = 0; 00122 } else { 00123 color = 1; 00124 } 00125 00126 // fade the RGB LED up to the right status color at half power 00127 for (float i=1.0; i>0.5; i-=0.01) { 00128 rgb_led[color] = i; 00129 Thread::wait(20); 00130 } 00131 00132 00133 00134 // loop forever ==================== 00135 while(1) { 00136 00137 // 00138 00139 // delay 00140 Thread::wait(300); 00141 } 00142 }
Generated on Sun Jul 17 2022 12:51:08 by
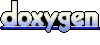