
Program reads temperature from internal thermo meter and voltage on A0. Both values are displayed on LCD
Dependencies: Freetronics_16x2_LCD mbed
Fork of Freetronics_16x2_LCD by
main.cpp
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "mbed.h" 00020 #include "freetronicsLCDShield.h" 00021 00022 Serial pc(SERIAL_TX, SERIAL_RX); 00023 00024 DigitalOut myled(LED1); 00025 AnalogIn analog_value(A0); 00026 00027 #define MV(x) ((0xFFF*x)/3300) 00028 #define V25 1.398 00029 #define SLOPE 0.0043 00030 00031 int main() 00032 { 00033 wait(0.1); 00034 00035 freetronicsLCDShield lcd(D6, D7, D2, D3, D4, D5, D8, A1); 00036 // turn on the back light (it's off by default) 00037 lcd.setBackLight(true); 00038 00039 00040 00041 00042 00043 00044 00045 // print the first line and wait 3 sec 00046 lcd.cls(); 00047 00048 int i = 1; 00049 float meas=0; 00050 float LP=0.025; 00051 float LPT=0.0025; 00052 float temp=0; 00053 lcd.setCursorPosition(0, 0); 00054 lcd.printf("Napeti"); 00055 ADC1->CR2|=ADC_CR2_TSVREFE; 00056 while(1) { 00057 meas = LP*analog_value.read()+(1-LP)*meas; // Converts and read the analog input value 00058 00059 ADC_RegularChannelConfig(ADC1, 16, 1, ADC_SampleTime_7Cycles5); 00060 00061 //// Temperature (in °C) = {(V25 - VSENSE) / Avg_Slope} + 25. 00062 //v25 = 1.43 00063 //avg_slope 4.3mv/c 00064 00065 ADC_SoftwareStartConvCmd(ADC1, ENABLE); // Start conversion 00066 00067 while (ADC_GetFlagStatus(ADC1, ADC_FLAG_EOC) == RESET); // Wait end of conversion 00068 00069 temp= LPT*((V25-(float)(ADC_GetConversionValue(ADC1))*3.3/4096)/SLOPE+25)+(1-LPT)*temp; // Get conversion value 00070 00071 00072 if(meas > 0.5) { // If the value is greater than 1000 mV toggle the LED 00073 myled = 1; 00074 } else { 00075 myled = 0; 00076 } 00077 i++; 00078 if(i==200) { 00079 i=0; 00080 lcd.setCursorPosition(0, 0); 00081 lcd.printf("%2.2f C ", temp); 00082 lcd.setCursorPosition(1, 0); 00083 lcd.printf("%1.4f V", meas*3.3); 00084 } 00085 wait(0.001); 00086 } 00087 }
Generated on Sun Jul 31 2022 08:20:11 by
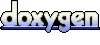