
plays sound via SD card
Dependencies: mbed wave_player mbed-rtos C12832_lcd 4DGL-uLCD-SE LCD_fonts SDFileSystem
main.cpp
00001 // example to test the mbed Lab Board lcd lib with the mbed rtos 00002 // Pot1 changes the contrast 00003 // Pot2 changes the speed of the sin wave 00004 00005 #include "mbed.h" 00006 #include "rtos.h" 00007 #include "stdio.h" 00008 #include "SDFileSystem.h" 00009 #include "wave_player.h" 00010 00011 DigitalOut led1(LED1); 00012 DigitalOut led2(LED2); 00013 DigitalOut led3(LED3); 00014 00015 SDFileSystem sd(p5, p6, p7, p8, "sd"); 00016 AnalogOut DACout(p18); 00017 wave_player waver(&DACout); 00018 00019 // mutex to make the lcd lib thread safe 00020 Mutex spk_mutex; 00021 00022 00023 // Thread 6 00024 // Speaker 00025 void thread6(void const *args) 00026 { 00027 FILE *wave_file; 00028 while(true) { // thread loop 00029 00030 spk_mutex.lock(); 00031 wave_file=fopen("/sd/sound01.wav","r"); 00032 spk_mutex.unlock(); 00033 00034 waver.play(wave_file); 00035 00036 spk_mutex.lock(); 00037 fclose(wave_file); 00038 spk_mutex.unlock(); 00039 00040 //Thread::wait(500); // wait 00041 } 00042 } 00043 00044 00045 int main() 00046 { 00047 00048 Thread t6(thread6); //start thread6 00049 00050 while(true) { // main is the next thread 00051 00052 //Thread::wait(1000); // wait 0.5s 00053 00054 } 00055 00056 }
Generated on Sun Jul 24 2022 14:45:48 by
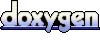