
Arianna autonomous DAQ firmware
Dependencies: mbed SDFileSystemFilinfo AriSnProtocol NetServicesMin AriSnComm MODSERIAL PowerControlClkPatch DS1820OW
Websocket.h
00001 /** 00002 * @author Samuel Mokrani 00003 * 00004 * @section LICENSE 00005 * 00006 * Copyright (c) 2011 mbed 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * 00026 * @section DESCRIPTION 00027 * Simple websocket client 00028 * 00029 */ 00030 00031 #ifndef WEBSOCKET_H 00032 #define WEBSOCKET_H 00033 00034 #ifdef IGNORE_THIS_FILE 00035 00036 #include "mbed.h" 00037 //#include "Wifly.h" 00038 #include <string> 00039 00040 00041 #ifdef TARGET_LPC1768 00042 #include "EthernetNetIf.h" 00043 #include "TCPSocket.h" 00044 #include "dnsresolve.h" 00045 #endif //target 00046 00047 #define RX_BUF_SIZE 1024 00048 #define RX_RECV_BYTES 512 00049 00050 /** Websocket client Class. 00051 * 00052 * Warning: you must use a wifi module (Wifly RN131-C) or an ethernet network to use this class 00053 * 00054 * Example (wifi network): 00055 * @code 00056 * #include "mbed.h" 00057 * #include "Wifly.h" 00058 * #include "Websocket.h" 00059 * 00060 * DigitalOut l1(LED1); 00061 * 00062 * //Here, we create an instance, with pins 9 and 10 connecting to the 00063 * //WiFly's TX and RX pins, and pin 21 to RESET. We are connecting to the 00064 * //"mbed" network, password "password", and we are using WPA. 00065 * Wifly wifly(p9, p10, p21, "mbed", "password", true); 00066 * 00067 * //Here, we create a Websocket instance in 'wo' (write-only) mode 00068 * //on the 'samux' channel 00069 * Websocket ws("ws://sockets.mbed.org/ws/samux/wo", &wifly); 00070 * 00071 * 00072 * int main() { 00073 * while (1) { 00074 * 00075 * //we connect the network 00076 * while (!wifly.join()) { 00077 * wifly.reset(); 00078 * } 00079 * 00080 * //we connect to the websocket server 00081 * while (!ws.connect()); 00082 * 00083 * while (1) { 00084 * wait(0.5); 00085 * 00086 * //Send Hello world 00087 * ws.send("Hello World! over Wifi"); 00088 * 00089 * // show that we are alive 00090 * l1 = !l1; 00091 * } 00092 * } 00093 * } 00094 * @endcode 00095 * 00096 * 00097 * 00098 * Example (ethernet network): 00099 * @code 00100 * #include "mbed.h" 00101 * #include "Websocket.h" 00102 * 00103 * Timer tmr; 00104 * 00105 * //Here, we create a Websocket instance in 'wo' (write-only) mode 00106 * //on the 'samux' channel 00107 * Websocket ws("ws://sockets.mbed.org/ws/samux/wo"); 00108 * 00109 * int main() { 00110 * while (1) { 00111 * 00112 * while (!ws.connect()); 00113 * 00114 * tmr.start(); 00115 * while (1) { 00116 * if (tmr.read() > 0.5) { 00117 * ws.send("Hello World! over Ethernet"); 00118 * tmr.start(); 00119 * } 00120 * Net::poll(); 00121 * } 00122 * } 00123 * } 00124 * @endcode 00125 */ 00126 class Websocket 00127 { 00128 public: 00129 /** 00130 * Constructor 00131 * 00132 * @param url The Websocket url in the form "ws://ip_domain[:port]/path" (by default: port = 80) 00133 * @param wifi pointer to a wifi module (the communication will be establish by this module) 00134 */ 00135 // Websocket(char * url, Wifly * wifi); 00136 00137 #ifdef TARGET_LPC1768 00138 /** 00139 * Constructor for an ethernet communication 00140 * 00141 * @param url The Websocket url in the form "ws://ip_domain[:port]/path" (by default: port = 80) 00142 */ 00143 Websocket(char * url); 00144 #endif //target 00145 00146 /** 00147 * Connect to the websocket url 00148 * 00149 *@return true if the connection is established, false otherwise 00150 */ 00151 bool connect(const uint32_t timeout); 00152 00153 /** 00154 * Send a string according to the websocket format 00155 * 00156 * @param str string to be sent 00157 */ 00158 void send(const char * str); 00159 00160 // CJR: add ones for binary data 00161 bool sendBinary(const char* str, const uint32_t len, char* const bbuf); 00162 bool sendBinary(const char* hbuf, const uint32_t hlen, FILE* f, const uint32_t nbytes, 00163 char* const bbuf); 00164 00165 /** 00166 * Read a websocket message 00167 * 00168 * @param message pointer to the string to be read (null if drop frame) 00169 * 00170 * @return true if a string has been read, false otherwise 00171 */ 00172 bool read(char * message, uint32_t& len_msg, const uint32_t maxlen, 00173 const uint32_t timeout, 00174 char* const bbuf, const uint32_t bbsize); 00175 00176 /** 00177 * To see if there is a websocket connection active 00178 * 00179 * @return true if there is a connection active 00180 */ 00181 bool connected(); 00182 00183 /** 00184 * Close the websocket connection 00185 * 00186 * @return true if the connection has been closed, false otherwise 00187 */ 00188 bool close(); 00189 00190 /** 00191 * Accessor: get path from the websocket url 00192 * 00193 * @return path 00194 */ 00195 std::string getPath(); 00196 00197 enum Type { 00198 WIF, 00199 ETH 00200 }; 00201 00202 00203 private: 00204 00205 bool sendBinaryDirect(const char* str, const uint32_t len); 00206 bool sendBinaryDirect(const char* hbuf, const uint32_t hlen, FILE* f, const uint32_t nbytes); 00207 bool sendBinaryB64txt(const char* str, const uint32_t len, char* const bbuf); 00208 bool sendBinaryB64txt(const char* hbuf, const uint32_t hlen, FILE* f, const uint32_t nbytes, 00209 char* const bbuf); 00210 00211 void fillFields(char * url); 00212 void sendOpcode(uint8_t opcode); 00213 void sendLength(uint32_t len); 00214 void sendMask(); 00215 int sendChar(uint8_t c); 00216 00217 std::string ip_domain; 00218 std::string path; 00219 std::string port; 00220 00221 // Wifly * wifi; 00222 00223 #ifdef TARGET_LPC1768 00224 void onTCPSocketEvent(TCPSocketEvent e); 00225 bool eth_connected; 00226 bool eth_readable; 00227 bool eth_writeable; 00228 char eth_rx[RX_BUF_SIZE]; 00229 bool response_server_eth; 00230 bool new_msg; 00231 00232 EthernetNetIf * eth; 00233 TCPSocket * sock; 00234 IpAddr * server_ip; 00235 #endif //target 00236 00237 Type netif; 00238 00239 static const bool kUseB64; 00240 }; 00241 00242 #endif 00243 00244 #endif
Generated on Fri Jul 15 2022 00:05:34 by
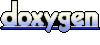