
Arianna autonomous DAQ firmware
Dependencies: mbed SDFileSystemFilinfo AriSnProtocol NetServicesMin AriSnComm MODSERIAL PowerControlClkPatch DS1820OW
SnCommWin.h
00001 #ifndef SN_SnCommWin 00002 #define SN_SnCommWin 00003 00004 #include "mbed.h" 00005 #include <stdint.h> 00006 #include <string> 00007 00008 #include "SnConfigFrame.h" 00009 class SnEventFrame; 00010 class SnPowerFrame; 00011 class SnSignalStrengthFrame; 00012 class SnCommPeripheral; 00013 class SnTempFrame; 00014 class SnClockSetFrame; 00015 class SnHeartbeatFrame; 00016 00017 // DAQ station communication utilities 00018 00019 class SnCommWin { 00020 public: 00021 enum ECommWinResult { 00022 kUndefFail, // undefined fail type 00023 kCanNotConnect, // unable to connect to port 00024 kFailTimeout, // timed out but message required 00025 kFailNoneSent, // none of the message sent 00026 kFailPartSent, // only part of the message sent 00027 kUnexpectedRec, // unexpected / unhandled message received 00028 kAllFails, // to be used for "if (ret > kAllFails) ==> success" 00029 // only successes should go below 00030 kConnected, // connection established, no messaging attempted 00031 kOkStopComm, // received signal to stop communicating 00032 kOkMsgSent, // message sent without waiting for "received" handshake 00033 kOkNoMsg, // timed out with no message, but that is ok 00034 kOkWithMsg, // successfully received message 00035 kOkWthMsgNoConf,// successfully received message and it says to stick with the same config 00036 kOkWthMsgDidDel // successfully received message and deleted a file because of it 00037 }; 00038 00039 static const char* kLocalDir; // the local mbed directory 00040 static const char* kDelAllConfCodeStr; // a magic string to confirm deletion of all files on the SD card 00041 static const uint8_t kDelAllConfCodeLen; // the length of the magic string 00042 00043 private: 00044 bool fSendingInHandshake; // true if we are sending files as a result of a handshake. 00045 // will ignore further handshake requests to send new files 00046 // in order to avoid a stack overflow 00047 00048 00049 SnCommWin::ECommWinResult GetFilename(const uint32_t timeout, 00050 char* const buf, 00051 const uint32_t namelen); 00052 bool BuildLocalFileName(std::string fname, 00053 const char* const dir, 00054 std::string& lfname); 00055 SnCommWin::ECommWinResult GetLocalFile(std::string fname, 00056 char* const buf, 00057 const uint32_t bsize, 00058 const uint32_t timeout, 00059 std::string& lfname); 00060 int16_t LoopLocalDirBinFiles(const bool doRemove, 00061 const std::string& fname); 00062 SnCommWin::ECommWinResult GetHeader(const uint32_t timeOut, 00063 char* const buf, 00064 const uint32_t bsize, 00065 uint8_t& mcode, 00066 uint32_t& mlen); 00067 00068 bool WriteStatusDataPackHeaderTo(char*& b, 00069 const uint32_t payloadLen); 00070 00071 bool CallHeaderWriteTo(char*& b, 00072 const uint8_t msgCode, 00073 const uint32_t msgLen); 00074 00075 int32_t CallSendAll(const char* const data, const uint32_t length, 00076 const uint32_t timeout_clock); 00077 00078 00079 template<class T> 00080 char* WriteStatusDataPackHeadersFor(const T& x, 00081 const uint8_t hdrCode, 00082 char*& b, 00083 const uint32_t timeout_clock) { 00084 // flag that this is a data packet 00085 const uint32_t xMsgLen = x.SizeOf(); 00086 WriteStatusDataPackHeaderTo(b, xMsgLen); 00087 CallHeaderWriteTo(b, hdrCode, xMsgLen); 00088 return b; 00089 } 00090 00091 template<class T> 00092 char* SendStatusDataPack(const T& x, 00093 const uint8_t hdrCode, 00094 int32_t& toBeSent, 00095 int32_t& byteSent, 00096 char* const genBuf, 00097 const uint32_t timeout_clock) { 00098 char* b = genBuf; 00099 b = WriteStatusDataPackHeadersFor(x, hdrCode, 00100 b, timeout_clock); 00101 x.WriteTo(b); 00102 const int32_t sendNow = (b - genBuf); 00103 toBeSent += sendNow; 00104 byteSent += CallSendAll(genBuf, sendNow, timeout_clock); 00105 return b; 00106 } 00107 00108 00109 protected: 00110 SnCommPeripheral* fComm; // the communication peripheral. deleted in dtor!! 00111 00112 virtual int32_t SendFileBlock(FILE* inf, 00113 const uint8_t blockHeaderCode, 00114 const uint32_t blockSize, 00115 char* const genBuf, 00116 int32_t& bytesToSend, 00117 const uint32_t timeout); 00118 00119 virtual int32_t SendFileContents(FILE* inf, 00120 const SnConfigFrame& curConf, 00121 SnEventFrame& evt, 00122 SnPowerFrame& pow, 00123 char* const genBuf, 00124 uint32_t nevts, 00125 int32_t& bytesToBeSent, 00126 const uint32_t timeout_clock); 00127 00128 public: 00129 SnCommWin(SnCommPeripheral* p); 00130 virtual ~SnCommWin(); 00131 00132 // probably no need to overload 00133 virtual bool TrySetSysTimeUnix(const uint32_t timeout, 00134 uint32_t& prvTime, 00135 uint32_t& setTime); 00136 virtual bool Connect(const uint32_t timeout); 00137 virtual bool CloseConn(const uint32_t timeout, 00138 char* const genBuf=0, 00139 const bool sendCloseSignal=false); 00140 virtual bool PowerDown(const uint32_t timeout); 00141 00142 // optional overloads 00143 virtual void Set(const SnConfigFrame& conf) {} 00144 00145 // mandatory overloads 00146 virtual SnConfigFrame::EDatPackBit GetCommType() const=0; 00147 00148 virtual ECommWinResult OpenWindow(const bool sendStatus, 00149 const SnConfigFrame& conf, 00150 const SnPowerFrame& pow, // com win power 00151 const SnEventFrame& stEvent, 00152 const uint16_t seq, 00153 // const float thmrate, 00154 // const float evtrate, 00155 const uint32_t numThmTrigs, 00156 const uint32_t numSavedEvts, 00157 const float seqlive, 00158 const uint32_t powerOnTime, 00159 const SnTempFrame& temper, // com win temp 00160 char* const genBuf, 00161 const uint32_t timeout_clock)=0; 00162 00163 virtual bool GetDeleteAllConfirmCode(const SnConfigFrame& conf, 00164 const uint32_t length, 00165 const uint32_t timeout, 00166 char* const buf, 00167 const uint32_t bsize); 00168 virtual ECommWinResult WaitHandshake(const SnConfigFrame& conf, 00169 const uint32_t timeout, 00170 char* const buf, 00171 const uint32_t bsize, 00172 uint8_t& hndShkCode, 00173 uint32_t* hndShkLen=0); 00174 00175 virtual ECommWinResult HandleHandshake(FILE* inf, const char* infn, 00176 const SnConfigFrame& curConf, 00177 SnEventFrame& evt, 00178 SnPowerFrame& pow, 00179 char* const genBuf, 00180 const uint32_t bsize, 00181 const uint32_t handshakeTimeout, 00182 const uint8_t hndshk, 00183 const uint32_t hndlen, 00184 const uint32_t* nevts=0); 00185 int32_t SendHndshkReq(char* const genBuf, 00186 const uint32_t timeout_clock); 00187 00188 ECommWinResult SendSignalStrength(char* const genBuf, 00189 SnSignalStrengthFrame& sigstr, 00190 const uint32_t timeout_clock); 00191 00192 ECommWinResult GetConfig(SnConfigFrame& conf, 00193 const uint32_t timeOut, 00194 char* const confBuf, 00195 const uint32_t bsize); 00196 00197 ECommWinResult SendStatus(const SnConfigFrame& conf, 00198 const SnPowerFrame& pow, // com win power 00199 const SnEventFrame& stEvent, 00200 const uint16_t seq, 00201 // const float thmrate, 00202 // const float evtrate, 00203 const uint32_t numThmTrigs, 00204 const uint32_t numSavedEvts, 00205 const float seqlive, 00206 const uint32_t powerOnTime, 00207 const SnTempFrame& temper, // com win temp 00208 char* const genBuf, 00209 const uint32_t timeout_clock); 00210 00211 virtual ECommWinResult SendStatusData(const SnConfigFrame& conf, 00212 const SnConfigFrame& stConf, 00213 const SnClockSetFrame& stTrgStartClk, 00214 const SnClockSetFrame& stTrgStopClk, 00215 const SnPowerFrame& stPower, 00216 const SnEventFrame& stEvent, 00217 const SnTempFrame& stTemperature, 00218 const SnHeartbeatFrame& stHeartbeat, 00219 const bool stNewPower, 00220 const bool stNewEvent, 00221 const bool stNewHeartbeat, 00222 const bool stNewTemperature, 00223 char* const genBuf, 00224 const uint32_t timeout_clock); 00225 00226 SnCommWin::ECommWinResult SendString(const char* str, 00227 const uint32_t timeout); 00228 00229 int32_t SendFilename(const char* fn, 00230 char* const genBuf, 00231 int32_t& bytesToBeSent, 00232 const uint32_t timeout_clock); 00233 00234 ECommWinResult SendDataFromFile(FILE* inf, const char* infn, 00235 const SnConfigFrame& curConf, 00236 SnEventFrame& evt, 00237 SnPowerFrame& pow, 00238 char* const genBuf, 00239 const uint32_t bsize, 00240 const uint32_t nevts, 00241 const uint32_t timeout_clock, 00242 uint8_t* hndcode=0, 00243 uint32_t* hndlen=0); 00244 00245 ECommWinResult SendData(SnConfigFrame& conf, 00246 SnEventFrame& evt, 00247 SnPowerFrame& pow, 00248 char* const genBuf, 00249 const uint32_t bsize, 00250 const uint32_t timeout); 00251 00252 // assume little endian 00253 static 00254 uint16_t GetMinSeqFrom(const uint32_t hndshk) { 00255 // left-most 2 bytes 00256 return (hndshk>>16u); 00257 } 00258 static 00259 uint16_t GetMaxSeqFrom(const uint32_t hndshk) { 00260 // right-most 2 bytes 00261 return (hndshk&0xFFFF); 00262 } 00263 00264 }; 00265 00266 #endif // SN_SnCommWin
Generated on Fri Jul 15 2022 00:05:33 by
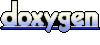