
Arianna autonomous DAQ firmware
Dependencies: mbed SDFileSystemFilinfo AriSnProtocol NetServicesMin AriSnComm MODSERIAL PowerControlClkPatch DS1820OW
SnCommWinTwitter.cpp
00001 #include "SnCommWinTwitter.h" 00002 00003 #include "SnSDUtils.h" 00004 #include "SnConfigFrame.h" 00005 #include "SnStatusFrame.h" 00006 #include "SnCommAfarNetIf.h" 00007 00008 //#define DEBUG 00009 00010 #define MAX_TWEET_LEN 130 00011 #define TWIT_PORT 80 00012 const char* SnCommWinTwitter::kTwitIP = "66.180.175.246"; // api.supertweet.net 00013 const char* SnCommWinTwitter::kTwitUrl = "/1.1/statuses/update.json"; 00014 // these are for ariannatwittest (the test account) 00015 //const char* SnCommWinTwitter::kCred = "ariannatwittest:st11amtice"; 00016 const char* SnCommWinTwitter::kB64cred = "YXJpYW5uYXR3aXR0ZXN0OnN0MTFhbXRpY2U="; // base64 encoded credential 00017 // these are for arianna.icicle (the "real" account) 00018 //const char* SnCommWinTwitter::kCred = "arianna.icicle:st11ibAmicetw"; 00019 //const char* SnCommWinTwitter::kB64cred = "YXJpYW5uYWljaWNsZTpzdDExaWJBbWljZXR3"; // base64 encoded credential 00020 00021 SnCommWinTwitter::SnCommWinTwitter(const SnConfigFrame& conf) : 00022 SnCommWinAfar(new SnCommAfarNetIf(kTwitIP, TWIT_PORT, 00023 conf.GetMbedIP(), 00024 conf.GetMbedMask(), 00025 conf.GetMbedGate())) { 00026 // prevent this second NetIf from re-initializing the ethernet, 00027 // which would cause a crash 00028 static_cast<SnCommAfarNetIf*>(fComm)->SetEthSetup(true); 00029 // make the random number sequence unique for each station 00030 srand(conf.GetMacAddress() & 0xFFFFFFFF); 00031 } 00032 00033 SnCommWin::ECommWinResult SnCommWinTwitter::SendTweet(const char* str, 00034 const uint32_t timeout) { 00035 SnCommWin::ECommWinResult res = SnCommWin::kUndefFail; 00036 // first send headers 00037 const uint32_t slen = strlen(str); 00038 const uint32_t len = (slen>MAX_TWEET_LEN) ? MAX_TWEET_LEN : slen; 00039 char lenstr[16]; 00040 sprintf(lenstr, "%u", len); 00041 std::string post( 00042 "POST /1.1/statuses/update.json HTTP/1.0\r\n"\ 00043 "HOST: api.supertweet.net\r\n"\ 00044 "Authorization: Basic "); 00045 post += kB64cred; 00046 post += "\r\n"\ 00047 "Content-length: "; 00048 post += lenstr; 00049 post += "\r\n"\ 00050 "Content-Type: application/x-www-form-urlencoded\r\n"\ 00051 "\r\n"; 00052 #ifdef DEBUG 00053 printf("sending tweet:\r\n"); 00054 printf("%s",post.c_str()); 00055 #endif 00056 const uint32_t ps = post.size(); 00057 int32_t mlen = fComm->SendAll(post.c_str(), ps, timeout); 00058 mlen += fComm->FinishSending(timeout); 00059 if (ps==mlen) { 00060 // send content 00061 #ifdef DEBUG 00062 printf("%s\r\n",str); 00063 #endif 00064 mlen = fComm->SendAll(str, len, timeout); 00065 mlen += fComm->SendAll("\r\n\r\n\r\n", 6u, timeout); 00066 mlen += fComm->FinishSending(timeout); 00067 if ((6u+len)==mlen) { 00068 res = SnCommWin::kOkMsgSent; 00069 } 00070 } 00071 return res; 00072 } 00073 00074 SnCommWin::ECommWinResult SnCommWinTwitter::Tweet( 00075 const SnConfigFrame& conf, 00076 const float thmrate, 00077 const float evtrate, 00078 char* genBuf, 00079 const uint32_t timeout) { 00080 #ifdef DEBUG 00081 printf("calling tweet with thmr=%g, evtr=%g, to=%u\r\n", 00082 thmrate, evtrate, timeout); 00083 #endif 00084 GetTweet(genBuf, conf, thmrate, evtrate); 00085 return SendTweet(genBuf, timeout); 00086 } 00087 00088 void SnCommWinTwitter::GetTweet(char* genBuf, 00089 const SnConfigFrame& conf, 00090 const float thmrate, 00091 const float evtrate) { 00092 const bool rok = (thmrate>0) && (evtrate>0); 00093 const int r = rok ? (rand() % 4) : (rand() % 3); 00094 char* b = genBuf; 00095 b += sprintf(b, "status="); 00096 if (r==0) { 00097 GetTimeTweet(b, conf); 00098 } else if (r==1) { 00099 GetRunTweet(b, conf); 00100 } else if (r==2) { 00101 GetBytesTweet(b, conf); 00102 } else if (r==3) { 00103 GetRatesTweet(b, conf, thmrate, evtrate); 00104 } 00105 } 00106 00107 void SnCommWinTwitter::GetTimeTweet(char* genBuf, 00108 const SnConfigFrame& conf) { 00109 time_t s = time(0); 00110 char* b = AppendMacId(genBuf, conf); 00111 b += sprintf(b, ": It's "); 00112 b += strftime(b, 32, "%H:%M:%S on %Y-%m-%d", localtime(&s)); 00113 b += sprintf(b, " in the ice."); 00114 } 00115 00116 void SnCommWinTwitter::GetRunTweet(char* genBuf, 00117 const SnConfigFrame& conf) { 00118 char* b = GetGreeting(genBuf, conf); 00119 b += sprintf(b, " I'm making run %u, sequence %hu.", 00120 conf.GetRun(), SnSDUtils::GetCurSeqNum()); 00121 } 00122 00123 void SnCommWinTwitter::GetRatesTweet(char* genBuf, 00124 const SnConfigFrame& conf, 00125 const float thmrate, 00126 const float evtrate) { 00127 char* b = GetGreeting(genBuf, conf); 00128 b += sprintf(b, " I'm getting %2.2f triggers and %2.2f " 00129 "events per second!", thmrate, evtrate); 00130 } 00131 00132 void SnCommWinTwitter::GetBytesTweet(char* genBuf, 00133 const SnConfigFrame& conf) { 00134 char* b = GetGreeting(genBuf, conf); 00135 b += sprintf(b, " I've got %2.2f MB of disk space left.", 00136 SnStatusFrame::GetFreeMB()); 00137 } 00138 00139 char* SnCommWinTwitter::GetGreeting(char* genBuf, 00140 const SnConfigFrame& conf) { 00141 const int r = rand() % 4; 00142 char* b = genBuf; 00143 if (r==0) { 00144 b = AppendMacId(b, conf); 00145 b += sprintf(b, " here."); 00146 } else if (r==1) { 00147 b += sprintf(b, "It's "); 00148 b = AppendMacId(b, conf); 00149 b += sprintf(b, "."); 00150 } else if (r==2) { 00151 b = AppendMacId(b, conf); 00152 b += sprintf(b, " reporting in."); 00153 } else { 00154 b = AppendMacId(b, conf); 00155 b += sprintf(b, " again."); 00156 } 00157 00158 return b; 00159 } 00160 00161 char* SnCommWinTwitter::AppendMacId(char* genBuf, 00162 const SnConfigFrame& conf) { 00163 char* b = genBuf; 00164 b += sprintf(b, "%04llX", ((conf.GetMacAddress() >> 16) & 0xFFFF)); 00165 return b; 00166 } 00167 /* 00168 bool SnCommWinTwitter::Connect(const uint32_t timeout) { 00169 00170 #ifdef DEBUG 00171 printf("SnCommAfarNetIf::Connect : bind\r\n"); 00172 #endif 00173 00174 ip_addr_t rserv_n; 00175 inet_aton(fRserv.c_str(), &rserv_n); 00176 Host server( IpAddr(&rserv_n), fRport ); 00177 //Host server( IpAddr(128,195,204,151), 6655 ); 00178 00179 SockConnect(server, timeout); 00180 00181 // catch events 00182 fSock->setOnEvent(this, &SnCommWinTwitter::onTCPSocketEvent); 00183 00184 #ifdef DEBUG 00185 printf("SnCommAfarNetIf::Connect : tcp connect\r\n"); 00186 #endif 00187 //fEthConnected = false; 00188 while ( (fEthConnected==false) && (IsTimedOut(timeout)==false) ) { 00189 Net::poll(); 00190 if (fConnAborted) { 00191 fConnAborted = false; 00192 //NewSocket(); 00193 SockConnect(server, timeout); 00194 } 00195 } 00196 00197 fEthWriteable = fEthConnected; 00198 return fEthConnected; 00199 00200 } 00201 00202 void SnCommWinTwitter::onTCPSocketEvent(TCPSocketEvent e) { 00203 SnCommAfarNetIf::onTCPSocketEvent(e); 00204 } 00205 */
Generated on Fri Jul 15 2022 00:05:33 by
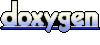