
Arianna autonomous DAQ firmware
Dependencies: mbed SDFileSystemFilinfo AriSnProtocol NetServicesMin AriSnComm MODSERIAL PowerControlClkPatch DS1820OW
SnCommAfarTCP.cpp
00001 #if 0 00002 00003 #include "SnCommAfarTCP.h" 00004 00005 #include "EthernetInterface.h" 00006 #include "TCPSocketConnection.h" 00007 00008 #include "Watchdog.h" 00009 00010 //#define DEBUG 00011 00012 #define CALL_MEMBER_FN(object,ptrToMember) ((object).*(ptrToMember)) 00013 00014 SnCommAfarTCP::SnCommAfarTCP(const SnConfigFrame& conf) : 00015 fUseB64(false), fRserv(conf.GetRemoteServer()), 00016 fRport(conf.GetRemotePort()), 00017 fMyIp(conf.GetMbedIP()), fMyMask(conf.GetMbedMask()), 00018 fMyGate(conf.GetMbedGate()), 00019 fEth(new EthernetInterface), fSock(new TCPSocketConnection) { 00020 00021 #ifdef DEBUG 00022 printf("init. ip=%s, mas=%s, gate=%s\r\n", 00023 fMyIp.c_str(),fMyMask.c_str(), fMyGate.c_str()); 00024 #endif 00025 fEth->init(fMyIp.c_str(),fMyMask.c_str(), fMyGate.c_str()); 00026 } 00027 00028 SnCommAfarTCP::~SnCommAfarTCP() { 00029 delete fEth; 00030 delete fSock; 00031 } 00032 00033 void SnCommAfarTCP::Set(const char* remote, const uint16_t rport, 00034 const char* myip, const char* mask, 00035 const char* gate, const bool useb64) { 00036 fUseB64 = useb64; 00037 fRserv = remote; 00038 fRport = rport; 00039 fMyIp = myip; 00040 fMyMask = mask; 00041 fMyGate = gate; 00042 #ifdef DEBUG 00043 printf("closing socket\r\n"); 00044 #endif 00045 fSock->close(); 00046 //delete fSock; 00047 //fSock = new TCPSocketConnection; 00048 #ifdef DEBUG 00049 printf("disconnect eth\r\n"); 00050 #endif 00051 fEth->disconnect(); 00052 //delete fEth; 00053 //fEth = new EthernetInterface; 00054 #ifdef DEBUG 00055 printf("init %s, %s, %s\r\n",fMyIp.c_str(), fMyMask.c_str(), fMyGate.c_str()); 00056 #endif 00057 fEth->init(fMyIp.c_str(), fMyMask.c_str(), fMyGate.c_str()); 00058 00059 #ifdef DEBUG 00060 printf("Set done\r\n"); 00061 #endif 00062 } 00063 00064 int SnCommAfarTCP::DoIO(char* const data, 00065 const uint32_t length, 00066 const uint32_t timeout_clock, 00067 TCPSendRecv fcn) { 00068 // TODO: if B64, must return number of bytes of raw (non encoded) message 00069 #ifdef DEBUG 00070 printf("SnCommAfarTCP::DoIO data:\r\n"); 00071 dispStrBytes(data, length); 00072 printf("\r\n"); 00073 #endif 00074 int res=0; 00075 uint32_t b=0; 00076 while ( (length>b) ) { 00077 if (IsTimedOut(timeout_clock)) { 00078 #ifdef DEBUG 00079 printf("SnCommAfarTCP::DoIO timing out\r\n"); 00080 #endif 00081 break; 00082 } 00083 res = CALL_MEMBER_FN(*fSock, fcn)(data+b, length-b); 00084 /* 00085 switch (res) { 00086 case -1: 00087 // TODO: how to check the error? 00088 continue; 00089 case 0: 00090 return b; 00091 default: 00092 b += res; 00093 }; 00094 */ 00095 if (res>0) { 00096 b += res; 00097 } 00098 //wait_ms(100); 00099 //Watchdog::kick(); // don't reset; wait until timeout 00100 } 00101 printf("return b=%d\r\n",b); 00102 return b; // timeout 00103 } 00104 00105 int32_t SnCommAfarTCP::ReceiveAll(char* const buf, const uint32_t mlen, 00106 const uint32_t timeout_clock) { 00107 // TODO: if B64, must return number of bytes of raw (non encoded) message 00108 //return DoIO(buf, mlen, timeout_clock, &TCPSocketConnection::receive_all); 00109 // use regular receive; DoIO will do a receive_all but use our timeout 00110 return DoIO(buf, mlen, timeout_clock, &TCPSocketConnection::receive); 00111 } 00112 00113 int32_t SnCommAfarTCP::SendAll(char* const data, const uint32_t length, 00114 const uint32_t timeout_clock) { 00115 // TODO: if B64, must return number of bytes of raw (non encoded) message 00116 //return DoIO(data, length, timeout_clock, &TCPSocketConnection::send_all); 00117 // use regular send; DoIO will do a send_all but use our timeout 00118 return DoIO(data, length, timeout_clock, &TCPSocketConnection::send); 00119 } 00120 00121 00122 bool SnCommAfarTCP::Connect(const uint32_t timeout) { 00123 #ifdef DEBUG 00124 printf("SnCommAfarTCP::Connect\r\n"); 00125 #endif 00126 bool isConn = false; 00127 00128 while ( (isConn==false) && (IsTimedOut(timeout)==false) ) { 00129 wait_ms(250); 00130 isConn = (fEth->connect()==0); 00131 } 00132 #ifdef DEBUG 00133 printf("eth isConn=%d\r\n",(int)isConn); 00134 #endif 00135 00136 isConn=false; 00137 while ( (isConn==false) && (IsTimedOut(timeout)==false) ) { 00138 wait_ms(250); 00139 isConn = (fSock->connect(fRserv.c_str(), fRport)==0); 00140 } 00141 #ifdef DEBUG 00142 printf("sock isConn=%d\r\n",(int)isConn); 00143 #endif 00144 return isConn; 00145 } 00146 00147 SnCommWin::ECommWinResult SnCommAfarTCP::OpenWindow(const uint32_t timeout, 00148 const bool sendStatus, 00149 const SnConfigFrame& conf, 00150 const SnEventFrame& evt, 00151 const SnPowerFrame& pow, 00152 const uint16_t seq, 00153 const float thmrate, 00154 const float evtrate, 00155 char* const genBuf) { 00156 #ifdef DEBUG 00157 printf("SnCommAfarTCP::OpenWindow\r\n"); 00158 #endif 00159 const bool canCon = Connect(timeout); 00160 00161 SnCommWin::ECommWinResult ret = canCon ? SnCommWin::kConnected 00162 : SnCommWin::kCanNotConnect; 00163 00164 if (canCon && sendStatus) { 00165 #ifdef DEBUG 00166 printf("calling SendStatus\r\n"); 00167 #endif 00168 ret = SendStatus(conf, evt, pow, seq, thmrate, evtrate, genBuf, timeout); 00169 } 00170 00171 return ret; 00172 } 00173 00174 bool SnCommAfarTCP::CloseConn(const uint32_t) { 00175 return (fSock->close())==0; 00176 } 00177 00178 #endif
Generated on Fri Jul 15 2022 00:05:33 by
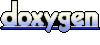