
Code to receive input from foot and hand module and sends data to computer as a key press
Dependencies: USBDevice XBee mbed
Wireless.h
00001 #ifndef WProtocol_h 00002 #define WProtocol_h 00003 00004 #include "XBee/XBee.h" 00005 #include <mbed.h> 00006 00007 #define HOST_ADDRESS 0x0003 00008 00009 enum Direction {DIR_UP, DIR_DOWN, DIR_LEFT, DIR_RIGHT, DIR_NONE, A_BUTTON, B_BUTTON}; 00010 enum WMessageType {FOOT_STEP, HAND_GESTURE}; 00011 00012 typedef struct WMessage { 00013 WMessageType type; 00014 Direction direction; 00015 } WMessage_t; 00016 00017 class WirelessModule { 00018 public: 00019 WirelessModule(PinName tx, PinName rx, WMessageType type) : 00020 uart(tx, rx), xbee(uart), 00021 tx16req(HOST_ADDRESS, (uint8_t *) &message, sizeof(message)) 00022 { 00023 uart.baud(9600); 00024 message.type = type; 00025 } 00026 00027 int sendDirection(Direction dir) { 00028 message.direction = dir; 00029 xbee.send(tx16req); 00030 return 0; 00031 } 00032 private: 00033 RawSerial uart; 00034 XBee xbee; 00035 WMessage_t message; 00036 Tx16Request tx16req; 00037 }; 00038 00039 class WirelessHost { 00040 public: 00041 WirelessHost(PinName tx, PinName rx) : uart(tx, rx), xbee(uart) {} 00042 00043 int waitForMessage(WMessage_t *store) { 00044 xbee.readPacket(5000); 00045 00046 if (xbee.getResponse().isError()) { 00047 // API error 00048 return -99; 00049 } 00050 00051 if (xbee.getResponse().isAvailable()) { 00052 if (xbee.getResponse().getApiId() != RX_16_RESPONSE) { 00053 // Unexpected API message 00054 return -5; 00055 } 00056 00057 // Retrieve response 00058 xbee.getResponse().getRx16Response(rx16resp); 00059 00060 // Validate packet length 00061 if (rx16resp.getDataLength() == sizeof(WMessage_t)) { 00062 // Store the payload 00063 memcpy(store, rx16resp.getData(), sizeof(WMessage_t)); 00064 return 0; 00065 } else { 00066 // Unexpected payload format 00067 return -10; 00068 } 00069 } else { 00070 // No Response 00071 return -1; 00072 } 00073 } 00074 00075 private: 00076 RawSerial uart; 00077 XBee xbee; 00078 Rx16Response rx16resp; 00079 }; 00080 00081 #endif
Generated on Sat Jul 23 2022 09:49:15 by
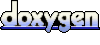