
Final code for our 4180 Drawing Robot!
Dependencies: 4DGL-uLCD-SE gCodeParser mbed
SerialIO.cpp
00001 // SerialIO.cpp : Defines the entry point for the console application. 00002 00003 #include "stdafx.h" 00004 #include <windows.h> 00005 #include <stdio.h> 00006 #include "listCOM.h" 00007 #include <iostream> 00008 #include <fstream> 00009 #include <string> 00010 #include <ctype.h> 00011 00012 using namespace std; 00013 00014 #define FEED 10 00015 #define END_OF_TRANSMISSION 23 00016 00017 BOOL ModifyCommSettings(HANDLE hComPort); 00018 HANDLE hSerial; 00019 00020 int openConn() 00021 { 00022 DWORD cBytes_out, cBytes_in; 00023 DWORD dwMask; 00024 00025 char cBuffer_in[2]; 00026 cBuffer_in[1] = 0; 00027 // Display message on console 00028 printf("\nOpening Serial Port \n\r"); 00029 00030 // Open Serial Port COMx: for Read and Write 00031 hSerial = CreateFile((LPCWSTR)my_port, GENERIC_READ | GENERIC_WRITE, 0, NULL, 00032 OPEN_EXISTING, 0, NULL); 00033 // Check for file open errors 00034 if (hSerial == INVALID_HANDLE_VALUE){ 00035 printf("file open errors\n"); 00036 Sleep(4000); 00037 return 0; 00038 } 00039 // Modify Com Port settings (i.e. Baud Rate, #bits, parity etc) 00040 if (!ModifyCommSettings(hSerial)){ 00041 printf("com port settings errors\n"); 00042 Sleep(4000); 00043 return 0; 00044 } 00045 printf("\n Opened Serial Connection with mbed \n\r Ctrl C to exit\n\r"); 00046 00047 // Set Communication event mask for WaitCommEvent for rxchar (receive character) in buffer 00048 SetCommMask(hSerial, EV_RXCHAR | EV_ERR); 00049 00050 // Setting up the reading of the text file 00051 ifstream iFile("C:\\ECE4180\\hellombed.gcode"); 00052 if (iFile) printf("\n Gcode file opened successfully\r\n"); 00053 00054 string line; 00055 // creating a pointer to char to store the C version of the line string 00056 char* c_line; 00057 00058 cBuffer_in[0] = 0; 00059 // reading the gcode file line by line 00060 while (getline(iFile, line)){ 00061 00062 // finding the length of the message 00063 cBytes_out = line.length() + 1; 00064 // allocating space for C representation of string to be sent over serial 00065 c_line = new char[cBytes_out]; 00066 00067 //coyping data to C string c_line 00068 strcpy_s(c_line, cBytes_out, line.c_str()); 00069 00070 cout << c_line << "\r\n"; 00071 00072 // checking that the line contains a valid command. 00073 // TODO: only checking for starting command being a G#*, might have invalid args that are not checked 00074 if (c_line[0] == 'G' && isdigit(c_line[1])){ 00075 // sending the line over the serial link 00076 if (!WriteFile(hSerial, c_line, cBytes_out, &cBytes_out, NULL)){ 00077 printf("\rFile write errors\n"); 00078 Sleep(4000); 00079 return 0; 00080 } 00081 00082 // deleting the dynamic buffer after sending 00083 delete[] c_line; 00084 } 00085 // if the line does not have a command continue to the next line 00086 else{ 00087 // deleting the dynamic buffer after sending 00088 delete[] c_line; 00089 continue; 00090 } 00091 00092 // Wait for character to be sent back to UARTs input buffer - events are more efficient than looping 00093 WaitCommEvent(hSerial, &dwMask, 0); 00094 00095 cBuffer_in[0] = 0; 00096 // Loop until receiving the FEED signal 00097 while (cBuffer_in[0] != FEED){ 00098 // Read constantly waiting for FEED character 00099 ReadFile(hSerial, cBuffer_in, 1, &cBytes_in, NULL); 00100 00101 /* 00102 // Read any serial data and display 00103 if (ReadFile(hSerial, cBuffer_in, 1, &cBytes_in, NULL)){ 00104 00105 cout << cBuffer_in << "\r\n"; 00106 cout << cBytes_in << " bytes received \r\n"; 00107 } 00108 */ 00109 } //while cBuffer_in[0] != FEED 00110 } // while getline 00111 00112 // after the last line has been sent, sent the end of transmission message 00113 c_line[0] = END_OF_TRANSMISSION; 00114 c_line[1] = 0; // NULL terminate the string 00115 cBytes_out = 2; 00116 00117 // attempt until message is sent 00118 while (!WriteFile(hSerial, c_line, cBytes_out, &cBytes_out, NULL)); 00119 00120 string input; 00121 cout << "Press any key followed by ENTER to exit...\n>"; 00122 cin >> input; 00123 00124 // Close Files 00125 iFile.close(); 00126 CloseHandle(hSerial); 00127 return 0; 00128 } 00129 00130 BOOL ModifyCommSettings(HANDLE hComPort) 00131 { 00132 COMMTIMEOUTS ctos; 00133 DCB PortDCB; 00134 // Initialize the DCBlength member. 00135 PortDCB.DCBlength = sizeof (DCB); 00136 // Get the default serial port settings DCB information. 00137 GetCommState(hSerial, &PortDCB); 00138 // Change the common DCB structure settings to modify serial port settings. 00139 PortDCB.BaudRate = 921600; // Current baud 00140 PortDCB.fBinary = TRUE; // Binary mode; no EOF check 00141 PortDCB.fParity = TRUE; // Enable parity checking 00142 PortDCB.fOutxCtsFlow = FALSE; // No CTS output flow control 00143 PortDCB.fOutxDsrFlow = FALSE; // No DSR output flow control 00144 PortDCB.fDtrControl = DTR_CONTROL_ENABLE; // DTR flow control type 00145 PortDCB.fDsrSensitivity = FALSE; // DSR sensitivity 00146 PortDCB.fTXContinueOnXoff = TRUE; // XOFF continues Tx 00147 PortDCB.fOutX = FALSE; // No XON/XOFF out flow control 00148 PortDCB.fInX = FALSE; // No XON/XOFF in flow control 00149 PortDCB.fErrorChar = FALSE; // Disable error replacement 00150 PortDCB.fNull = FALSE; // Disable null stripping 00151 PortDCB.fRtsControl = RTS_CONTROL_ENABLE; // RTS flow control 00152 PortDCB.fAbortOnError = FALSE; // Do not abort reads/writes on error 00153 PortDCB.ByteSize = 8; // Number of bits/byte, 4-8 00154 PortDCB.Parity = NOPARITY; // 0-4=no,odd,even,mark,space 00155 PortDCB.StopBits = ONESTOPBIT; // 0,1,2 = 1, 1.5, 2 00156 // Configure the port settings according to the new specifications 00157 // of the DCB structure. 00158 if (!SetCommState(hSerial, &PortDCB)){ 00159 printf("Unable to configure the serial port"); 00160 Sleep(4000); 00161 return false; 00162 } 00163 // Set read time outs 00164 ctos.ReadIntervalTimeout = MAXDWORD; 00165 ctos.ReadTotalTimeoutMultiplier = MAXDWORD; 00166 ctos.ReadTotalTimeoutConstant = 1; 00167 ctos.WriteTotalTimeoutMultiplier = 0; 00168 ctos.WriteTotalTimeoutConstant = 0; 00169 if (!SetCommTimeouts(hSerial, &ctos)){ 00170 printf("Unable to configure the serial port"); 00171 Sleep(4000); 00172 return false; 00173 } 00174 return true; 00175 } 00176
Generated on Thu Jul 21 2022 12:50:48 by
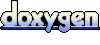