
Driver for controlling Renishaw RenBuggy
Dependencies: SevenSegmentDisplay DCMotorDrive mbed MotorController
main.cpp
00001 /******************************************************************************* 00002 * This program demonstrates how to drive the seven segment display * 00003 * * 00004 * Jon Fuge * 00005 * V1.0 24/12/2013 First issue of code * 00006 *******************************************************************************/ 00007 00008 #include "mbed.h" 00009 #include "SevenSegmentDisplay.h" 00010 #include "MotorController.h" 00011 00012 uint16_t u16LeftSpeed = 0, u16RightSpeed = 0; 00013 00014 // Options to instantiate SevenSegmentDisplay are... 00015 // FADE: causes the number changes to fade in smoothly 00016 // INSTANT: causes the an instant number change 00017 // + FLASH: causes the display to flash 00018 SevenSegmentDisplay segmentled( FADE ); 00019 00020 MotorController RenBuggy(p5, p7, p9, p6, p8, p10); 00021 DigitalIn LFast(p9), LSlow(p12), RFast(p15), RSlow(p18); 00022 00023 void attimeout(); //declare prototype for timeout handler. 00024 00025 Ticker timeout; //Create an instance of class Ticker called timeout. 00026 00027 int main() 00028 { 00029 LFast.mode(PullUp); 00030 LSlow.mode(PullUp); 00031 RFast.mode(PullUp); 00032 RSlow.mode(PullUp); 00033 00034 timeout.attach(&attimeout, 3); 00035 // LeftMotor.SetMotorPwmAndRevolutions(18000,32); 00036 00037 RenBuggy.Forwards(100); 00038 00039 for(;;) { 00040 } // Loop forever (the program uses interrupts) 00041 } 00042 00043 void attimeout() 00044 { 00045 static int Flip = 0; 00046 00047 if (Flip++ == 0) 00048 segmentled.DisplayInt(RenBuggy.ReadLeftOdometer()); 00049 else 00050 { 00051 segmentled.DisplayInt(RenBuggy.ReadRightOdometer()); 00052 Flip = 0; 00053 } 00054 }
Generated on Thu Jul 14 2022 03:16:02 by
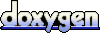