A motor control class for driving two DC motors as part of RenBuggy
Embed:
(wiki syntax)
Show/hide line numbers
MotorController.h
00001 /******************************************************************************* 00002 * RenBED DC Motor Drive for RenBuggy * 00003 * Copyright (c) 2014 Jon Fuge * 00004 * * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy * 00006 * of this software and associated documentation files (the "Software"), to deal* 00007 * in the Software without restriction, including without limitation the rights * 00008 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell * 00009 * copies of the Software, and to permit persons to whom the Software is * 00010 * furnished to do so, subject to the following conditions: * 00011 * * 00012 * The above copyright notice and this permission notice shall be included in * 00013 * all copies or substantial portions of the Software. * 00014 * * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR * 00016 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, * 00017 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE * 00018 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER * 00019 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,* 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN * 00021 * THE SOFTWARE. * 00022 * * 00023 * DCMotorDrive.h * 00024 * * 00025 * V1.0 06/01/2014 First issue of code Jon Fuge * 00026 *******************************************************************************/ 00027 00028 #ifndef _DCMOTORCONTROLLER_H 00029 #define _DCMOTORCONTROLLER_H 00030 00031 #include "mbed.h" 00032 #include "DCMotorDrive.h" 00033 00034 #define WHEEL_CIRCUMFERENCE 114.4 00035 #define COUNTS_PER_ROTATION 64 00036 #define PWM_SPEED_ADJUST 20 00037 #define ROTATION_LIMIT 20 00038 00039 class MotorController 00040 { 00041 public: 00042 MotorController(PinName LMotorOut, PinName LBrakeOut, PinName LSensorIn, PinName LMotorOut, PinName RBrakeOut, PinName LSensorIn); 00043 void Forwards(int ForwardCount); 00044 void ResetOdometer(void); 00045 int ReadOdometer(void); 00046 int ReadLeftOdometer(void); 00047 int ReadRightOdometer(void); 00048 private: 00049 DCMotorDrive _LeftMotor; 00050 DCMotorDrive _RightMotor; 00051 int LeftMotorSpeed; 00052 int RightMotorSpeed; 00053 int LeftToRightRatio; 00054 int DistanceToTravel; 00055 Ticker trackomatic; 00056 00057 void MatchSpeeds(void); 00058 void UpdatePwms(void); 00059 }; 00060 00061 #endif // _DCMOTORCONTROLLER_H
Generated on Sat Jul 16 2022 18:57:57 by
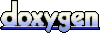