Dual DC Motor Drive using PWM for RenBuggy; there are two inputs to feed a hall sensor for distance / speed feedback.
DCMotorDrive.cpp
00001 /******************************************************************************* 00002 * RenBED DC Motor Drive for RenBuggy * 00003 * Copyright (c) 2014 Jon Fuge * 00004 * * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy * 00006 * of this software and associated documentation files (the "Software"), to deal* 00007 * in the Software without restriction, including without limitation the rights * 00008 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell * 00009 * copies of the Software, and to permit persons to whom the Software is * 00010 * furnished to do so, subject to the following conditions: * 00011 * * 00012 * The above copyright notice and this permission notice shall be included in * 00013 * all copies or substantial portions of the Software. * 00014 * * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR * 00016 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, * 00017 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE * 00018 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER * 00019 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,* 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN * 00021 * THE SOFTWARE. * 00022 * * 00023 * DCMotorDrive.h * 00024 * * 00025 * V1.0 06/01/2014 First issue of code Jon Fuge * 00026 *******************************************************************************/ 00027 00028 #ifndef _DCMOTORDRIVE_C 00029 #define _DCMOTORDRIVE_C 00030 00031 #include "mbed.h" 00032 #include "DCMotorDrive.h" 00033 00034 DCMotorDrive::DCMotorDrive(PinName MotorOut, PinName BrakeOut, PinName SensorIn): 00035 _MotorPin(MotorOut), _BrakePin(BrakeOut), _SensorIn(SensorIn) 00036 { 00037 _MotorPin.period_ms(PWM_PERIOD); 00038 SetMotorPwmAndRevolutions(0,0); 00039 _SensorIn.mode(PullUp); 00040 _SensorIn.fall(this, &DCMotorDrive::Counter); 00041 _BrakePin = 1; 00042 PulseClock.start(); 00043 PulseClock.reset(); 00044 ResetOdometer(); 00045 } 00046 00047 void DCMotorDrive::SetMotorPwm(int PwmValue) 00048 { 00049 if (PwmValue <= 0) 00050 { 00051 _MotorPin.pulsewidth_us(0); 00052 _BrakePin = 1; 00053 } else 00054 { 00055 _BrakePin = 0; 00056 timeout.attach(this, &DCMotorDrive::Stall, MOTOR_STALL_TIME); 00057 _MotorPin.pulsewidth_us(PwmValue); 00058 } 00059 } 00060 00061 void DCMotorDrive::SetMotorPwmAndRevolutions(int PwmValue, int MaxRevolutions) 00062 { 00063 SetMotorPwm(PwmValue); 00064 RevolutionLimit = MaxRevolutions; 00065 } 00066 00067 int DCMotorDrive::GetLastPulseTime(void) 00068 { 00069 return(LastPulseTime); 00070 } 00071 00072 int DCMotorDrive::GetRevolutionsLeft(void) 00073 { 00074 return(RevolutionLimit); 00075 } 00076 void DCMotorDrive::ResetOdometer(void) 00077 { 00078 RotationCounter = 0; 00079 } 00080 00081 int DCMotorDrive::ReadOdometer(void) 00082 { 00083 return(RotationCounter); 00084 } 00085 00086 void DCMotorDrive::Counter(void) 00087 { 00088 LastPulseTime = PulseClock.read_us(); 00089 PulseClock.reset(); 00090 timeout.detach(); 00091 timeout.attach(this, &DCMotorDrive::Stall, MOTOR_STALL_TIME); 00092 RotationCounter++; 00093 if (RevolutionLimit != 0) 00094 if (--RevolutionLimit == 0) 00095 { 00096 SetMotorPwmAndRevolutions(0,0); 00097 _BrakePin = 1; 00098 timeout.detach(); 00099 } 00100 } 00101 00102 void DCMotorDrive::Stall(void) 00103 { 00104 SetMotorPwmAndRevolutions(0,0); 00105 _BrakePin = 1; 00106 timeout.detach(); 00107 } 00108 00109 #endif
Generated on Sun Jul 24 2022 16:27:15 by
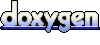