
This program simply connects to a HTS221 I2C device to read Temperature
Embed:
(wiki syntax)
Show/hide line numbers
hts221_driver.cpp
00001 00002 #include "HTS221.h" 00003 00004 00005 // ------------------------------------------------------------------------------ 00006 //jmf -- define I2C pins and functions to read & write to I2C device 00007 00008 #include <string> 00009 #include "mbed.h" 00010 00011 #include "hardware.h" 00012 //I2C i2c(PTC11, PTC10); //SDA, SCL -- define the I2C pins being used. Defined in a 00013 //common locatioin since sensors also use I2C 00014 00015 // Read a single unsigned char from addressToRead and return it as a unsigned char 00016 unsigned char HTS221::readRegister(unsigned char slaveAddress, unsigned char ToRead) 00017 { 00018 char data = ToRead; 00019 00020 //i2c.write(slaveAddress, &data, 1, 0); 00021 i2c.write(slaveAddress, &data, 1, 1); //by Stefan 00022 i2c.read(slaveAddress, &data, 1, 0); 00023 return data; 00024 } 00025 00026 // Writes a single unsigned char (dataToWrite) into regToWrite 00027 int HTS221::writeRegister(unsigned char slaveAddress, unsigned char regToWrite, unsigned char dataToWrite) 00028 { 00029 const char data[] = {regToWrite, dataToWrite}; 00030 00031 return i2c.write(slaveAddress,data,2,0); 00032 } 00033 00034 00035 //jmf end 00036 // ------------------------------------------------------------------------------ 00037 00038 //static inline int humidityReady(uint8_t data) { 00039 // return (data & 0x02); 00040 //} 00041 //static inline int temperatureReady(uint8_t data) { 00042 // return (data & 0x01); 00043 //} 00044 00045 00046 HTS221::HTS221(void) : _address(HTS221_ADDRESS) 00047 { 00048 _temperature = 0; 00049 _humidity = 0; 00050 } 00051 00052 00053 int HTS221::begin(void) 00054 { 00055 uint8_t data; 00056 00057 data = readRegister(_address, WHO_AM_I); 00058 if (data == WHO_AM_I_RETURN){ 00059 if (activate()){ 00060 storeCalibration(); 00061 return data; 00062 } 00063 } 00064 00065 return 0; 00066 } 00067 00068 int 00069 HTS221::storeCalibration(void) 00070 { 00071 uint8_t data; 00072 uint16_t tmp; 00073 00074 for (int reg=CALIB_START; reg<=CALIB_END; reg++) { 00075 if ((reg!=CALIB_START+8) && (reg!=CALIB_START+9) && (reg!=CALIB_START+4)) { 00076 00077 data = readRegister(HTS221_ADDRESS, reg); 00078 00079 switch (reg) { 00080 case CALIB_START: 00081 _h0_rH = data; 00082 break; 00083 case CALIB_START+1: 00084 _h1_rH = data; 00085 break; 00086 case CALIB_START+2: 00087 _T0_degC = data; 00088 break; 00089 case CALIB_START+3: 00090 _T1_degC = data; 00091 break; 00092 00093 case CALIB_START+5: 00094 tmp = _T0_degC; 00095 _T0_degC = (data&0x3)<<8; 00096 _T0_degC |= tmp; 00097 00098 tmp = _T1_degC; 00099 _T1_degC = ((data&0xC)>>2)<<8; 00100 _T1_degC |= tmp; 00101 break; 00102 case CALIB_START+6: 00103 _H0_T0 = data; 00104 break; 00105 case CALIB_START+7: 00106 _H0_T0 |= data<<8; 00107 break; 00108 case CALIB_START+0xA: 00109 _H1_T0 = data; 00110 break; 00111 case CALIB_START+0xB: 00112 _H1_T0 |= data <<8; 00113 break; 00114 case CALIB_START+0xC: 00115 _T0_OUT = data; 00116 break; 00117 case CALIB_START+0xD: 00118 _T0_OUT |= data << 8; 00119 break; 00120 case CALIB_START+0xE: 00121 _T1_OUT = data; 00122 break; 00123 case CALIB_START+0xF: 00124 _T1_OUT |= data << 8; 00125 break; 00126 00127 00128 case CALIB_START+8: 00129 case CALIB_START+9: 00130 case CALIB_START+4: 00131 //DO NOTHING 00132 break; 00133 00134 // to cover any possible error 00135 default: 00136 return false; 00137 } /* switch */ 00138 } /* if */ 00139 } /* for */ 00140 return true; 00141 } 00142 00143 00144 int 00145 HTS221::activate(void) 00146 { 00147 uint8_t data; 00148 00149 data = readRegister(_address, CTRL_REG1); 00150 data |= POWER_UP; 00151 data |= ODR0_SET; 00152 writeRegister(_address, CTRL_REG1, data); 00153 00154 return true; 00155 } 00156 00157 00158 int HTS221::deactivate(void) 00159 { 00160 uint8_t data; 00161 00162 data = readRegister(_address, CTRL_REG1); 00163 data &= ~POWER_UP; 00164 writeRegister(_address, CTRL_REG1, data); 00165 return true; 00166 } 00167 00168 00169 int 00170 HTS221::bduActivate(void) 00171 { 00172 uint8_t data; 00173 00174 data = readRegister(_address, CTRL_REG1); 00175 data |= BDU_SET; 00176 writeRegister(_address, CTRL_REG1, data); 00177 00178 return true; 00179 } 00180 00181 00182 int 00183 HTS221::bduDeactivate(void) 00184 { 00185 uint8_t data; 00186 00187 data = readRegister(_address, CTRL_REG1); 00188 data &= ~BDU_SET; 00189 writeRegister(_address, CTRL_REG1, data); 00190 return true; 00191 } 00192 00193 00194 int 00195 HTS221::readHumidity(void) 00196 { 00197 uint8_t data = 0; 00198 uint16_t h_out = 0; 00199 double h_temp = 0.0; 00200 double hum = 0.0; 00201 00202 data = readRegister(_address, STATUS_REG); 00203 00204 if (data & HUMIDITY_READY) { 00205 data = readRegister(_address, HUMIDITY_H_REG); 00206 h_out = data << 8; // MSB 00207 data = readRegister(_address, HUMIDITY_L_REG); 00208 h_out |= data; // LSB 00209 00210 // Decode Humidity 00211 hum = ((int16_t)(_h1_rH) - (int16_t)(_h0_rH))/2.0; // remove x2 multiple 00212 00213 // Calculate humidity in decimal of grade centigrades i.e. 15.0 = 150. 00214 h_temp = (((int16_t)h_out - (int16_t)_H0_T0) * hum) / ((int16_t)_H1_T0 - (int16_t)_H0_T0); 00215 hum = ((int16_t)_h0_rH) / 2.0; // remove x2 multiple 00216 _humidity = (int16_t)((hum + h_temp)); // provide signed % measurement unit 00217 } 00218 return _humidity; 00219 } 00220 00221 00222 00223 double 00224 HTS221::readTemperature(void) 00225 { 00226 uint8_t data = 0; 00227 uint16_t t_out = 0; 00228 double t_temp = 0.0; 00229 double deg = 0.0; 00230 00231 data = readRegister(_address, STATUS_REG); 00232 00233 if (data & TEMPERATURE_READY) { 00234 00235 data= readRegister(_address, TEMP_H_REG); 00236 t_out = data << 8; // MSB 00237 data = readRegister(_address, TEMP_L_REG); 00238 t_out |= data; // LSB 00239 00240 // Decode Temperature 00241 deg = ((int16_t)(_T1_degC) - (int16_t)(_T0_degC))/8.0; // remove x8 multiple 00242 00243 // Calculate Temperature in decimal of grade centigrades i.e. 15.0 = 150. 00244 t_temp = (((int16_t)t_out - (int16_t)_T0_OUT) * deg) / ((int16_t)_T1_OUT - (int16_t)_T0_OUT); 00245 deg = ((int16_t)_T0_degC) / 8.0; // remove x8 multiple 00246 _temperature = deg + t_temp; // provide signed celsius measurement unit 00247 } 00248 00249 return _temperature; 00250 }
Generated on Mon Jul 18 2022 21:05:05 by
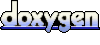