
Game controller_v2: 091815. changed to read acceleration in x axis
Dependencies: DebounceIn MMA8451Q USBDevice mbed
Fork of HW3_Game_Controller by
gamecontroller2.cpp
00001 #include "mbed.h" 00002 #include "DebounceIn.h" 00003 #include "USBKeyboard.h" 00004 #include "MMA8451Q.h" 00005 00006 PinName const SDA = PTE25; 00007 PinName const SCL = PTE24; 00008 #define MMA8451_I2C_ADDRESS (0X1d<<1) 00009 Serial pc(USBTX, USBRX); 00010 00011 USBKeyboard keyboard; 00012 00013 DigitalOut ledr(LED_RED); 00014 DigitalOut ledg(LED_GREEN); 00015 DigitalOut ledb(LED_BLUE); 00016 00017 DebounceIn pb1(D13); 00018 DebounceIn pb2(D3); 00019 DebounceIn pb3(D4); 00020 DebounceIn pb4(D5); 00021 DebounceIn pb5(D6); 00022 DebounceIn pb6(D7); 00023 DebounceIn pb7(D8); 00024 DebounceIn pbs(D9); 00025 DebounceIn pbm(D10); 00026 00027 int main() 00028 { 00029 int m = 1; 00030 ledr = 1; 00031 ledg = 1; 00032 ledb = 1; 00033 // Use internal pullup for pushbutton 00034 pb1.mode(PullUp); 00035 pb2.mode(PullUp); 00036 pb3.mode(PullUp); 00037 pb4.mode(PullUp); 00038 pb5.mode(PullUp); 00039 pb6.mode(PullUp); 00040 pb7.mode(PullUp); 00041 pbs.mode(PullUp); 00042 pbm.mode(PullUp); 00043 00044 // Delay for initial pullup to take effect 00045 wait(.001); 00046 00047 MMA8451Q acc(SDA, SCL, MMA8451_I2C_ADDRESS); 00048 00049 float x; 00050 float y; 00051 float z; 00052 00053 while(true) 00054 { 00055 x = acc.getAccX(); 00056 y = acc.getAccY(); 00057 z = acc.getAccZ(); 00058 00059 00060 //keyboard.printf("X = %1.2f ", x); 00061 //keyboard.printf("Y = %1.2f ", y); 00062 //keyboard.printf("Z = ", z); 00063 00064 if (x < -0.35) {keyboard.keyCode(LEFT_ARROW); 00065 wait(.2); 00066 00067 } 00068 if (x > 0.35) {keyboard.keyCode(RIGHT_ARROW); 00069 wait(.2); 00070 } 00071 if (pb1==0) {keyboard.keyCode(UP_ARROW); //rotates tiles 00072 wait(.2); 00073 } 00074 if (z < 0.2) {keyboard.keyCode('d'); // drops tile 00075 wait(.2); 00076 } 00077 00078 } 00079 00080 }
Generated on Thu Aug 18 2022 09:21:19 by
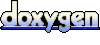