
BLE example with Environmental Sensing service.
Dependencies: BSP_B-L475E-IOT01
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include <events/mbed_events.h> 00018 #include "mbed.h" 00019 #include "ble/BLE.h" 00020 #include "EnvironmentalService_v2.h" 00021 //#include "debug.h" 00022 00023 // Uncomment this line if you want to use the board temperature sensor instead of 00024 // a simulated one. 00025 #define USE_BOARD_SENSORS 00026 00027 #ifdef USE_BOARD_SENSORS 00028 #include "stm32l475e_iot01_tsensor.h" 00029 #include "stm32l475e_iot01_hsensor.h" 00030 #include "stm32l475e_iot01_psensor.h" 00031 #endif 00032 00033 #define ADVERTISING_INTERVAL 1000 00034 #define UPDATE_VALUES_INTERVAL 1000 00035 00036 DigitalOut led1(LED1, 0); 00037 DigitalOut led2(LED2, 0); 00038 00039 const static char DEVICE_NAME[] = "IoT_SENSOR"; 00040 static const uint16_t uuid16_list[] = {GattService::UUID_ENVIRONMENTAL_SERVICE}; 00041 static EnvironmentalService *environmentServicePtr; 00042 00043 /* initial dummy values */ 00044 static float currentTemperature = -15.0; 00045 static float currentHumidity = 0.0; 00046 static float currentPressure = 260.0 * 100; // hPa -> Pascal 00047 00048 static EventQueue eventQueue(/* event count */ 16 * EVENTS_EVENT_SIZE); 00049 static int id_adv; 00050 static int id_conn; 00051 00052 void updateSensorValue(void) { 00053 00054 static int16_t lastTemperature; 00055 static uint16_t lastHumidity; 00056 static uint32_t lastPressure; 00057 00058 int16_t tempTemperature = 0; 00059 uint16_t tempHumidity = 0; 00060 uint32_t tempPressure = 0; 00061 00062 #ifdef USE_BOARD_SENSORS 00063 currentTemperature = BSP_TSENSOR_ReadTemp(); 00064 currentHumidity = BSP_HSENSOR_ReadHumidity(); 00065 currentPressure = BSP_PSENSOR_ReadPressure(); 00066 currentPressure = currentPressure*100; // hPa -> Pascal 00067 #else 00068 /* dummy values */ 00069 currentTemperature = (currentTemperature + 0.1f > 43.0f) ? -15.0f : currentTemperature + 0.1f; 00070 currentHumidity = (currentHumidity + 0.1f > 100.0f) ? 0.0f : currentHumidity + 0.1f; 00071 currentPressure = (currentPressure + 10 > 126000.0f) ? 26000.0f : currentPressure + 10; 00072 #endif 00073 00074 #ifdef DEBUG 00075 pc.printf("\r\n"); 00076 pc.printf("T_sensor = %.2f C\r\n", ((int16_t)(currentTemperature*100))/100.0); 00077 pc.printf("H_sensor = %.2f %%\r\n", ((uint16_t)(currentHumidity*100))/100.0); 00078 pc.printf("P_sensor = %.1f Pa\r\n", ((uint32_t)(currentPressure*10))/10.0); 00079 pc.printf("\r\n"); 00080 #endif 00081 00082 tempTemperature = (int16_t)(currentTemperature*100); 00083 tempHumidity = (uint16_t)(currentHumidity*100); 00084 tempPressure = (uint32_t)(currentPressure*10); 00085 00086 /* Update char values, but only if they differ from previous */ 00087 if(tempTemperature != lastTemperature) 00088 { 00089 environmentServicePtr->updateTemperature(tempTemperature); 00090 lastTemperature = tempTemperature; 00091 } 00092 if(tempHumidity != lastHumidity) 00093 { 00094 environmentServicePtr->updateHumidity(tempHumidity); 00095 lastHumidity = tempHumidity; 00096 } 00097 if(tempPressure != lastPressure) 00098 { 00099 environmentServicePtr->updatePressure(tempPressure); 00100 lastPressure = tempPressure; 00101 } 00102 } 00103 00104 void periodicCallback(void) 00105 { 00106 /* Do blinky on LED1 while advertising */ 00107 led1 = !led1; 00108 wait(0.25); 00109 led1 = !led1; 00110 } 00111 00112 /* On Connection event start updating sensor values */ 00113 void connectionCallback(const Gap::ConnectionCallbackParams_t *) 00114 { 00115 #ifdef DEBUG 00116 pc.printf("\r\n"); 00117 pc.printf("Connection Event.\r\n"); 00118 #endif 00119 eventQueue.cancel(id_adv); 00120 led1 = 0; 00121 led2 = 1; // LED2 on when connected 00122 updateSensorValue(); 00123 id_conn = eventQueue.call_every(UPDATE_VALUES_INTERVAL, updateSensorValue); 00124 } 00125 00126 /* Restart Advertising on disconnection*/ 00127 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *) 00128 { 00129 eventQueue.cancel(id_conn); 00130 led2 = 0; // LED2 off when disconnected 00131 BLE::Instance().gap().startAdvertising(); 00132 id_adv = eventQueue.call_every(ADVERTISING_INTERVAL, periodicCallback); 00133 00134 00135 #ifdef DEBUG 00136 pc.printf("\r\n"); 00137 pc.printf("Disconnection Event - Start Advertising...\r\n"); 00138 #endif 00139 } 00140 00141 void onBleInitError(BLE &ble, ble_error_t error) 00142 { 00143 /* Initialization error handling should go here */ 00144 #ifdef DEBUG 00145 pc.printf("BLE Init Error: %u\r\n", error); 00146 #endif 00147 00148 while(1) 00149 { /* Do blinky on LED2 on Error */ 00150 led2 = !led2; 00151 wait(0.25); 00152 } 00153 } 00154 00155 void bleInitComplete(BLE::InitializationCompleteCallbackContext *params) 00156 { 00157 BLE& ble = params->ble; 00158 ble_error_t error = params->error; 00159 00160 if (error != BLE_ERROR_NONE) { 00161 onBleInitError(ble, error); 00162 return; 00163 } 00164 00165 /* Ensure that it is the default instance of BLE */ 00166 if (ble.getInstanceID() != BLE::DEFAULT_INSTANCE) { 00167 return; 00168 } 00169 00170 /* Callback function when connected */ 00171 ble.gap().onConnection(connectionCallback); 00172 /* Callback function when disconnected */ 00173 ble.gap().onDisconnection(disconnectionCallback); 00174 00175 /* Setup primary service. */ 00176 environmentServicePtr = new EnvironmentalService(ble); 00177 00178 /* setup advertising */ 00179 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); 00180 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, (uint8_t *)uuid16_list, sizeof(uuid16_list)); 00181 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); 00182 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00183 ble.gap().setAdvertisingInterval(ADVERTISING_INTERVAL); 00184 ble.gap().startAdvertising(); 00185 } 00186 00187 void scheduleBleEventsProcessing(BLE::OnEventsToProcessCallbackContext* context) { 00188 BLE &ble = BLE::Instance(); 00189 eventQueue.call(Callback<void()>(&ble, &BLE::processEvents)); 00190 } 00191 00192 int main() 00193 { 00194 #ifdef USE_BOARD_SENSORS 00195 BSP_TSENSOR_Init(); 00196 BSP_HSENSOR_Init(); 00197 BSP_PSENSOR_Init(); 00198 #endif 00199 00200 #ifdef DEBUG 00201 pc.printf("== BLE - EnvironmentalService ==\r\n"); 00202 #endif 00203 00204 id_adv = eventQueue.call_every(ADVERTISING_INTERVAL, periodicCallback); 00205 00206 BLE &ble = BLE::Instance(); 00207 ble.onEventsToProcess(scheduleBleEventsProcessing); 00208 00209 #ifdef DEBUG 00210 pc.printf("Init BLE...\r\n"); 00211 #endif 00212 00213 ble.init(bleInitComplete); 00214 00215 #ifdef DEBUG 00216 pc.printf("Init complete - Advertising...\r\n"); 00217 #endif 00218 00219 eventQueue.dispatch(); 00220 00221 return 0; 00222 }
Generated on Wed Jul 13 2022 01:02:12 by
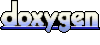