PushDuration maps n callback functions to the duration of a button press. E.g. foo() is called when a button is released after 1 second where bar() is called after 3 seconds.
PushDuration.cpp
00001 #include "mbed.h" 00002 #include "PushDuration.h" 00003 /** 00004 * React() function is called by release() when the button is released. It traverses through the two 00005 * dimensional key vale array and matches the push duration with the corresponding callback function. 00006 **/ 00007 void ButtonHandler::react(int counter) const { 00008 const action *a = mTable.table; 00009 for (std::size_t i=mTable.size; i; --i, ++a) { 00010 if ((counter >= a->counter_limit) && counter < (a+1)->counter_limit ) { 00011 a->transition(); 00012 return; 00013 } 00014 } 00015 } 00016 00017 /** 00018 * Configures the button pin mode, attaches callback functions for rise and fall 00019 * and enables IRQ for the given pin. 00020 **/ 00021 void ButtonHandler::enable() { 00022 buttonPin.mode(PullUp); 00023 wait(0.01); 00024 buttonPin.rise(this, &ButtonHandler::release); 00025 buttonPin.fall(this, &ButtonHandler::press); 00026 buttonPin.enable_irq(); 00027 } 00028 00029 /** 00030 * 00031 * 00032 * 00033 **/ 00034 00035 void ButtonHandler::disable() { 00036 buttonPin.disable_irq(); 00037 ticker.detach(); 00038 } 00039 00040 void ButtonHandler::press() { 00041 counter = 0; 00042 ticker.attach(this, &ButtonHandler::secondsCount, intervalInSeconds); 00043 } 00044 00045 void ButtonHandler::secondsCount() { 00046 ++counter; 00047 } 00048 00049 void ButtonHandler::release() { 00050 ticker.detach(); 00051 react(counter); 00052 counter = 0; 00053 }
Generated on Fri Jul 29 2022 07:49:11 by
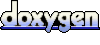