LedBlink library makes it possible to change blink frequency of a given LED by calling the Blink function with a float parameter elsewhere in the code to visualise state changes in a program.
LedBlink.h
00001 /** 00002 * This program demonstrates the usage of the LedBlink Library. 00003 * It counts the number of button interrupts (falling edge) and 00004 * sets the blink frequency to a new value by calling the Blinker 00005 * function of class LedBlink and passing a float as paramter for 00006 * the frequency where 0 = off and 1 = 1 hz. 00007 00008 * Example: 00009 * @code 00010 00011 * #include "mbed.h" 00012 * #include "LedBlink.h" 00013 00014 * InterruptIn pb(p17); 00015 * // SPST Pushbutton count demo using interrupts 00016 * // no external PullUp resistor needed 00017 * // Pushbutton from P17 to GND. 00018 * // A pb falling edge (hit) generates an interrupt and activates the interrupt routine 00019 00020 * // Global count variable 00021 * int volatile count=0; 00022 00023 * //Instantiate LedBlink object. 00024 * LedBlink ledb(p21); 00025 00026 * // pb Interrupt routine - is interrupt activated by a falling edge of pb input 00027 * void pb_hit_interrupt (void) { 00028 * count++; 00029 * //Set Blink Frequency based on button count. 00030 * //Function Blinker accepts a parameter of type float to set the blink frequency. 00031 * if (count == 1){ 00032 * ledb.Blinker(1.0); 00033 * } 00034 * if (count == 2){ 00035 * ledb.Blinker(0.5); 00036 * } 00037 * if (count == 3){ 00038 * ledb.Blinker(0.1); 00039 * } 00040 * if (count == 4){ 00041 * ledb.Blinker(0); 00042 * count = 0; 00043 * } 00044 * } 00045 00046 *int main(void) { 00047 * // Use internal pullup for pushbutton 00048 * pb.mode(PullUp); 00049 * // Delay for initial pullup to take effect 00050 * wait(.01); 00051 * // Attach the address of the interrupt handler routine for pushbutton 00052 * pb.fall(&pb_hit_interrupt); 00053 00054 * while (1){ 00055 * } 00056 *} 00057 *@endcode 00058 */ 00059 #ifndef MBED_LEDBLINK_H 00060 #define MBED_LEDBLINK_H 00061 00062 #include "mbed.h" 00063 00064 class LedBlink 00065 { 00066 public: 00067 /** Create a LedBlink object 00068 * @param frequency 00069 * @param led 00070 */ 00071 LedBlink(PinName pin); 00072 void Blinker(float frequency); 00073 00074 private: 00075 00076 DigitalOut _pin; 00077 00078 00079 00080 /** Internal ticker to set LED blink frequency 00081 * 00082 */ 00083 void LedBlinkCallback(void); 00084 00085 }; 00086 #endif
Generated on Mon Jul 18 2022 00:08:22 by
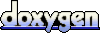