
this version has all of Jim's fixes for reading the GPS and IMU data synchronously
Dependencies: MODSERIAL SDFileSystem mbed SDShell CRC CommHandler FP LinkedList LogUtil
OEM615.h
00001 #include "crc.h" 00002 00003 //GPS-specific pins 00004 DigitalOut GPS_Reset(p18); //GPS RESET line 00005 InterruptIn PPSInt(p15); // GPS 1PPS (timemark) from the OEM615 00006 InterruptIn IMUClock(p17); 00007 00008 Timer timeFromPPS; 00009 unsigned long GPSTimemsecs = 0; 00010 00011 //mbed tx/rx interface to the GPS COM1 port 00012 MODSERIAL GPS_COM1(p9,p10); //this serial port communicates with the GPS receiver serial port (COM1) 00013 00014 bool loadingMessageBuffer = false; 00015 00016 const unsigned short maxGPSbytesPerSec = 2048; 00017 00018 int messagePerSecCounter = 0; 00019 char msgBuffer0[2048]; //array to contain one full second of GPS bytes 00020 char msgBuffer1[512]; //array to contain one full second of GPS bytes 00021 char msgBuffer2[512]; //array to contain one full second of GPS bytes 00022 int GPSbyteCounter0 = 0; 00023 int GPSbyteCounter1 = 0; 00024 int GPSbyteCounter2 = 0; 00025 bool message0Complete = false; 00026 bool message1Complete = false; 00027 bool message2Complete = false; 00028 00029 //////////////////////////////////////////////////////////// 00030 //hotstart procedure 00031 //////////////////////////////////////////////////////////// 00032 // Novatel OEM615 startup messages: 00033 // setapproxtime 1605 425384 // GPSWeek & GPSSeconds 00034 // setapproxpos 51.116 -114.038 0 //lat, lon, alt 00035 //////////////////////////////////////////////////////////// 00036 // how to use? 00037 // Assumes a certain power-up sequence: WALDO_FCS must be running on the PC?? 00038 // unlikely this start-up sequence will work!! 00039 // PC MAY generates a startUp message that is checked for before the GPS RX starts 00040 // mbed looks for the startup message for 1 sec. 00041 // startUp message contains: Last PCTime, lastLat, lastLon, LastAlt, LastGPSWeek, LastGPSSeconds 00042 // If it is found, send the startup messages to the receiver 00043 // if it is not found, then just start without the messages (cold start) 00044 // On the PC side, check for a startUp file stored within the .exe folder. 00045 // However, if the PC clock time between now and the lastStart is > 24 hours, ignore startup file 00046 // if the startUp file IS PRESENT, use it to send the startUp message 00047 // After reading the startUp file on the PC, always delete it. 00048 // Every time the system enters "FINESTEERING", save the StartUp file 00049 00050 void sendASCII(char* ASCI_message, int numChars) 00051 { 00052 ///////////////////////////////////////////////// 00053 //send an ASCII command to the GPS receiver 00054 ///////////////////////////////////////////////// 00055 00056 //char ASCI_message[] = "unlogall COM1"; 00057 int as = numChars - 1; 00058 unsigned char CR = 0x0d; //ASCII Carriage Return 00059 unsigned char LF = 0x0a; //ASCII Line Feed 00060 00061 //printf("%s", ch); 00062 //printf("\n"); 00063 00064 for (int i=0; i<as; i++) GPS_COM1.putc(ASCI_message[i]); 00065 GPS_COM1.putc(CR); //carriage return at end 00066 GPS_COM1.putc(LF); //line feed at end 00067 }; 00068 00069 00070 //see the mbed COOKBOOK for MODSERIAL 00071 //MODSERIAL is an easy to use library that extends Serial to add fully buffered input and output. 00072 void readSerialByte(MODSERIAL_IRQ_INFO *q) 00073 { 00074 MODSERIAL *serial = q->serial; //see example of MODSERIAL usage in cookbook 00075 unsigned char synch0 = serial->getc(); //get the next byte 00076 00077 totalGPSBytes++; 00078 00079 //all OEM615 GPS ASCII messages begin with the unique character: "#" 00080 //read til we get a "#" and then start storing the message 00081 if (synch0 == '#') 00082 { 00083 if (messagePerSecCounter == 0) GPSbyteCounter0 = 0; 00084 else if(messagePerSecCounter == 1) GPSbyteCounter1 = 0; 00085 else if(messagePerSecCounter == 2) GPSbyteCounter2 = 0; 00086 //loadingMessageBuffer = true; 00087 } 00088 00089 if (messagePerSecCounter == 0) { msgBuffer0[GPSbyteCounter0 % 1024] = synch0; GPSbyteCounter0++; } 00090 else if(messagePerSecCounter == 1) { msgBuffer1[GPSbyteCounter1 % 512] = synch0; GPSbyteCounter1++; } 00091 else if(messagePerSecCounter == 2) { msgBuffer2[GPSbyteCounter2 % 512] = synch0; GPSbyteCounter2++; } 00092 00093 //stop storing the message when we get a LF 00094 if (synch0 == 0x0a /* LF*/) //test for line feed 00095 { 00096 //as further confirmation, we could test the prior byte for a CR = 0x0d; 00097 00098 if (messagePerSecCounter == 0) 00099 { 00100 if ( msgBuffer0[GPSbyteCounter0 - 2] == 0x0d) //ensure the two byte end of message 00101 message0Complete = true; 00102 } 00103 else if(messagePerSecCounter == 1) 00104 { 00105 if ( msgBuffer0[GPSbyteCounter0 - 2] == 0x0d) //ensure the two byte end of message 00106 message1Complete = true; 00107 } 00108 else if(messagePerSecCounter == 2) 00109 { 00110 if ( msgBuffer0[GPSbyteCounter0 - 2] == 0x0d) //ensure the two byte end of message 00111 message2Complete = true; 00112 } 00113 messagePerSecCounter++; //count the messages per second 00114 } 00115 00116 //how this can fail?? 00117 // 1) get noisy # occurrences (unique starting character) 00118 // 2) get noisy LF occurrences (unique ending character) 00119 // 3) get noisy data packet values or extra values 00120 // 4) we will also need to vet the data on the PC side 00121 // 5) here, we should do minimal testing and just pass the data as is 00122 00123 }; 00124
Generated on Wed Jul 13 2022 09:05:56 by
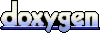