
xbee gateway
Dependencies: NTPClient SDFileSystem WIZnetInterface XBeeLib mbed
main.cpp
00001 #include "mbed.h" 00002 #include "XBeeLib.h" 00003 #include "NTPClient.h" 00004 #include "EthernetInterface.h" 00005 #include "SDFileSystem.h" 00006 00007 #define _SDENABLE 00008 #define _DBG 00009 00010 00011 #define REMOTE_NODE_ADDR64_MSB ((uint32_t)0x0013A200) 00012 #define REMOTE_NODE_ADDR64_LSB ((uint32_t)0x40e82830) 00013 //#define REMOTE_NODE_ADDR64_LSB ((uint32_t)0x40B8EBCE) 00014 00015 #define REMOTE_NODE_ADDR16 ((uint16_t)0xFFFF) 00016 #define REMOTE_NODE_ADDR64 UINT64(REMOTE_NODE_ADDR64_MSB, REMOTE_NODE_ADDR64_LSB) 00017 00018 00019 using namespace XBeeLib; 00020 00021 Serial pc(DEBUG_TX, DEBUG_RX); 00022 00023 DigitalOut LED(D6); 00024 time_t cTime; 00025 Ticker now; 00026 time_t rtime; 00027 unsigned long waterlog = 0; 00028 00029 enum dataType { 00030 REQTOTAL = 0, 00031 SENDDATA 00032 }; 00033 00034 00035 void ethInit(); 00036 int storeSDCard(uint8_t *data, uint16_t len); 00037 static void receiveXbee(const RemoteXBee802& remote, bool broadcast, const uint8_t *const data, uint16_t len); 00038 static void sendRemoteXbee(XBee802& xbee, const RemoteXBee802& RemoteDevice); 00039 time_t getCurTime(); 00040 00041 00042 00043 00044 00045 00046 00047 void ledBlink(int cnt, int delay_ms = 50){ 00048 00049 LED = 0; 00050 00051 for(int i = 0; i < cnt; i++){ 00052 LED = 1; 00053 wait_ms(delay_ms); 00054 LED = 0; 00055 wait_ms(delay_ms); 00056 } 00057 } 00058 00059 static void sendRemoteXbee(XBee802& xbee, const RemoteXBee802& RemoteDevice) 00060 { 00061 char data[20] = {'\0'}; 00062 sprintf(data, "init"); 00063 00064 const uint16_t data_len = strlen(data); 00065 printf("%s\n",data); 00066 const TxStatus txStatus = xbee.send_data(RemoteDevice, (const uint8_t *)data, data_len); 00067 00068 if (txStatus == TxStatusSuccess) 00069 printf(">> Send_data_to_remote_node OK... %s\r\n",data); 00070 else 00071 printf(">> send_data_to_remote_node failed with %d\r\n", (int)txStatus); 00072 } 00073 00074 00075 static void receiveXbee(const RemoteXBee802& remote, bool broadcast, const uint8_t *const data, uint16_t len) 00076 { 00077 char tmp[50] = {'\0'}; 00078 00079 #ifdef _DBG 00080 00081 if (remote.is_valid_addr16b()) { 00082 printf("\r\n>> Got a %s 16-bit RX packet [%04x], len %d\r\nData: ", broadcast ? "BROADCAST" : "UNICAST", remote.get_addr16(), len); 00083 } else { 00084 printf("\r\n>> Got a %s 64-bit RX packet [%08x:%08x], len %d\r\nData: ", broadcast ? "BROADCAST" : "UNICAST", remote.get_addr64(), len); 00085 } 00086 #endif 00087 00088 for (int i = 1; i < len; i++){ 00089 printf("%c", data[i]); 00090 tmp[i - 1] = data[i]; 00091 } 00092 printf("\r\n"); 00093 00094 // int val = atoi(tmp); 00095 //printf("%d",val); 00096 if(data[0] == SENDDATA + 48){ 00097 storeSDCard((uint8_t*)(data + 1), len - 1); 00098 } 00099 else if(data[0] == REQTOTAL + 48){ //plust ascii 00100 // readSDCard(); 00101 // sendRemoteXbee(xbee, remoteDevice64b, val); 00102 } 00103 00104 } 00105 00106 void ethInit(){ 00107 00108 int phy_link; 00109 printf("Wait a second...\r\n"); 00110 00111 uint8_t mac_addr[6] = {0x00,0x08,0xDC,0x1C,0xA8,0x95}; 00112 00113 EthernetInterface eth; 00114 // eth.init(mac_addr,"222.98.173.194","255.255.255.0","222.98.173.254"); //Use DHCP 00115 eth.init(mac_addr); 00116 eth.connect(); 00117 00118 do{ 00119 phy_link = eth.ethernet_link(); 00120 printf("..."); 00121 ledBlink(1); 00122 wait(2); 00123 }while(!phy_link); 00124 printf("\r\n"); 00125 00126 printf("IP Address is %s\r\n", eth.getIPAddress()); 00127 00128 } 00129 // copied from Real time bus arrival alarm by eric 00130 time_t getCurTime(){ 00131 00132 // NTP initialize 00133 NTPClient ntp; 00134 00135 printf("\r\nTrying to update time...\r\n"); 00136 00137 00138 if (ntp.setTime("211.233.40.78") == 0) 00139 { 00140 printf("Set time successfully\r\n"); 00141 time_t ctTime; 00142 ctTime = time(NULL); 00143 ctTime += 32400; // GMT+9/Seoul 00144 printf("Time is set to (GMT+9): %s\r\n", ctime(&ctTime)); 00145 return ctTime; 00146 } 00147 else 00148 { 00149 printf("Error\r\n"); 00150 return 0; 00151 } 00152 00153 00154 } 00155 int readSDCard(){ 00156 00157 00158 } 00159 00160 int storeSDCard(uint8_t *data, uint16_t len){ 00161 char buffer[35]; 00162 char storeString[50] = {'\0'}; 00163 time_t ctTime = getCurTime(); 00164 data[len] = '\0'; 00165 strftime(buffer, 35, "%Y/%m/%d %I:%M %p", localtime(&ctTime)); 00166 00167 //printf("%s\n%s\n", buffer ,data); 00168 00169 mkdir("/sd/WaterLogger", 0777); 00170 00171 FILE *fp = fopen("/sd/WaterLogger/WarerLevel.txt", "a"); 00172 if(fp == NULL) { 00173 error("Could not open file for write\n"); 00174 return 0; 00175 } 00176 ledBlink(5); 00177 sprintf(storeString,"%s %sml\r\n", buffer, data); 00178 fprintf(fp, storeString); 00179 fclose(fp); 00180 waterlog += atoi((char*)data); 00181 00182 #ifdef _DBG 00183 printf("%s",storeString); 00184 printf("Stored Water Level\n"); 00185 #endif 00186 00187 return 1; 00188 } 00189 00190 00191 ////////////////////////////////////// 00192 ////////////////////////////////////// 00193 ////////////////////////////////////// 00194 ////////////////////////////////////// 00195 ////////////////////////////////////// 00196 ////////////////////////////////////// 00197 ////////////////////////////////////// 00198 void addTime(){ 00199 00200 rtime++; 00201 } 00202 00203 00204 int main() 00205 { 00206 00207 // printf("%d",val); 00208 ethInit(); // ethernet initialiaze 00209 00210 pc.baud(9600); 00211 00212 XBee802 xbee = XBee802(RADIO_TX, RADIO_RX, RADIO_RESET, NC, NC, 9600); 00213 SDFileSystem sd(PB_3, PB_2, PB_1, PB_0, "sd"); // the pinout on the mbed Cool Components workshop board 00214 00215 /* Register callback */ 00216 xbee.register_receive_cb(&receiveXbee); 00217 RadioStatus const radioStatus = xbee.init(); 00218 MBED_ASSERT(radioStatus == Success); 00219 const RemoteXBee802 remoteDevice64b = RemoteXBee802(REMOTE_NODE_ADDR64); 00220 00221 rtime = getCurTime(); 00222 struct tm *realtime; 00223 realtime = localtime(&rtime); 00224 set_time(rtime); 00225 now.attach(&addTime, 1); //tick for realtime 00226 00227 //strftime(buffer, 35, "%Y/%m/%d %I:%M %p", localtime(&rtime)); 00228 while (true) { 00229 // strftime(buffer, 35, "%Y/%m/%d %I:%M %p", localtime(&rtime)); 00230 // strftime(buffer, 35, "%I:%M %p %S\n",localtime(&rtime)); 00231 // printf("%s",buffer); 00232 00233 //if( realtime->tm_hour == 0 && realtime->tm_min == 0 && realtime->tm_sec == 0) 00234 // sendRemoteXbee(xbee, remoteDevice64b); 00235 00236 xbee.process_rx_frames(); 00237 wait_ms(100); 00238 } 00239 00240 00241 } 00242 00243 00244 00245 00246
Generated on Tue Jul 12 2022 23:10:54 by
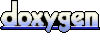