
WizFi310, WiFi module example, based NetworkSocketAPI TCP Server, TCP Client, AP mode, STA mode
Dependencies: NetworkSocketAPI WizFi310Interface mbed-rtos mbed
main.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 #include "TCPSocket.h" 00004 #include "TCPServer.h" 00005 #include "WizFi310Interface.h" 00006 00007 00008 //#define SOFTAPMODE 00009 //#define RUN_SERVER 00010 00011 #ifdef SOFTAPMODE 00012 #define AP_SSID "AP_SSID" 00013 #define AP_PASSWORD "AP_PASSWORD" 00014 #define AP_SECURITY NSAPI_SECURITY_WPA2 00015 #else 00016 #define AP_SSID "AP_SSID" 00017 #define AP_PASSWORD "AP_PASSWORD" 00018 #define AP_SECURITY NSAPI_SECURITY_WPA2 00019 #endif 00020 00021 //server ip, clinet mode 00022 uint8_t ipaddr[4] = {192,168,1,194}; 00023 #define PORT 5000 00024 00025 Serial pc(USBTX,USBRX); 00026 WizFi310Interface wifi(D8, D2, D7, D6, D9, NC, 115200); 00027 00028 char rx_buf[512] = {'\0',}; 00029 00030 TCPServer server; 00031 TCPSocket client; 00032 00033 int TryApUp(); 00034 int TryServerUpAndWaitClient(); 00035 int ClientExample(); 00036 int RunEcho(); 00037 00038 typedef enum ConnectionStep { DISASSOCIATED = 1, ASSOCIATED, LISTENED, CONNECTED, DISCONNECTED } conStep; 00039 conStep ConnectionStep = DISASSOCIATED; 00040 00041 00042 void print_char(char c = '*') 00043 { 00044 printf("%c", c); 00045 fflush(stdout); 00046 } 00047 00048 DigitalOut led1(LED1); 00049 00050 00051 void print_star(void const *argument) 00052 { 00053 00054 while (true) { 00055 Thread::wait(1000); 00056 print_char(); 00057 } 00058 } 00059 00060 void connection_thread(void const *arg) 00061 { 00062 while(true){ 00063 00064 TryApUp(); 00065 00066 #ifdef RUN_SERVER 00067 TryServerUpAndWaitClient(); 00068 #else 00069 ClientExample(); 00070 #endif 00071 00072 RunEcho(); 00073 } 00074 } 00075 00076 int main() 00077 { 00078 pc.baud(115200); 00079 00080 printf("\n\n*** Run WizFi310 on RTOS ***\r\n"); 00081 Thread star_thread(print_star, NULL, osPriorityNormal, DEFAULT_STACK_SIZE); 00082 Thread con_thread(connection_thread, NULL, osPriorityNormal, DEFAULT_STACK_SIZE); 00083 00084 while (true) { 00085 led1 = !led1; 00086 Thread::wait(500); 00087 } 00088 } 00089 00090 00091 00092 int TryApUp() 00093 { 00094 00095 if( ConnectionStep == DISASSOCIATED){ 00096 printf(" AP UP\r\n"); 00097 printf("SSID: %s\r\nPASS: %s\r\n", AP_SSID, AP_PASSWORD); 00098 00099 for(int i= 0; i<5; i++) 00100 { 00101 #ifdef SOFTAPMODE 00102 if ( wifi.connectAP(AP_SSID, AP_PASSWORD, AP_SECURITY) < 0 ) 00103 #else 00104 if ( wifi.connect(AP_SSID, AP_PASSWORD, AP_SECURITY) < 0 ) 00105 #endif 00106 continue; 00107 else { 00108 const char *ip = wifi.get_ip_address(); 00109 printf("my IP address is: %s\r\n", ip ? ip : "No IP"); 00110 ConnectionStep = ASSOCIATED; 00111 return 0; 00112 } 00113 } 00114 printf("Fail to make AP\r\n"); 00115 ConnectionStep = DISASSOCIATED; 00116 } 00117 00118 return -1; 00119 } 00120 00121 int ClientExample() 00122 { 00123 int ret = 0; 00124 if( ConnectionStep == ASSOCIATED ) 00125 { 00126 SocketAddress addr((void*)ipaddr, NSAPI_IPv4, PORT); 00127 //SocketAddress addr(&wifi, "www.google.com", 80); 00128 00129 printf("server IP address is: %s\r\n", addr.get_ip_address()); 00130 00131 00132 00133 client.set_timeout(1000); // Set Block Mode. 00134 00135 client.open(&wifi); 00136 00137 if(client.connect(addr) < 0){ 00138 printf("Connection fail\r\n"); 00139 } 00140 else{ 00141 printf("Connection Success!!\r\nIP: %s, %d\r\n", addr.get_ip_address(), addr.get_port()); 00142 ConnectionStep = CONNECTED; 00143 } 00144 } 00145 } 00146 00147 00148 int TryServerUpAndWaitClient() 00149 { 00150 if( ConnectionStep == ASSOCIATED ) 00151 { 00152 00153 if( server.open(&wifi) < 0 ) 00154 { 00155 printf("WiFi Binding fail..\r\n"); 00156 return -1; 00157 } 00158 00159 00160 if( server.bind(wifi.get_ip_address(), PORT) < 0 ) 00161 { 00162 printf("Bind fail..\r\n"); 00163 return -1; 00164 } 00165 00166 if( server.listen(0) < 0 ) 00167 { 00168 printf("Listen fail..\r\n"); 00169 return -1; 00170 } else { 00171 printf("Listen PORT: %d\r\n",PORT); 00172 ConnectionStep = LISTENED; 00173 } 00174 } 00175 00176 //server.set_blocking(true); 00177 00178 while(ConnectionStep == LISTENED) 00179 { 00180 if( server.accept(&client) < 0 ) 00181 { 00182 printf("accept fail..\r\n"); 00183 return -1; 00184 }else{ 00185 printf("Connection Success!!\r\n"); 00186 ConnectionStep = CONNECTED; 00187 return 0; 00188 } 00189 } 00190 00191 return -1; 00192 } 00193 00194 int RunEcho() 00195 { 00196 int rcv_length = 0; 00197 if(ConnectionStep == CONNECTED) 00198 { 00199 memset(rx_buf, 0, sizeof(rx_buf)); 00200 rcv_length = client.recv(rx_buf, sizeof(rx_buf)); 00201 if(rcv_length > 0) 00202 { 00203 printf("RECV: %s\r\n", rx_buf); 00204 client.send(rx_buf, rcv_length); 00205 } 00206 } 00207 }
Generated on Tue Jul 19 2022 19:13:39 by
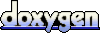