
WIZwiki-W7500 mbed platform & IFTTT maker channel. The aim of this project is to catch a thief when I wasn't home. If the PIR sensor catches a thief. It will send notification to your mobile device.
Dependencies: IFTTT WIZnetInterface mbed
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "TCPSocketConnection.h" 00004 #include "ifttt.h" 00005 00006 #define YourEventName "YOUREVENT" 00007 #define YourSecretKey "YOURKEY" 00008 00009 #define DuringSafety 5 //hours 00010 00011 EthernetInterface eth; 00012 Serial pc(USBTX, USBRX); // tx, rx 00013 DigitalOut led(LED1); 00014 AnalogIn sw(A0); 00015 DigitalIn pir(D8,PullUp); 00016 Timer timer; 00017 00018 int main() 00019 { 00020 int phy_link; 00021 int flag = 0; 00022 int onetimeflag = 0; 00023 float sec = 0.0; 00024 00025 pc.baud(115200); 00026 printf("Wait a second...\r\n"); 00027 uint8_t mac_addr[6] = {0x00, 0x08, 0xDC, 0x12, 0x34, 0x56}; 00028 eth.init(mac_addr); //Use DHCP 00029 eth.connect(); 00030 00031 do{ 00032 phy_link = eth.ethernet_link(); 00033 printf("..."); 00034 wait(2); 00035 }while(!phy_link); 00036 printf("\r\nIP Address is %s \r\n", eth.getIPAddress()); 00037 TCPSocketConnection sock; 00038 00039 // Initialize ifttt object, add up to 3 optional values, trigger event. 00040 IFTTT ifttt(YourEventName,YourSecretKey, &sock); // EventName, Secret Key, socket to use 00041 00042 00043 while(1) { 00044 00045 if(!pir){ 00046 led = 0; 00047 onetimeflag = 0; 00048 printf("Safety\r\n"); 00049 if(!flag){ 00050 timer.start(); 00051 flag = 1; 00052 } 00053 wait(1); 00054 } 00055 else if(pir && (float)sw.read()>=0.9 && !onetimeflag){ 00056 led=1; 00057 onetimeflag=1; 00058 printf("Thief Detected\r\n"); 00059 ifttt.addIngredients("===WARNING===","The Thief was found","Report him to the police"); 00060 ifttt.trigger(); 00061 timer.reset(); 00062 wait(1); 00063 } 00064 sec = timer.read(); 00065 if(sec > DuringSafety*3600 && (float)sw.read()>=0.9){ 00066 timer.reset(); 00067 ifttt.addIngredients("Your home in safety during",(char*)DuringSafety,"hours!"); 00068 ifttt.trigger(); 00069 } 00070 } 00071 00072 }
Generated on Wed Jul 13 2022 16:05:37 by
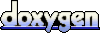