
ddd
Embed:
(wiki syntax)
Show/hide line numbers
MAX30001_RPC.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 #include <stdio.h> 00035 #include "StringHelper.h" 00036 #include "MAX30001.h" 00037 #include "Streaming.h" 00038 #include "StringInOut.h" 00039 #include "MAX30001_helper.h" 00040 #include "RpcFifo.h" 00041 #include "RpcServer.h" 00042 #include "Peripherals.h" 00043 #include "DataLoggingService.h" 00044 00045 /*static int startedEcg = 0; 00046 static int startedBioz = 0; 00047 static int startedCal = 0; 00048 static int startedPace = 0; 00049 static int startedRtor = 0; 00050 static void StopAll(); 00051 */ 00052 00053 extern int highDataRate; 00054 00055 uint32_t max30001_RegRead(MAX30001::MAX30001_REG_map_t addr) { 00056 uint32_t data; 00057 Peripherals::max30001()->max30001_reg_read(addr, &data); 00058 return data; 00059 } 00060 00061 void max30001_RegWrite(MAX30001::MAX30001_REG_map_t addr, uint32_t data) { 00062 Peripherals::max30001()->max30001_reg_write(addr, data); 00063 } 00064 00065 int MAX30001_WriteReg(char argStrs[32][32], char replyStrs[32][32]) { 00066 uint32_t args[2]; 00067 uint32_t reply[1]; 00068 uint32_t value; 00069 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00070 00071 max30001_RegWrite((MAX30001::MAX30001_REG_map_t)args[0], args[1]); 00072 reply[0] = 0x80; 00073 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00074 return 0; 00075 } 00076 00077 int MAX30001_ReadReg(char argStrs[32][32], char replyStrs[32][32]) { 00078 uint32_t args[1]; 00079 uint32_t reply[1]; 00080 uint32_t value; 00081 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00082 value = max30001_RegRead((MAX30001::MAX30001_REG_map_t)args[0]); 00083 reply[0] = value; 00084 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00085 return 0; 00086 } 00087 00088 int MAX30001_Rbias_FMSTR_Init(char argStrs[32][32], char replyStrs[32][32]) { 00089 uint32_t args[5]; 00090 uint32_t reply[1]; 00091 uint32_t value; 00092 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00093 00094 value = Peripherals::max30001()->max30001_Rbias_FMSTR_Init(args[0], // En_rbias 00095 args[1], // Rbiasv 00096 args[2], // Rbiasp 00097 args[3], // Rbiasn 00098 args[4]); // Fmstr 00099 00100 reply[0] = value; 00101 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00102 return 0; 00103 } 00104 00105 int MAX30001_CAL_InitStart(char argStrs[32][32], char replyStrs[32][32]) { 00106 uint32_t args[6]; 00107 uint32_t reply[1]; 00108 uint32_t value; 00109 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00110 00111 // Peripherals::serial()->printf("MAX30001_CAL_InitStart 0 "); 00112 value = Peripherals::max30001()->max30001_CAL_InitStart(args[0], // En_Vcal 00113 args[1], // Vmag 00114 args[2], // Fcal 00115 args[3], // Thigh 00116 args[4], // Fifty 00117 args[5]); // Vmode 00118 00119 reply[0] = value; 00120 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00121 return 0; 00122 } 00123 00124 int MAX30001_ECG_InitStart(char argStrs[32][32], char replyStrs[32][32]) { 00125 uint32_t args[11]; 00126 uint32_t reply[1]; 00127 uint32_t value; 00128 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00129 00130 // Peripherals::serial()->printf("MAX30001_ECG_InitStart 0 "); 00131 value = Peripherals::max30001()->max30001_ECG_InitStart(args[0], // En_ecg 00132 args[1], // Openp 00133 args[2], // Openn 00134 args[3], // Pol 00135 args[4], // Calp_sel 00136 args[5], // Caln_sel 00137 args[6], // E_fit 00138 args[7], // Rate 00139 args[8], // Gain 00140 args[9], // Dhpf 00141 args[10]); // Dlpf 00142 // Peripherals::serial()->printf("MAX30001_ECG_InitStart 1 "); 00143 MAX30001_Helper_SetStreamingFlag(eStreaming_ECG, 1); 00144 reply[0] = value; 00145 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00146 return 0; 00147 } 00148 00149 int MAX30001_ECGFast_Init(char argStrs[32][32], char replyStrs[32][32]) { 00150 uint32_t args[3]; 00151 uint32_t reply[1]; 00152 uint32_t value; 00153 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00154 00155 value = Peripherals::max30001()->max30001_ECGFast_Init(args[0], // Clr_Fast 00156 args[1], // Fast 00157 args[2]); // Fast_Th 00158 00159 reply[0] = value; 00160 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00161 return 0; 00162 } 00163 00164 int MAX30001_PACE_InitStart(char argStrs[32][32], char replyStrs[32][32]) { 00165 uint32_t args[9]; 00166 uint32_t reply[1]; 00167 uint32_t value; 00168 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00169 00170 value = 00171 Peripherals::max30001()->max30001_PACE_InitStart(args[0], // En_pace 00172 args[1], // Clr_pedge 00173 args[2], // Pol 00174 args[3], // Gn_diff_off 00175 args[4], // Gain 00176 args[5], // Aout_lbw 00177 args[6], // Aout 00178 args[7], // Dacp 00179 args[8]); // Dacn 00180 00181 MAX30001_Helper_SetStreamingFlag(eStreaming_PACE, 1); 00182 reply[0] = value; 00183 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00184 return 0; 00185 } 00186 00187 int MAX30001_BIOZ_InitStart(char argStrs[32][32], char replyStrs[32][32]) { 00188 uint32_t args[17]; 00189 uint32_t reply[1]; 00190 uint32_t value; 00191 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00192 00193 value = Peripherals::max30001()->max30001_BIOZ_InitStart(args[0], // En_bioz 00194 args[1], // Openp 00195 args[2], // Openn 00196 args[3], // Calp_sel 00197 args[4], // Caln_sel 00198 args[5], // CG_mode 00199 args[6], // B_fit 00200 args[7], // Rate 00201 args[8], // Ahpf 00202 args[9], // Ext_rbias 00203 args[10], // Gain 00204 args[11], // Dhpf 00205 args[12], // Dlpf 00206 args[13], // Fcgen 00207 args[14], // Cgmon 00208 args[15], // Cgmag 00209 args[16]); // Phoff 00210 00211 MAX30001_Helper_SetStreamingFlag(eStreaming_BIOZ, 1); 00212 reply[0] = value; 00213 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00214 return 0; 00215 } 00216 00217 int MAX30001_RtoR_InitStart(char argStrs[32][32], char replyStrs[32][32]) { 00218 uint32_t args[9]; 00219 uint32_t reply[1]; 00220 uint32_t value; 00221 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00222 00223 value = Peripherals::max30001()->max30001_RtoR_InitStart(args[0], // En_rtor 00224 args[1], // Wndw 00225 args[2], // Gain 00226 args[3], // Pavg 00227 args[4], // Ptsf 00228 args[5], // Hoff 00229 args[6], // Ravg 00230 args[7], // Rhsf 00231 args[8]); // Clr_rrint 00232 00233 MAX30001_Helper_SetStreamingFlag(eStreaming_RtoR, 1); 00234 reply[0] = value; 00235 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00236 return 0; 00237 } 00238 00239 int MAX30001_Stop_ECG(char argStrs[32][32], char replyStrs[32][32]) { 00240 uint32_t reply[1]; 00241 Peripherals::max30001()->max30001_Stop_ECG(); 00242 reply[0] = 0x80; 00243 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00244 return 0; 00245 } 00246 int MAX30001_Stop_PACE(char argStrs[32][32], char replyStrs[32][32]) { 00247 uint32_t reply[1]; 00248 Peripherals::max30001()->max30001_Stop_PACE(); 00249 reply[0] = 0x80; 00250 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00251 return 0; 00252 } 00253 int MAX30001_Stop_BIOZ(char argStrs[32][32], char replyStrs[32][32]) { 00254 uint32_t reply[1]; 00255 Peripherals::max30001()->max30001_Stop_BIOZ(); 00256 reply[0] = 0x80; 00257 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00258 return 0; 00259 } 00260 int MAX30001_Stop_RtoR(char argStrs[32][32], char replyStrs[32][32]) { 00261 uint32_t reply[1]; 00262 Peripherals::max30001()->max30001_Stop_RtoR(); 00263 reply[0] = 0x80; 00264 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00265 return 0; 00266 } 00267 int MAX30001_Stop_Cal(char argStrs[32][32], char replyStrs[32][32]) { 00268 uint32_t reply[1]; 00269 // max30001_Stop_Cal(); 00270 reply[0] = 0x80; 00271 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00272 return 0; 00273 } 00274 00275 void max30001_ServiceStreaming() { 00276 char ch; 00277 uint32_t val; 00278 USBSerial *usbSerial = Peripherals::usbSerial(); 00279 00280 fifo_clear(GetStreamOutFifo()); 00281 00282 SetStreaming(TRUE); 00283 clearOutReadFifo(); 00284 while (IsStreaming() == TRUE) { 00285 00286 if (fifo_empty(GetStreamOutFifo()) == 0) { 00287 fifo_get32(GetStreamOutFifo(), &val); 00288 00289 usbSerial->printf("%02X ", val); 00290 00291 } 00292 if (usbSerial->available()) { 00293 ch = usbSerial->_getc(); 00294 00295 MAX30001_Helper_Stop(); 00296 SetStreaming(FALSE); 00297 fifo_clear(GetUSBIncomingFifo()); // clear USB serial incoming fifo 00298 fifo_clear(GetStreamOutFifo()); 00299 } 00300 00301 } 00302 } 00303 00304 int MAX30001_Start(char argStrs[32][32], char replyStrs[32][32]) { 00305 uint32_t reply[1]; 00306 uint32_t all; 00307 fifo_clear(GetUSBIncomingFifo()); 00308 Peripherals::max30001()->max30001_synch(); 00309 // max30001_ServiceStreaming(); 00310 highDataRate = 0; 00311 Peripherals::max30001()->max30001_reg_read(MAX30001::STATUS, &all); 00312 LoggingService_StartLoggingUsb(); 00313 00314 reply[0] = 0x80; 00315 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00316 return 0; 00317 } 00318 00319 int MAX30001_Stop(char argStrs[32][32], char replyStrs[32][32]) { 00320 /* uint32_t args[1]; 00321 uint32_t reply[1]; 00322 uint32_t value; 00323 //ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00324 max30001_StopTest(); 00325 reply[0] = 0x80; 00326 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs);*/ 00327 return 0; 00328 } 00329 00330 int MAX30001_INT_assignment(char argStrs[32][32], char replyStrs[32][32]) { 00331 uint32_t args[17]; 00332 uint32_t reply[1]; 00333 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00334 /* 00335 printf("MAX30001_INT_assignment "); 00336 printf("%d ",args[0]); 00337 printf("%d ",args[1]); 00338 printf("%d ",args[2]); 00339 printf("%d ",args[3]); 00340 printf("%d ",args[4]); 00341 printf("%d ",args[5]); 00342 printf("%d ",args[6]); 00343 printf("%d ",args[7]); 00344 printf("%d ",args[8]); 00345 printf("%d ",args[9]); 00346 printf("%d ",args[10]); 00347 printf("%d ",args[11]); 00348 printf("%d ",args[12]); 00349 printf("%d ",args[13]); 00350 printf("%d ",args[14]); 00351 printf("%d ",args[15]); 00352 printf("%d ",args[16]); 00353 printf("\n"); 00354 fflush(stdout); 00355 */ 00356 00357 Peripherals::max30001()->max30001_INT_assignment( 00358 (MAX30001::max30001_intrpt_Location_t)args[0], 00359 (MAX30001::max30001_intrpt_Location_t)args[1], 00360 (MAX30001::max30001_intrpt_Location_t)args[2], 00361 (MAX30001::max30001_intrpt_Location_t)args[3], 00362 (MAX30001::max30001_intrpt_Location_t)args[4], 00363 (MAX30001::max30001_intrpt_Location_t)args[5], 00364 (MAX30001::max30001_intrpt_Location_t)args[6], 00365 (MAX30001::max30001_intrpt_Location_t)args[7], 00366 (MAX30001::max30001_intrpt_Location_t)args[8], 00367 (MAX30001::max30001_intrpt_Location_t)args[9], 00368 (MAX30001::max30001_intrpt_Location_t)args[10], 00369 (MAX30001::max30001_intrpt_Location_t)args[11], 00370 (MAX30001::max30001_intrpt_Location_t)args[12], 00371 (MAX30001::max30001_intrpt_Location_t)args[13], 00372 (MAX30001::max30001_intrpt_Location_t)args[14], 00373 (MAX30001::max30001_intrpt_type_t)args[15], 00374 (MAX30001::max30001_intrpt_type_t)args[16]); 00375 reply[0] = 0x80; 00376 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00377 return 0; 00378 } 00379 00380 int MAX30001_StartTest(char argStrs[32][32], char replyStrs[32][32]) { 00381 uint32_t reply[1]; 00382 // ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00383 00384 /*** Set FMSTR over here ****/ 00385 00386 /*** Set and Start the VCAL input ***/ 00387 /* NOTE VCAL must be set first if VCAL is to be used */ 00388 Peripherals::max30001()->max30001_CAL_InitStart(0b1, 0b1, 0b1, 0b011, 0x7FF, 0b0); 00389 00390 /**** ECG Initialization ****/ 00391 Peripherals::max30001()->max30001_ECG_InitStart(0b1, 0b1, 0b1, 0b0, 0b10, 0b11, 31, 0b00, 0b00, 0b0, 0b01); 00392 00393 /***** PACE Initialization ***/ 00394 Peripherals::max30001()->max30001_PACE_InitStart(0b1, 0b0, 0b0, 0b1, 0b000, 0b0, 0b00, 0b0, 0b0); 00395 00396 /**** BIOZ Initialization ****/ 00397 Peripherals::max30001()->max30001_BIOZ_InitStart( 00398 0b1, 0b1, 0b1, 0b10, 0b11, 0b00, 7, 0b0, 0b111, 0b0, 0b10, 0b00, 0b00, 0b0001, 0b0, 0b111, 0b0000); 00399 00400 /*** Set RtoR registers ***/ 00401 Peripherals::max30001()->max30001_RtoR_InitStart( 00402 0b1, 0b0011, 0b1111, 0b00, 0b0011, 0b000001, 0b00, 0b000, 0b01); 00403 00404 /*** Set Rbias & FMSTR over here ****/ 00405 Peripherals::max30001()->max30001_Rbias_FMSTR_Init(0b01, 0b10, 0b1, 0b1, 0b00); 00406 00407 /**** Interrupt Setting ****/ 00408 00409 /*** Set ECG Lead ON/OFF ***/ 00410 // max30001_ECG_LeadOnOff(); 00411 00412 /*** Set BIOZ Lead ON/OFF ***/ 00413 // max30001_BIOZ_LeadOnOff(); Does not work yet... 00414 00415 /**** Do a Synch ****/ 00416 Peripherals::max30001()->max30001_synch(); 00417 00418 fifo_clear(GetUSBIncomingFifo()); 00419 max30001_ServiceStreaming(); 00420 00421 reply[0] = 0x80; 00422 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00423 return 0; 00424 } 00425 /* 00426 static void StopAll() { 00427 if (startedEcg == 1) { 00428 max30001_Stop_ECG(); 00429 } 00430 if (startedCal == 1) { 00431 } 00432 if (startedBioz == 1) { 00433 max30001_Stop_BIOZ(); 00434 } 00435 if (startedPace == 1) { 00436 max30001_Stop_PACE(); 00437 } 00438 if (startedRtor == 1) { 00439 max30001_Stop_RtoR(); 00440 } 00441 startedEcg = 0; 00442 startedBioz = 0; 00443 startedCal = 0; 00444 startedPace = 0; 00445 startedRtor = 0; 00446 } 00447 */ 00448 /* 00449 // switch to ECG DC Lead ON 00450 max30001_Enable_LeadON(0b01); 00451 // switch to BIOZ DC Lead ON 00452 max30001_Enable_LeadON(0b10); 00453 */ 00454 int MAX30001_Enable_ECG_LeadON(char argStrs[32][32], char replyStrs[32][32]) { 00455 uint32_t reply[1]; 00456 // switch to ECG DC Lead ON 00457 Peripherals::max30001()->max30001_Enable_LeadON(0b01); 00458 reply[0] = 0x80; 00459 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00460 return 0; 00461 } 00462 int MAX30001_Enable_BIOZ_LeadON(char argStrs[32][32], char replyStrs[32][32]) { 00463 uint32_t reply[1]; 00464 // switch to BIOZ DC Lead ON 00465 Peripherals::max30001()->max30001_Enable_LeadON(0b10); 00466 reply[0] = 0x80; 00467 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00468 return 0; 00469 } 00470 // uint32_t max30001_LeadOn; // This holds the LeadOn data, BIT1 = BIOZ Lead ON, BIT0 = ECG Lead ON 00471 int MAX30001_Read_LeadON(char argStrs[32][32], char replyStrs[32][32]) { 00472 uint32_t reply[1]; 00473 // return the max30001_LeadOn var from the MAX30001 driver 00474 reply[0] = Peripherals::max30001()->max30001_LeadOn; 00475 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00476 return 0; 00477 }
Generated on Tue Jul 12 2022 21:12:44 by
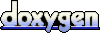