
Program to control an accelerometer, motors and a rangefinder using the ScmRTOS ported to mbed. (Work in progress and buggy)
Command_handler.h
00001 #pragma once 00002 #ifndef COMMAND_HANDLER_H 00003 #define COMMAND_HANDLER_H 00004 #include <messages.h> 00005 #include <processes.h> 00006 00007 //DigitalOut led2(LED2); 00008 extern BusOut leds; 00009 template<> OS_PROCESS void Command_handler::Exec() //serial stream handling process 00010 { 00011 //led2 = 0; 00012 char RX_Command[100] = ""; 00013 RX_Command[99] = '\0'; 00014 00015 for(;;) 00016 { 00017 00018 //RX_channel.pop(temp); 00019 for(byte i = 0; i<100; i++) 00020 { 00021 RX_channel.pop(RX_Command[i]); 00022 leds = 0x4; 00023 if(i == 99) 00024 { 00025 RX_Command[i] = '\0'; 00026 } 00027 //pc.putc(RX_Command[i]); 00028 if(RX_Command[i] == '\0') //keep popping chars until a delimiter is found 00029 break; 00030 } 00031 //led2 = !led2; 00032 TX_channel.write(RX_Command, strlen(RX_Command)+1); //output the command for debugging 00033 TX_channel.push('\n'); 00034 //char temp = 0; 00035 //TX_channel.pop(temp); 00036 //OUT->putc(temp); 00037 //OUT->printf(RX_Command); 00038 00039 if (strstr(RX_Command,"SER:") != NULL) //check for Serv: in the command - this will indicate that the command is destined for the servo process 00040 { 00041 SER_channel.write(RX_Command, strlen(RX_Command)+1); 00042 //Servo_Command_Event.Signal(); 00043 } 00044 00045 else if (strstr(RX_Command,"PIN") != NULL) //check for PING in the command - this will indicate that the command is destined for the PING process 00046 { 00047 00048 PING_MESSAGE = 0; 00049 PING_MESSAGE.send(); 00050 } 00051 00052 else if (strstr(RX_Command,"PIC") != NULL) //check for PING in the command - this will indicate that the command is destined for the PING process 00053 { 00054 00055 PING_MESSAGE = 1; 00056 PING_MESSAGE.send(); 00057 } 00058 00059 else if (strstr(RX_Command, "MOT:") != NULL) //check to see if incoming motor control command 00060 { 00061 MOT_channel.write(RX_Command, strlen(RX_Command)+1); 00062 00063 } 00064 else if (strstr(RX_Command, "MO2:") != NULL) //this command will deal with both motors 00065 { 00066 MOT_channel.write(RX_Command, strlen(RX_Command)+1); 00067 00068 } 00069 00070 else if (strstr(RX_Command, "ACR") != NULL) //check to see if incoming motor control command 00071 { //OS::message<byte> ACCELEROMETER_MESSAGE; //if message is a zero, then return acceleration once, if a 1 then continually return acceleration data 00072 00073 ACCELEROMETER_MESSAGE = 1; 00074 ACCELEROMETER_MESSAGE.send(); //send the message 00075 00076 } 00077 else if (strstr(RX_Command, "ACC") != NULL) //check to see if incoming motor control command 00078 { //OS::message<byte> ACCELEROMETER_Message; //if message is a zero, then return acceleration once, if a 1 then continually return acceleration data 00079 00080 ACCELEROMETER_MESSAGE = 0; 00081 ACCELEROMETER_MESSAGE.send(); //send the message 00082 00083 } 00084 leds = 0x4; 00085 } 00086 00087 } 00088 00089 00090 #endif 00091
Generated on Wed Jul 13 2022 05:20:16 by
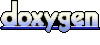