
screen display for roommate tracker
Dependencies: mbed-rtos mbed uLCD_4D_Picaso
Adafruit_FONA.h
00001 /*************************************************** 00002 This is a library for our Adafruit FONA Cellular Module 00003 00004 Designed specifically to work with the Adafruit FONA 00005 ----> http://www.adafruit.com/products/1946 00006 ----> http://www.adafruit.com/products/1963 00007 00008 These displays use TTL Serial to communicate, 2 pins are required to 00009 interface 00010 Adafruit invests time and resources providing this open source code, 00011 please support Adafruit and open-source hardware by purchasing 00012 products from Adafruit! 00013 00014 Written by Limor Fried/Ladyada for Adafruit Industries. 00015 BSD license, all text above must be included in any redistribution 00016 ****************************************************/ 00017 00018 /* 00019 * Modified by George Tzintzarov & Jesse Baker 03/14/2016 for use in mbed LPC1768 00020 */ 00021 00022 #ifndef ADAFRUIT_FONA_H 00023 #define ADAFRUIT_FONA_H 00024 00025 #include "mbed.h" 00026 00027 //#define ADAFRUIT_FONA_DEBUG 00028 00029 #define FONA_HEADSETAUDIO 0 00030 #define FONA_EXTAUDIO 1 00031 00032 #define FONA_STTONE_DIALTONE 1 00033 #define FONA_STTONE_BUSY 2 00034 #define FONA_STTONE_CONGESTION 3 00035 #define FONA_STTONE_PATHACK 4 00036 #define FONA_STTONE_DROPPED 5 00037 #define FONA_STTONE_ERROR 6 00038 #define FONA_STTONE_CALLWAIT 7 00039 #define FONA_STTONE_RINGING 8 00040 #define FONA_STTONE_BEEP 16 00041 #define FONA_STTONE_POSTONE 17 00042 #define FONA_STTONE_ERRTONE 18 00043 #define FONA_STTONE_INDIANDIALTONE 19 00044 #define FONA_STTONE_USADIALTONE 20 00045 00046 #define FONA_DEFAULT_TIMEOUT_MS 500 //timeout between send AT and reply from FONA 00047 00048 #define FONA_HTTP_GET 0 00049 #define FONA_HTTP_POST 1 00050 #define FONA_HTTP_HEAD 2 00051 00052 #define RX_BUFFER_SIZE 255 00053 00054 /** Adafruit FONA 800H Class 00055 * Modified by George Tzintzarov & Jesse Baker 03/14/2016 for use in mbed LPC1768 00056 */ 00057 00058 class Adafruit_FONA : public Stream { 00059 public: 00060 /** 00061 Listener for FONA events. Inherit this class to customize. 00062 @code 00063 #define FONA_RST p12 00064 #define FONA_TX p13 00065 #define FONA_RX p14 00066 #define FONA_RI p11 00067 00068 Adafruit_FONA my_fona(FONA_TX, FONA_RX, FONA_RST, FONA_RI); 00069 DigitalOut led1(LED1); 00070 class FonaEventListener : public Adafruit_FONA::EventListener { 00071 virtual void onRing() { 00072 led1 = 1; 00073 } 00074 00075 virtual void onNoCarrier() { 00076 led1 = 0; 00077 } 00078 }; 00079 FonaEventListener fonaEventListener; 00080 my_fona.setEventListener(&fonaEventListener); 00081 @endcode 00082 */ 00083 00084 class EventListener { 00085 public: 00086 /** 00087 * Method called when somebody call the FONA. 00088 */ 00089 virtual void onRing() = 0; 00090 00091 /** 00092 * Method called when the calling person stop his call. 00093 */ 00094 virtual void onNoCarrier() = 0; 00095 }; 00096 00097 public: 00098 /** Create instance of the Adafruit_FONA 00099 @param tx Set mbed TX 00100 @param rx Set mbed RX 00101 @param rst Set reset pin 00102 @param ringIndicator Set ring indicator pin. This is to let mbed know if there is an incoming call 00103 */ 00104 00105 Adafruit_FONA(PinName tx, PinName rx, PinName rst, PinName ringIndicator) : 00106 _rstpin(rst, false), _ringIndicatorInterruptIn(ringIndicator), 00107 apn("FONAnet"), apnusername(NULL), apnpassword(NULL), httpsredirect(false), useragent("FONA"), 00108 _incomingCall(false), eventListener(NULL), mySerial(tx, rx), rxBufferInIndex(0), rxBufferOutIndex(0), 00109 currentReceivedLineSize(0) {} 00110 00111 /** Built-in Test to see if FONA is connected 00112 @param baudrate test and set at baudrate 00113 @return true upon success 00114 @return false upon failure. Most likely something is not hooked up. 00115 00116 EXAMPLE CODE: 00117 @code 00118 // See if the FONA is responding 00119 // fona is an instance of Adafruit_FONA 00120 if (! fona.begin(9600)) { 00121 printf("Couldn't find FONA\r\n"); 00122 while (1); 00123 } 00124 @endcode 00125 */ 00126 bool begin(int baudrate); 00127 00128 /** Set the event listener for incoming calls 00129 @param eventListener A pointer to the event listener 00130 @see Adafruit_FONA::EventListener for specific example 00131 */ 00132 00133 void setEventListener(EventListener *eventListener); 00134 00135 // Stream---------------------------------------------------------------------- 00136 virtual int _putc(int value); 00137 virtual int _getc(); 00138 00139 /** Check if FONA has anything in its output buffer 00140 @return 0 if nothing 00141 */ 00142 int readable(void); 00143 00144 // RTC---------------------------------------------------------------------- 00145 bool enableRTC(uint8_t i); // i = 0 <=> disable, i = 1 <=> enable 00146 00147 // Battery and ADC---------------------------------------------------------------------- 00148 /** Get ADC voltage from external pin 00149 @param v uint16_t pointer to insert ADC voltage data 00150 @return TRUE if successful 00151 00152 EXAMPLE CODE: 00153 @code 00154 // read the ADC 00155 // fona is an instance of Adafruit_FONA 00156 uint16_t adc; 00157 if (! fona.getADCVoltage(&adc)) { 00158 printf("Failed to read ADC\r\n"); 00159 } 00160 else { 00161 printf("ADC = %d mV\r\n", adc); 00162 } 00163 @endcode 00164 */ 00165 bool getADCVoltage(uint16_t *v); 00166 00167 /** Get battery percentage level 00168 @param p uint16_t pointer to insert battery percent data 00169 @return TRUE if successful 00170 00171 EXAMPLE CODE: 00172 @code 00173 // read the battery percent level 00174 // fona is an instance of Adafruit_FONA 00175 uint16_t vbatPer; 00176 if (! fona.getBattPercent(&vbatPer)) { 00177 printf("Failed to read Batt\r\n"); 00178 } 00179 else { 00180 printf("VPct = %d%%\r\n", vbatPer); 00181 } 00182 @endcode 00183 */ 00184 00185 bool getBattPercent(uint16_t *p); 00186 00187 /** Get battery voltage level 00188 @param v uint16_t pointer to insert battery voltage data 00189 @return TRUE if successful 00190 00191 EXAMPLE CODE: 00192 @code 00193 // read the battery voltage 00194 // fona is an instance of Adafruit_FONA 00195 uint16_t vbat; 00196 if (! fona.getBattPercent(&vbat)) { 00197 printf("Failed to read Batt\r\n"); 00198 } 00199 else { 00200 printf("Vbat = %d%%\r\n", vbat); 00201 } 00202 @endcode 00203 */ 00204 bool getBattVoltage(uint16_t *v); 00205 00206 // SIM query---------------------------------------------------------------------- 00207 /** Unlock SIM if needed 00208 @param pin 4 digit char arrary 00209 @return TRUE if successful 00210 */ 00211 bool unlockSIM(char *pin); 00212 /** Get the SIM chip card interface device (CCID) 00213 @param ccid make sure it is at least 21 bytes long 00214 @return length of CCID 00215 */ 00216 00217 uint8_t getSIMCCID(char *ccid); 00218 /** Get the Network Status of FONA 00219 @return Code 0-5 00220 @see https://www.adafruit.com/datasheets/sim800_series_at_command_manual_v1.01.pdf page 80 00221 */ 00222 uint8_t getNetworkStatus(void); 00223 00224 /** Get the RSSI of the network signal 00225 @return RSSI value in dBm per below reference 00226 00227 EXAMPLE 00228 @code 00229 // read the RSSI 00230 uint8_t n = fona.getRSSI(); 00231 int8_t r = 0; 00232 00233 pcSerial.printf("RSSI = %d: ", n); 00234 if (n == 0) r = -115; 00235 if (n == 1) r = -111; 00236 if (n == 31) r = -52; 00237 if ((n >= 2) && (n <= 30)) { 00238 r = map(n, 2, 30, -110, -54); 00239 } 00240 printf("%d dBm\r\n", r); 00241 00242 // helper function MAP to do calculations 00243 long MAP(long x, long in_min, long in_max, long out_min, long out_max) 00244 { 00245 return (x - in_min) * (out_max - out_min) / (in_max - in_min) + out_min; 00246 } 00247 @endcode 00248 @see https://www.adafruit.com/datasheets/sim800_series_at_command_manual_v1.01.pdf page 82 00249 */ 00250 uint8_t getRSSI(void); 00251 00252 // IMEI---------------------------------------------------------------------- 00253 /** Get the International Mobile Station Equipment Identity (IMEI) 00254 @param imei A char array with minimum length 16 00255 @return The IMEI of the device 00256 00257 EXAMPLE CODE: 00258 @code 00259 // Print SIM card IMEI number. 00260 char imei[15] = {0}; // MUST use a 16 character buffer for IMEI! 00261 uint8_t imeiLen = fona.getIMEI(imei); //fona is an instance of Adafruit_FONA 00262 if (imeiLen > 0) { 00263 pcSerial.printf("SIM card IMEI: %s\r\n", imei); 00264 } 00265 @endcode 00266 */ 00267 uint8_t getIMEI(char *imei); 00268 00269 // set Audio output---------------------------------------------------------------------- 00270 /** Set the Audio Output interface 00271 @param a 0 is headset, 1 is external audio 00272 @return TRUE if successful 00273 */ 00274 bool setAudio(uint8_t a); 00275 00276 /** Set the Audio Volume 00277 @param i a unit8_t volume number 00278 @return TRUE if successful 00279 */ 00280 bool setVolume(uint8_t i); 00281 00282 /** Get the Audio Volume 00283 @return the current volume 00284 */ 00285 uint8_t getVolume(void); 00286 bool playToolkitTone(uint8_t t, uint16_t len); 00287 bool setMicVolume(uint8_t a, uint8_t level); 00288 bool playDTMF(char tone); 00289 00290 // FM radio functions---------------------------------------------------------------------- 00291 /** Tune the FM radio 00292 @param station frequency, for example 107.9 MHz -> 1079 00293 @return TRUE if successful 00294 */ 00295 bool tuneFMradio(uint16_t station); 00296 00297 /** FM radio set output 00298 @param onoff bool to turn on if TRUE 00299 @param a 0 (default) is headset, 1 is external audio 00300 @return TRUE if successful 00301 */ 00302 bool FMradio(bool onoff, uint8_t a = FONA_HEADSETAUDIO); 00303 00304 /** Set the FM Radio Volume 00305 @param i a unit8_t volume number 00306 @return TRUE if successful 00307 */ 00308 bool setFMVolume(uint8_t i); 00309 00310 /** Get the FM Volume 00311 @return the current FM volume 00312 */ 00313 int8_t getFMVolume(); 00314 00315 /** Get the FM signal strength 00316 @param station a unit8_t volume number 00317 @return TRUE if successful 00318 */ 00319 int8_t getFMSignalLevel(uint16_t station); 00320 00321 // SMS handling---------------------------------------------------------------------- 00322 /** Set the SMS Interrupt 00323 @param i 0 = OFF, 1 = ON with TCPIP, FTP, and URC control Ring Indicator Pin, 2 = ON with only TCPIP control 00324 @return TRUE if successful 00325 @see https://www.adafruit.com/datasheets/sim800_series_at_command_manual_v1.01.pdf page 152 00326 */ 00327 bool setSMSInterrupt(uint8_t i); 00328 00329 /** Get SMS Interrupt Setting 00330 @return setting 00331 @see https://www.adafruit.com/datasheets/sim800_series_at_command_manual_v1.01.pdf page 152 00332 */ 00333 uint8_t getSMSInterrupt(void); 00334 00335 /** Set the SMS Interrupt 00336 @return number of SMS messages in inbox 00337 */ 00338 int8_t getNumSMS(void); 00339 00340 /** Read SMS 00341 @param i sms number in memory 00342 @param smsbuff char pointer to char array 00343 @param max Maximum length of smsbuff 00344 @param readsize the size in bytes of the SMS 00345 @return TRUE if successful 00346 */ 00347 bool readSMS(uint8_t i, char *smsbuff, uint16_t max, uint16_t *readsize); 00348 00349 /** Send SMS 00350 @param smsaddr Phone number to send out 00351 @param smsmsg Char array containing message 00352 @return TRUE if successful 00353 */ 00354 bool sendSMS(char *smsaddr, char *smsmsg); 00355 00356 /** Delete SMS 00357 @param i number of SMS in memory 00358 @return TRUE if successful 00359 */ 00360 bool deleteSMS(uint8_t i); 00361 00362 /** Send SMS 00363 @param i Number of SMS in memory 00364 @param sender Char array to store the sender number 00365 @param senderlen length of sender 00366 @return TRUE if successful 00367 */ 00368 bool getSMSSender(uint8_t i, char *sender, int senderlen); 00369 00370 // Time---------------------------------------------------------------------- 00371 /** Enable FONA to sync time with the cellular network 00372 @param onoff on = true, off = false 00373 @return TRUE if successful 00374 */ 00375 bool enableNetworkTimeSync(bool onoff); 00376 00377 /** Enable FONA to sync time with the time server 00378 @param onoff true = on, false = off 00379 @return TRUE if successful 00380 */ 00381 bool enableNTPTimeSync(bool onoff, const char* ntpserver=0); 00382 00383 /** Retrieve the current time from the enabled server 00384 @param buff char array to store time value. Given as "yy/MM/dd,hh:mm:ss+zz" 00385 @param maxlen Maximum length of the char array 00386 @return TRUE if successful 00387 */ 00388 bool getTime(char* buff, uint16_t maxlen); 00389 00390 // GPRS handling---------------------------------------------------------------------- 00391 bool enableGPRS(bool onoff); 00392 uint8_t GPRSstate(void); 00393 bool getGSMLoc(uint16_t *replycode, char *buff, uint16_t maxlen); 00394 bool getGSMLoc(float *lat, float *lon); 00395 void setGPRSNetworkSettings(const char* apn, const char* username=0, const char* password=0); 00396 00397 // GPS handling---------------------------------------------------------------------- 00398 bool enableGPS(bool onoff); 00399 int8_t GPSstatus(void); 00400 uint8_t getGPS(uint8_t arg, char *buffer, uint8_t maxbuff); 00401 bool getGPS(float *lat, float *lon, float *speed_kph=0, float *heading=0, float *altitude=0); 00402 bool enableGPSNMEA(uint8_t nmea); 00403 00404 // TCP raw connections---------------------------------------------------------------------- 00405 bool TCPconnect(char *server, uint16_t port); 00406 bool TCPclose(void); 00407 bool TCPconnected(void); 00408 bool TCPsend(char *packet, uint8_t len); 00409 uint16_t TCPavailable(void); 00410 uint16_t TCPread(uint8_t *buff, uint8_t len); 00411 00412 // HTTP low level interface (maps directly to SIM800 commands).---------------------------------------------------------------------- 00413 bool HTTP_init(); 00414 bool HTTP_term(); 00415 void HTTP_para_start(const char* parameter, bool quoted = true); 00416 bool HTTP_para_end(bool quoted = true); 00417 bool HTTP_para(const char* parameter, const char *value); 00418 bool HTTP_para(const char* parameter, int32_t value); 00419 bool HTTP_data(uint32_t size, uint32_t maxTime=10000); 00420 bool HTTP_action(uint8_t method, uint16_t *status, uint16_t *datalen, int32_t timeout = 10000); 00421 bool HTTP_readall(uint16_t *datalen); 00422 bool HTTP_ssl(bool onoff); 00423 00424 // HTTP high level interface (easier to use, less flexible).---------------------------------------------------------------------- 00425 bool HTTP_GET_start(char *url, uint16_t *status, uint16_t *datalen); 00426 void HTTP_GET_end(void); 00427 bool HTTP_POST_start(char *url, const char* contenttype, const uint8_t *postdata, uint16_t postdatalen, uint16_t *status, uint16_t *datalen); 00428 void HTTP_POST_end(void); 00429 void setUserAgent(const char* useragent); 00430 00431 // HTTPS---------------------------------------------------------------------- 00432 void setHTTPSRedirect(bool onoff); 00433 00434 // PWM (buzzer)---------------------------------------------------------------------- 00435 /** Control the buzzer capability of the PWM out on the FONA 00436 @param period of the buzzing cycle (max 2000) 00437 @param duty the duty cycle of the buzzer (0 to 100) 00438 @return TRUE if successful 00439 */ 00440 bool setPWM(uint16_t period, uint8_t duty = 50); 00441 00442 // Phone calls---------------------------------------------------------------------- 00443 /** Call a phone 00444 @param phonenum a character array of the phone number 00445 @return TRUE if successful 00446 */ 00447 bool callPhone(char *phonenum); 00448 00449 /** Hang up a phone call 00450 */ 00451 bool hangUp(void); 00452 00453 /** Answer a phone call 00454 */ 00455 bool pickUp(void); 00456 00457 /** Enable/disable caller ID 00458 @param enable true to enable, false to disable 00459 @return TRUE if successful 00460 */ 00461 bool callerIdNotification(bool enable); 00462 00463 /** Retrieve the incoming call number 00464 @param phonenum a character array of the phone number calling 00465 @return TRUE if successful 00466 */ 00467 bool incomingCallNumber(char* phonenum); 00468 00469 // Helper functions to verify responses. 00470 bool expectReply(const char* reply, uint16_t timeout = 10000); 00471 00472 private: 00473 DigitalOut _rstpin; 00474 InterruptIn _ringIndicatorInterruptIn; 00475 00476 char replybuffer[255]; // the output of getreply(), readline() is the function that changes the replybuffer 00477 char* apn; 00478 char* apnusername; 00479 char* apnpassword; 00480 bool httpsredirect; 00481 char* useragent; 00482 00483 volatile bool _incomingCall; 00484 EventListener *eventListener; 00485 Serial mySerial; 00486 00487 // Circular buffer used to receive serial data from an interruption 00488 int rxBuffer[RX_BUFFER_SIZE + 1]; 00489 volatile int rxBufferInIndex; // Index where new data is added to the buffer 00490 volatile int rxBufferOutIndex; // Index where data is removed from the buffer 00491 char currentReceivedLine[RX_BUFFER_SIZE]; // Array containing the current received line 00492 int currentReceivedLineSize; 00493 00494 inline bool isRxBufferFull() { 00495 return ((rxBufferInIndex + 1) % RX_BUFFER_SIZE) == rxBufferOutIndex; 00496 } 00497 00498 inline bool isRxBufferEmpty() { 00499 return rxBufferInIndex == rxBufferOutIndex; 00500 } 00501 00502 inline void incrementRxBufferInIndex() { 00503 rxBufferInIndex = (rxBufferInIndex + 1) % RX_BUFFER_SIZE; 00504 } 00505 00506 inline void incrementRxBufferOutIndex() { 00507 rxBufferOutIndex = (rxBufferOutIndex + 1) % RX_BUFFER_SIZE; 00508 } 00509 00510 /** 00511 * Method called when Serial data is received (interrupt routine). 00512 */ 00513 void onSerialDataReceived(); 00514 00515 // HTTP helpers 00516 bool HTTP_setup(char *url); 00517 00518 void flushInput(); 00519 uint16_t readRaw(uint16_t b); 00520 uint8_t readline(uint16_t timeout = FONA_DEFAULT_TIMEOUT_MS, bool multiline = false); 00521 uint8_t getReply(const char* send, uint16_t timeout = FONA_DEFAULT_TIMEOUT_MS); 00522 uint8_t getReply(const char* prefix, char *suffix, uint16_t timeout = FONA_DEFAULT_TIMEOUT_MS); 00523 uint8_t getReply(const char* prefix, int32_t suffix, uint16_t timeout = FONA_DEFAULT_TIMEOUT_MS); 00524 uint8_t getReply(const char* prefix, int32_t suffix1, int32_t suffix2, uint16_t timeout); // Don't set default value or else function call is ambiguous. 00525 uint8_t getReplyQuoted(const char* prefix, const char* suffix, uint16_t timeout = FONA_DEFAULT_TIMEOUT_MS); 00526 00527 bool sendCheckReply(const char* send, const char* reply, uint16_t timeout = FONA_DEFAULT_TIMEOUT_MS); 00528 bool sendCheckReply(const char* prefix, char *suffix, const char* reply, uint16_t timeout = FONA_DEFAULT_TIMEOUT_MS); 00529 bool sendCheckReply(const char* prefix, int32_t suffix, const char* reply, uint16_t timeout = FONA_DEFAULT_TIMEOUT_MS); 00530 bool sendCheckReply(const char* prefix, int32_t suffix, int32_t suffix2, const char* reply, uint16_t timeout = FONA_DEFAULT_TIMEOUT_MS); 00531 bool sendCheckReplyQuoted(const char* prefix, const char* suffix, const char* reply, uint16_t timeout = FONA_DEFAULT_TIMEOUT_MS); 00532 00533 bool parseReply(const char* toreply, uint16_t *v, char divider = ',', uint8_t index=0); 00534 bool parseReply(const char* toreply, char *v, char divider = ',', uint8_t index=0); 00535 bool parseReplyQuoted(const char* toreply, char* v, int maxlen, char divider, uint8_t index); 00536 00537 bool sendParseReply(const char* tosend, const char* toreply, uint16_t *v, char divider = ',', uint8_t index = 0); 00538 00539 void onIncomingCall(); 00540 }; 00541 00542 #endif
Generated on Fri Jul 15 2022 04:25:10 by
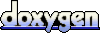