
1 player Black jack for mbed
Dependencies: 4DGL-uLCD-SE PinDetect mbed
main.cpp
00001 #include "mbed.h" 00002 #include "uLCD_4DGL.h" 00003 #include <string> 00004 #define PURPLE 0x800080 00005 #define ORANGE 0xFFA500 00006 #include "deck.h" 00007 #include <mpr121.h> 00008 #include "PinDetect.h" 00009 uLCD_4DGL uLCD(p28, p27, p30); 00010 PinDetect pb1(p8); 00011 PinDetect pb2(p11); 00012 DigitalOut led1(LED1); 00013 DigitalOut led2(LED2); 00014 DigitalOut led3(LED3); 00015 DigitalOut led4(LED4); 00016 // Setup the i2c bus on pins 9 and 10 00017 I2C i2c(p9, p10); 00018 // Setup the Mpr121: 00019 // constructor(i2c object, i2c address of the mpr121) 00020 Mpr121 mpr121(&i2c, Mpr121::ADD_VSS); 00021 //InterruptIn interrupt(p26); 00022 Deck gameDeck; 00023 Card playerHand[10]; 00024 Card dealerHand[10]; 00025 int playerCount=2; 00026 int dealerCount=2; 00027 bool isPlayer=true; 00028 int playerPoints; 00029 int dealerPoints; 00030 int money = 1000; 00031 int bet=100; 00032 int sumHand(Card[]); 00033 00034 void printPoints(int points) 00035 { 00036 if(points>21) { 00037 uLCD.printf("Pts=bust"); 00038 } else { 00039 uLCD.printf("Pts=%d",points); 00040 } 00041 } 00042 void initialDeal() 00043 { 00044 playerCount=2; 00045 dealerCount=2; 00046 playerHand[0]= gameDeck.cards[0]; 00047 dealerHand[0]=gameDeck.cards[1]; 00048 playerHand[1] = gameDeck.cards[2]; 00049 dealerHand[1] = gameDeck.cards[3]; 00050 uLCD.locate(1,8); 00051 uLCD.printf("%s%s",playerHand[0].convertRank(),playerHand[0].convertSuit()); 00052 uLCD.locate(4,8); 00053 uLCD.printf("%s%s",playerHand[1].convertRank(),playerHand[1].convertSuit()); 00054 uLCD.locate(1,3); 00055 uLCD.printf("%s%s",dealerHand[0].convertRank(),dealerHand[1].convertSuit()); 00056 00057 uLCD.locate(6,10); 00058 playerPoints = sumHand(playerHand); 00059 printPoints(playerPoints); 00060 } 00061 00062 00063 void hit() 00064 { 00065 if(isPlayer) { 00066 playerHand[playerCount]=gameDeck.cards[playerCount+dealerCount]; 00067 playerCount++; 00068 uLCD.locate((playerCount-1)*3+1,8); 00069 uLCD.printf("%s%s",playerHand[playerCount-1].convertRank(),playerHand[playerCount-1].convertSuit()); 00070 uLCD.locate(6,10); 00071 playerPoints = sumHand(playerHand); 00072 printPoints(playerPoints); 00073 wait(1); 00074 } else { 00075 dealerHand[dealerCount] = gameDeck.cards[playerCount+dealerCount]; 00076 dealerCount++; 00077 uLCD.locate((dealerCount-1)*3+1,3); 00078 uLCD.printf("%s%s",dealerHand[dealerCount-1].convertRank(),dealerHand[dealerCount-1].convertSuit()); 00079 uLCD.locate(6,6.5); 00080 dealerPoints = sumHand(dealerHand); 00081 printPoints(dealerPoints); 00082 wait(1); 00083 } 00084 } 00085 00086 void getBet() 00087 { 00088 uLCD.cls(); 00089 uLCD.printf("How much will you bet?\n(10-500)"); 00090 int len=0; 00091 int amount=0; 00092 while(len<3) { 00093 int key=0; 00094 int i=0; 00095 int value=mpr121.read(0x00); 00096 value +=mpr121.read(0x01)<<8; 00097 // LED demo mod 00098 i=0; 00099 // puts key number out to LEDs for demo 00100 for (i=0; i<12; i++) { 00101 if (((value>>i)&0x01)==1) key=i+1; 00102 00103 } 00104 if(key != 0 ) { 00105 if(key>10) { 00106 break; 00107 } else { 00108 len++; 00109 amount*=10; 00110 amount+=key-1; 00111 uLCD.printf("%d",key-1); 00112 wait(.25); 00113 } 00114 } 00115 } 00116 bet=max(min(amount,500),10); 00117 bet = min(bet,money); 00118 uLCD.printf("\nBet = %d",bet); 00119 00120 } 00121 void setup() 00122 { 00123 uLCD.color(ORANGE); 00124 00125 00126 getBet(); 00127 uLCD.cls(); 00128 uLCD.locate(6,0.5); 00129 uLCD.printf("Dealer"); 00130 00131 uLCD.locate(6,7.5); 00132 uLCD.printf("Player"); 00133 00134 uLCD.filled_rectangle(0,112,127,148,DGREY); 00135 gameDeck.shuffleDeck(); 00136 initialDeal(); 00137 00138 } 00139 00140 // Callback routine is interrupt activated by a debounced pb1 hit 00141 void pb1_hit_callback (void) 00142 { 00143 hit(); 00144 00145 } 00146 00147 // Callback routine is interrupt activated by a debounced pb2 hit 00148 void pb2_hit_callback (void) 00149 { 00150 isPlayer=false; 00151 00152 } 00153 void walletSize() 00154 { 00155 uLCD.cls(); 00156 uLCD.printf("NOTE:\nEnter input on pad followed by key 10\n\n"); 00157 uLCD.printf("top pushbutton is hit and bottom is stand"); 00158 00159 wait(5); 00160 uLCD.cls(); 00161 uLCD.printf("How much will you start with?\n(1000-10000)"); 00162 int len=0; 00163 int amount=0; 00164 while(len<5) { 00165 int key=0; 00166 int i=0; 00167 int value=mpr121.read(0x00); 00168 value +=mpr121.read(0x01)<<8; 00169 // LED demo mod 00170 i=0; 00171 // puts key number out to LEDs for demo 00172 for (i=0; i<12; i++) { 00173 if (((value>>i)&0x01)==1) key=i+1; 00174 00175 } 00176 if(key != 0 ) { 00177 if(key>10) { 00178 break; 00179 } else { 00180 len++; 00181 amount*=10; 00182 amount+=key-1; 00183 uLCD.printf("%d",key-1); 00184 wait(.25); 00185 } 00186 } 00187 } 00188 money=max(min(amount,50000),1000); 00189 00190 uLCD.printf("\nWallet = %d",money); 00191 00192 } 00193 void game(); 00194 int main() 00195 { 00196 pb1.mode(PullUp); 00197 pb2.mode(PullUp); 00198 // Delay for initial pullup to take effect 00199 wait(.01); 00200 // Setup Interrupt callback functions for a pb hit 00201 pb1.attach_deasserted(&pb1_hit_callback); 00202 pb2.attach_deasserted(&pb2_hit_callback); 00203 // Start sampling pb inputs using interrupts 00204 pb1.setSampleFrequency(); 00205 pb2.setSampleFrequency(); 00206 //uLCD.color(ORANGE); 00207 //uLCD.printf("How much money to play with?(minimum bet $10)"); 00208 walletSize(); 00209 game(); 00210 00211 } 00212 int sumHand(Card[]); 00213 void result(int); 00214 void dealerTurn(); 00215 void game() 00216 { 00217 while(money>=100) { 00218 isPlayer = true; 00219 setup(); 00220 uLCD.locate(0,14); 00221 uLCD.printf("Cash=%d",money); 00222 uLCD.locate(10,14); 00223 uLCD.printf("Bet=%d",bet); 00224 while(isPlayer) { 00225 if(sumHand(playerHand)>21) { 00226 result(2); 00227 } 00228 } 00229 dealerTurn(); 00230 } 00231 while(1) { 00232 uLCD.cls(); 00233 uLCD.locate(3,7); 00234 uLCD.printf("GAME OVER"); 00235 wait(1); 00236 } 00237 } 00238 void result(int outcome) 00239 { 00240 uLCD.cls(); 00241 if(outcome == 0) { 00242 00243 uLCD.locate(6,7); 00244 uLCD.printf("Player Won "); 00245 money += bet; 00246 } else if(outcome == 1) { 00247 uLCD.locate(6,7); 00248 uLCD.printf("Game Draw"); 00249 00250 } else if(outcome == 2) { 00251 uLCD.locate(6,7); 00252 uLCD.printf("Player Lost "); 00253 money -=bet; 00254 } 00255 wait(1); 00256 game(); 00257 } 00258 void dealerTurn() 00259 { 00260 isPlayer = false; 00261 uLCD.locate(4,3); 00262 uLCD.printf("%s%s",dealerHand[1].convertRank(),dealerHand[1].convertSuit()); 00263 uLCD.locate(6,6.5); 00264 dealerPoints = sumHand(dealerHand); 00265 printPoints(dealerPoints); 00266 wait(1); 00267 while(dealerPoints<17) { 00268 hit(); 00269 } 00270 if(dealerPoints>21) { 00271 result(0); 00272 } else if(playerPoints>dealerPoints) { 00273 result(0); 00274 } else if(playerPoints<dealerPoints) { 00275 result(2); 00276 } else { 00277 result(1); 00278 } 00279 } 00280 00281 00282 int sumHand(Card hand[]) 00283 { 00284 int sum=0; 00285 int aces=0; 00286 int count= isPlayer? playerCount:dealerCount; 00287 for(int i=0; i<count; i++) { 00288 Card card = hand[i]; 00289 if(card._rank==1) { 00290 sum+=11; 00291 aces++; 00292 } else if(card._rank < 11) { 00293 sum+=card._rank; 00294 } else { 00295 sum+=10; 00296 } 00297 } 00298 if(sum>21) { 00299 if(aces>0) { 00300 if(isPlayer) { 00301 while(aces>0 && sum > 21) { 00302 sum-=10; 00303 aces--; 00304 } 00305 } else { 00306 if(sum>=27) { 00307 while(aces>0 && sum > 21) { 00308 sum-=10; 00309 aces--; 00310 } 00311 } 00312 00313 } 00314 } 00315 } 00316 return sum; 00317 }
Generated on Sat Jul 16 2022 20:56:06 by
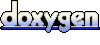