
Peer to peer xDot
Dependencies: ISL29011 libxDot-dev-mbed5-deprecated
main.cpp
00001 #include "mbed.h" 00002 #include "mDot.h" 00003 #include "rtos.h" 00004 #include "ChannelPlan.h" 00005 #include "ISL29011.h" 00006 #include "dot_util.h" 00007 #include "RadioEvent.h" 00008 00009 static uint8_t network_address[] = { 0x04, 0x05, 0x03, 0x04 }; 00010 static uint8_t network_session_key[] = { 0x04, 0x05, 0x03, 0x04, 0x04, 0x05, 0x03, 0x04, 0x04, 0x05, 0x03, 0x04, 0x04, 0x05, 0x03, 0x04 }; 00011 static uint8_t data_session_key[] = { 0x04, 0x05, 0x03, 0x04, 0x04, 0x05, 0x03, 0x04, 0x04, 0x05, 0x03, 0x04, 0x04, 0x05, 0x03, 0x04 }; 00012 00013 mDot* dot = NULL; 00014 lora::ChannelPlan* plan = NULL; 00015 00016 static Serial pc(USBTX, USBRX); 00017 00018 I2C i2c(I2C_SDA, I2C_SCL); 00019 ISL29011 lux(i2c); 00020 00021 int main() { 00022 00023 // Custom event handler for automatically displaying RX data 00024 RadioEvent events; 00025 uint32_t tx_frequency; 00026 uint8_t tx_datarate; 00027 uint8_t tx_power; 00028 00029 pc.baud(115200); 00030 00031 plan = new lora::ChannelPlan_US915(); 00032 dot = mDot::getInstance(plan); 00033 assert(dot); 00034 00035 logInfo("defaulting Dot configuration"); 00036 dot->resetConfig(); 00037 00038 // make sure library logging is turned on 00039 dot->setLogLevel(mts::MTSLog::INFO_LEVEL); 00040 00041 // attach the custom events handler 00042 dot->setEvents(&events); 00043 00044 // update configuration if necessary 00045 if (dot->getJoinMode() != mDot::PEER_TO_PEER) { 00046 logInfo("changing network join mode to PEER_TO_PEER"); 00047 if (dot->setJoinMode(mDot::PEER_TO_PEER) != mDot::MDOT_OK) { 00048 logError("failed to set network join mode to PEER_TO_PEER"); 00049 } 00050 } 00051 00052 tx_frequency = 915500000; 00053 tx_datarate = lora::DR_13; 00054 // 915 bands have no duty cycle restrictions, set tx power to max 00055 tx_power = 20; 00056 00057 // in PEER_TO_PEER mode there is no join request/response transaction 00058 // as long as both Dots are configured correctly, they should be able to communicate 00059 update_peer_to_peer_config(network_address, network_session_key, data_session_key, tx_frequency, tx_datarate, tx_power); 00060 00061 // save changes to configuration 00062 logInfo("saving configuration"); 00063 if (!dot->saveConfig()) { 00064 logError("failed to save configuration"); 00065 } 00066 00067 // display configuration 00068 display_config(); 00069 00070 lux.setMode(ISL29011::ALS_CONT); 00071 lux.setResolution(ISL29011::ADC_16BIT); 00072 lux.setRange(ISL29011::RNG_64000); 00073 00074 while (true) { 00075 uint16_t light; 00076 std::vector<uint8_t> tx_data; 00077 00078 // join network if not joined 00079 if (!dot->getNetworkJoinStatus()) { 00080 join_network(); 00081 } 00082 00083 light = lux.getData(); 00084 tx_data.push_back((light >> 8) & 0xFF); 00085 tx_data.push_back(light & 0xFF); 00086 logInfo("light: %lu [0x%04X]", light, light); 00087 send_data(tx_data); 00088 00089 // the Dot can't sleep in PEER_TO_PEER mode 00090 // it must be waiting for data from the other Dot 00091 // send data every 5 seconds 00092 logInfo("waiting for 5s"); 00093 wait(5); 00094 } 00095 return 0; 00096 }
Generated on Tue Jul 12 2022 21:13:14 by
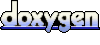