
Peer to peer xDot
Dependencies: ISL29011 libxDot-dev-mbed5-deprecated
dot_util.cpp
00001 #include "dot_util.h" 00002 #if defined(TARGET_XDOT_L151CC) 00003 #include "xdot_low_power.h" 00004 #endif 00005 00006 void display_config() { 00007 // display configuration and library version information 00008 logInfo("====================="); 00009 logInfo("general configuration"); 00010 logInfo("====================="); 00011 logInfo("version ------------------ %s", dot->getId().c_str()); 00012 logInfo("device ID/EUI ------------ %s", mts::Text::bin2hexString(dot->getDeviceId()).c_str()); 00013 logInfo("default channel plan ----- %s", mDot::FrequencyBandStr(dot->getDefaultFrequencyBand()).c_str()); 00014 logInfo("current channel plan ----- %s", mDot::FrequencyBandStr(dot->getFrequencyBand()).c_str()); 00015 if (lora::ChannelPlan::IsPlanFixed(dot->getFrequencyBand())) { 00016 logInfo("frequency sub band ------- %u", dot->getFrequencySubBand()); 00017 } 00018 logInfo("public network ----------- %s", dot->getPublicNetwork() ? "on" : "off"); 00019 logInfo("========================="); 00020 logInfo("credentials configuration"); 00021 logInfo("========================="); 00022 logInfo("device class ------------- %s", dot->getClass().c_str()); 00023 logInfo("network join mode -------- %s", mDot::JoinModeStr(dot->getJoinMode()).c_str()); 00024 if (dot->getJoinMode() == mDot::MANUAL || dot->getJoinMode() == mDot::PEER_TO_PEER) { 00025 logInfo("network address ---------- %s", mts::Text::bin2hexString(dot->getNetworkAddress()).c_str()); 00026 logInfo("network session key------- %s", mts::Text::bin2hexString(dot->getNetworkSessionKey()).c_str()); 00027 logInfo("data session key---------- %s", mts::Text::bin2hexString(dot->getDataSessionKey()).c_str()); 00028 } else { 00029 logInfo("network name ------------- %s", dot->getNetworkName().c_str()); 00030 logInfo("network phrase ----------- %s", dot->getNetworkPassphrase().c_str()); 00031 logInfo("network EUI -------------- %s", mts::Text::bin2hexString(dot->getNetworkId()).c_str()); 00032 logInfo("network KEY -------------- %s", mts::Text::bin2hexString(dot->getNetworkKey()).c_str()); 00033 } 00034 logInfo("========================"); 00035 logInfo("communication parameters"); 00036 logInfo("========================"); 00037 if (dot->getJoinMode() == mDot::PEER_TO_PEER) { 00038 logInfo("TX frequency ------------- %lu", dot->getTxFrequency()); 00039 } else { 00040 logInfo("acks --------------------- %s, %u attempts", dot->getAck() > 0 ? "on" : "off", dot->getAck()); 00041 } 00042 logInfo("TX datarate -------------- %s", mDot::DataRateStr(dot->getTxDataRate()).c_str()); 00043 logInfo("TX power ----------------- %lu dBm", dot->getTxPower()); 00044 logInfo("antenna gain ------------- %u dBm", dot->getAntennaGain()); 00045 logInfo("LBT ---------------------- %s", dot->getLbtTimeUs() ? "on" : "off"); 00046 if (dot->getLbtTimeUs()) { 00047 logInfo("LBT time ----------------- %lu us", dot->getLbtTimeUs()); 00048 logInfo("LBT threshold ------------ %d dBm", dot->getLbtThreshold()); 00049 } 00050 } 00051 00052 void update_peer_to_peer_config(uint8_t *network_address, uint8_t *network_session_key, uint8_t *data_session_key, uint32_t tx_frequency, uint8_t tx_datarate, uint8_t tx_power) { 00053 std::vector<uint8_t> current_network_address = dot->getNetworkAddress(); 00054 std::vector<uint8_t> current_network_session_key = dot->getNetworkSessionKey(); 00055 std::vector<uint8_t> current_data_session_key = dot->getDataSessionKey(); 00056 uint32_t current_tx_frequency = dot->getTxFrequency(); 00057 uint8_t current_tx_datarate = dot->getTxDataRate(); 00058 uint8_t current_tx_power = dot->getTxPower(); 00059 00060 std::vector<uint8_t> network_address_vector(network_address, network_address + 4); 00061 std::vector<uint8_t> network_session_key_vector(network_session_key, network_session_key + 16); 00062 std::vector<uint8_t> data_session_key_vector(data_session_key, data_session_key + 16); 00063 00064 if (current_network_address != network_address_vector) { 00065 logInfo("changing network address from \"%s\" to \"%s\"", mts::Text::bin2hexString(current_network_address).c_str(), mts::Text::bin2hexString(network_address_vector).c_str()); 00066 if (dot->setNetworkAddress(network_address_vector) != mDot::MDOT_OK) { 00067 logError("failed to set network address to \"%s\"", mts::Text::bin2hexString(network_address_vector).c_str()); 00068 } 00069 } 00070 00071 if (current_network_session_key != network_session_key_vector) { 00072 logInfo("changing network session key from \"%s\" to \"%s\"", mts::Text::bin2hexString(current_network_session_key).c_str(), mts::Text::bin2hexString(network_session_key_vector).c_str()); 00073 if (dot->setNetworkSessionKey(network_session_key_vector) != mDot::MDOT_OK) { 00074 logError("failed to set network session key to \"%s\"", mts::Text::bin2hexString(network_session_key_vector).c_str()); 00075 } 00076 } 00077 00078 if (current_data_session_key != data_session_key_vector) { 00079 logInfo("changing data session key from \"%s\" to \"%s\"", mts::Text::bin2hexString(current_data_session_key).c_str(), mts::Text::bin2hexString(data_session_key_vector).c_str()); 00080 if (dot->setDataSessionKey(data_session_key_vector) != mDot::MDOT_OK) { 00081 logError("failed to set data session key to \"%s\"", mts::Text::bin2hexString(data_session_key_vector).c_str()); 00082 } 00083 } 00084 00085 if (current_tx_frequency != tx_frequency) { 00086 logInfo("changing TX frequency from %lu to %lu", current_tx_frequency, tx_frequency); 00087 if (dot->setTxFrequency(tx_frequency) != mDot::MDOT_OK) { 00088 logError("failed to set TX frequency to %lu", tx_frequency); 00089 } 00090 } 00091 00092 if (current_tx_datarate != tx_datarate) { 00093 logInfo("changing TX datarate from %u to %u", current_tx_datarate, tx_datarate); 00094 if (dot->setTxDataRate(tx_datarate) != mDot::MDOT_OK) { 00095 logError("failed to set TX datarate to %u", tx_datarate); 00096 } 00097 } 00098 00099 if (current_tx_power != tx_power) { 00100 logInfo("changing TX power from %u to %u", current_tx_power, tx_power); 00101 if (dot->setTxPower(tx_power) != mDot::MDOT_OK) { 00102 logError("failed to set TX power to %u", tx_power); 00103 } 00104 } 00105 } 00106 00107 void join_network() { 00108 int32_t j_attempts = 0; 00109 int32_t ret = mDot::MDOT_ERROR; 00110 00111 // attempt to join the network 00112 while (ret != mDot::MDOT_OK) { 00113 logInfo("attempt %d to join network", ++j_attempts); 00114 ret = dot->joinNetwork(); 00115 if (ret != mDot::MDOT_OK) { 00116 logError("failed to join network %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00117 // in some frequency bands we need to wait until another channel is available before transmitting again 00118 uint32_t delay_s = (dot->getNextTxMs() / 1000) + 1; 00119 if (delay_s < 2) { 00120 logInfo("waiting %lu s until next free channel", delay_s); 00121 wait(delay_s); 00122 } else { 00123 logInfo("sleeping %lu s until next free channel", delay_s); 00124 dot->sleep(delay_s, mDot::RTC_ALARM, false); 00125 } 00126 } 00127 } 00128 } 00129 00130 void send_data(std::vector<uint8_t> data) { 00131 int32_t ret; 00132 00133 ret = dot->send(data); 00134 if (ret != mDot::MDOT_OK) { 00135 logError("failed to send data to %s [%d][%s]", dot->getJoinMode() == mDot::PEER_TO_PEER ? "peer" : "gateway", ret, mDot::getReturnCodeString(ret).c_str()); 00136 } else { 00137 logInfo("successfully sent data to %s", dot->getJoinMode() == mDot::PEER_TO_PEER ? "peer" : "gateway"); 00138 } 00139 } 00140
Generated on Tue Jul 12 2022 21:13:14 by
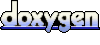