libEinkShield_mbedcli_ARM_NUCLEO_F446RE
Embed:
(wiki syntax)
Show/hide line numbers
EinkShield.h
00001 /* 00002 Copyright (c) 2017-2018, E Ink Holdings Inc., All Rights Reserved 00003 SPDX-License-Identifier: LicenseRef-PBL 00004 00005 This file and the related binary are licensed under the Permissive Binary 00006 License, Version 1.0 (the "License"); you may not use these files except in 00007 compliance with the License. 00008 00009 You may obtain a copy of the License here: 00010 LICENSE-permissive-binary-license-1.0.txt and at 00011 https://www.mbed.com/licenses/PBL-1.0 00012 00013 See the License for the specific language governing permissions and limitations 00014 under the License. 00015 */ 00016 /** 00017 * \mainpage EinkShield usage sample code 00018 * \code 00019 * #include "mbed.h" 00020 * #include "EinkShield.h" 00021 * #include "image3.h" 00022 * int main() { 00023 * EinkShield epd(EL029TR1, 00024 * D7, 00025 * D6, 00026 * D5, 00027 * D10, 00028 * D2, 00029 * D13, 00030 * D11); 00031 * epd.EPD_Init(); 00032 * epd.EPD_Display_Red(); 00033 * wait_ms(2000); 00034 * while(1) { 00035 * epd.EPD_Display_KWR(sale2_KW, sale2_R); 00036 * wait_ms(2000); 00037 * } 00038 * } 00039 * \endcode 00040 */ 00041 #ifndef EINK_SHIELD_H 00042 #define EINK_SHIELD_H 00043 00044 typedef enum { 00045 EL029TR1, 00046 } EPD_driver; 00047 00048 00049 /** @class EinkShield EinkShield.h */ 00050 /** class EinkShield for mbed-os */ 00051 class EinkShield { 00052 private: 00053 DigitalOut bsi ;//(D7); 00054 DigitalOut rstn ;//(D6); 00055 DigitalIn busyn;//(D5); 00056 DigitalOut csb ;//(D10); 00057 DigitalOut dc ;//(D2); 00058 DigitalOut scl ;//(D13); 00059 DigitalOut sda ;//(D11); 00060 00061 EPD_driver driver; 00062 00063 public: 00064 /** 00065 * Constructor to set pin assignment and driver 00066 * @param driver select different size display driver for EinkShield, for example: EL029TR1 00067 * @param bsi_pin bus selection pin 00068 * @param rstn_pin reset pin, L: driver will reset when low 00069 * @param busyn_pin busy pin, L: driver is busy 00070 * @param csb_pin chip-select pin 00071 * @param dc_pin data/command pin 00072 * @param scl_pin serial clock pin 00073 * @param sda_pin serial data pin 00074 * @return none 00075 */ 00076 EinkShield(EPD_driver driver, 00077 PinName bsi_pin, 00078 PinName rstn_pin, 00079 PinName busyn_pin, 00080 PinName csb_pin, 00081 PinName dc_pin, 00082 PinName scl_pin, 00083 PinName sda_pin); 00084 /** 00085 * Driver initial 00086 * @param none 00087 * @return none 00088 */ 00089 void EPD_Init(void); 00090 /** 00091 * Display image with color: black, white and red. 00092 * <pre> 00093 * Resolution of EL029TR1 is 128x296. 00094 * Pixel data alignment is from left to right and from top to bottom. 00095 * 00096 * <STRONG>img_kw</STRONG> point to black and white raw pixel data of image, 00097 * 1 bit per pixel, 0 = black, 1 = white, 00098 * Total size of img_kw is 128x296/8 = 4736 bytes for EL029TR1. 00099 * 00100 * <STRONG>img_r</STRONG> point to red raw pixel data of image, 00101 * 1 bit per pixel, 0 = red, 1 = reserved, 00102 * Total size of img_r is 128x296/8 = 4736 bytes for EL029TR1. 00103 * </pre> 00104 * 00105 * @param img_kw <pre>point to black and white raw pixel data of image, 00106 * 1 bit per pixel, 0 = black, 1 = white,</pre> 00107 * 00108 * @param img_r <pre>point to red raw pixel data of image, 00109 * 1 bit per pixel, 0 = red, 1 = reserved,</pre> 00110 * 00111 * 00112 * @return none 00113 */ 00114 void EPD_Display_KWR(unsigned char const *img_kw, unsigned char const *img_r); 00115 /** 00116 * Display full screen red 00117 * 00118 * @param none 00119 * @return none 00120 */ 00121 void EPD_Display_Red(void); 00122 }; 00123 00124 00125 00126 #endif
Generated on Tue Jul 12 2022 12:28:33 by
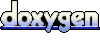