
RaheeNew
Fork of Adafruit9-DOf by
Embed:
(wiki syntax)
Show/hide line numbers
I2C_base.h
00001 #ifndef I2C_BASE_H 00002 #define I2C_BASE_H 00003 00004 #define _MBED_ 00005 00006 //Base class to extend I2C support to multiple platforms 00007 typedef char byte; 00008 00009 00010 class I2C_base 00011 { 00012 public: 00013 virtual void frequency (int frequency) = 0; //set the communication frequency 00014 virtual bool read (int address, byte* data, int length) = 0; //read data from the bus 00015 virtual bool write (int address, const byte* data, int length) = 0; //write data to the bus 00016 virtual bool writeByte (int address, byte data) = 0; //write byte to the bus 00017 00018 private: 00019 int _frequency; 00020 }; 00021 00022 extern I2C_base* i2c; 00023 00024 00025 //MBED programming environmnet 00026 #ifdef _MBED_ 00027 #include "mbed.h" 00028 //define SDA and SCL if not already defined 00029 #ifndef SDA 00030 #define SDA p28 00031 #endif 00032 00033 #ifndef SCL 00034 #define SCL p27 00035 #endif 00036 //******************************************* 00037 00038 00039 /* should be placed somewhere else, just debug code*/ 00040 00041 00042 00043 int millis(); 00044 00045 /***************************************************/ 00046 00047 class I2C_MBED: public I2C_base 00048 { 00049 public: 00050 I2C_MBED (PinName pSDA, PinName pSCL): _i2c(pSDA,pSCL){} 00051 virtual void frequency (int hz) 00052 { 00053 _i2c.frequency(hz); 00054 } 00055 virtual bool read(int address, byte* data, int length) 00056 { 00057 return !_i2c.read(address<<1,data,length); 00058 } 00059 virtual bool write(int address, const byte* data, int length) 00060 { 00061 return !_i2c.write(address<<1,data,length); 00062 } 00063 virtual bool writeByte(int address, byte data) 00064 { 00065 return write(address, &data, 1); 00066 } 00067 00068 private: 00069 I2C _i2c; 00070 }; 00071 00072 00073 00074 #endif //_MBED_ 00075 00076 00077 #endif //I2C_BASE_H
Generated on Wed Jul 13 2022 18:41:10 by
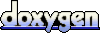