
RaheeNew
Fork of Adafruit9-DOf by
Embed:
(wiki syntax)
Show/hide line numbers
Adafruit_9DOF.h
00001 /*************************************************************************** 00002 This is a library for the Adafruit 9DOF Breakout 00003 00004 Designed specifically to work with the Adafruit 9DOF Breakout: 00005 http://www.adafruit.com/products/1714 00006 00007 These displays use I2C to communicate, 2 pins are required to interface. 00008 00009 Adafruit invests time and resources providing this open source code, 00010 please support Adafruit andopen-source hardware by purchasing products 00011 from Adafruit! 00012 00013 Written by Kevin Townsend for Adafruit Industries. 00014 BSD license, all text above must be included in any redistribution 00015 ***************************************************************************/ 00016 #ifndef __ADAFRUIT_9DOF_H__ 00017 #define __ADAFRUIT_9DOF_H__ 00018 00019 #include <Adafruit_Sensor.h> 00020 #include <Adafruit_LSM303_U.h> 00021 #include <Adafruit_L3GD20_U.h> 00022 00023 00024 /** Sensor axis */ 00025 typedef enum 00026 { 00027 SENSOR_AXIS_X = (1), 00028 SENSOR_AXIS_Y = (2), 00029 SENSOR_AXIS_Z = (3) 00030 } sensors_axis_t; 00031 00032 /* Driver for the the 9DOF breakout sensors */ 00033 class Adafruit_9DOF 00034 { 00035 public: 00036 Adafruit_9DOF(void); 00037 bool begin(void); 00038 00039 bool accelGetOrientation ( sensors_event_t *event, sensors_vec_t *orientation ); 00040 bool magTiltCompensation ( sensors_axis_t axis, sensors_event_t *mag_event, sensors_event_t *accel_event ); 00041 bool magGetOrientation ( sensors_axis_t axis, sensors_event_t *event, sensors_vec_t *mag_orientation ); 00042 bool fusionGetOrientation ( sensors_event_t *accel_event, sensors_event_t *mag_event, sensors_vec_t *orientation ); 00043 00044 private: 00045 }; 00046 00047 #endif
Generated on Wed Jul 13 2022 18:41:10 by
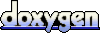