
Using personalization mode for the Multitech mDot. Works out of the box with f.e. LORIOT (just copy in DevAddr and the Keys).
Dependencies: libmDot mbed-rtos mbed
Fork of mDot_LoRa_Sleep_Example by
main.cpp
00001 #include "mbed.h" 00002 #include "mDot.h" 00003 #include "MTSLog.h" 00004 #include <string> 00005 #include <vector> 00006 #include <algorithm> 00007 #include "mbed.h" 00008 00009 static const char LORIOT_DEV_ADDR[] = "AABBCCDD"; // Use the big endian version here 00010 static const char LORIOT_NWK_S_KEY[] = "E8A25EBD07F85800E08478A041FACBA7"; // NWKSKEY 00011 static const char LORIOT_APP_S_KEY[] = "BE8EF84E745D0AB14D4507B0BA600555"; // APPSKEY 00012 00013 static mDot* dot; 00014 00015 // This is where we keep state, every time button D6 is pressed we up it 00016 // also we up it every time we send a package (in case you don't have a button) 00017 static int8_t counter = 0; 00018 00019 // Make sure to set this var every time you handle an interrupt! 00020 // It's important so we know how we woke up... 00021 static bool from_interrupt = false; 00022 00023 static InterruptIn btn(PA_1); /* D6 */ 00024 00025 void parseKeys() { 00026 int32_t ret; 00027 00028 // parse devkey 00029 static uint8_t NwkSKey[16]; 00030 static int ni; 00031 for (ni = 0; ni < 16; ni++) 00032 { 00033 char *non_numeric_ptr; 00034 const char hex[] = { '0', 'x', LORIOT_NWK_S_KEY[ni * 2], LORIOT_NWK_S_KEY[ni * 2 + 1] }; 00035 NwkSKey[ni] = strtoul(hex, &non_numeric_ptr, 16); 00036 free(non_numeric_ptr); 00037 } 00038 const std::vector<uint8_t> loriot_nwk_skey (NwkSKey, NwkSKey + sizeof(NwkSKey) / sizeof(NwkSKey[0]) ); 00039 00040 // parse appkey 00041 static uint8_t ArtSKey[16]; 00042 static int ai; 00043 for (ai = 0; ai < 16; ai++) 00044 { 00045 char *non_numeric_ptr; 00046 const char hex[] = { '0', 'x', LORIOT_APP_S_KEY[ai * 2], LORIOT_APP_S_KEY[ai * 2 + 1] }; 00047 ArtSKey[ai] = strtoul(hex, &non_numeric_ptr, 16); 00048 free(non_numeric_ptr); 00049 } 00050 const std::vector<uint8_t> loriot_app_skey (ArtSKey, ArtSKey + sizeof(ArtSKey) / sizeof(ArtSKey[0]) ); 00051 00052 // parse dev addr 00053 static uint8_t DevAddr[4]; 00054 for (ai = 0; ai < 4; ai++) 00055 { 00056 char *non_numeric_ptr; 00057 const char hex[] = { '0', 'x', LORIOT_DEV_ADDR[ai * 2], LORIOT_DEV_ADDR[ai * 2 + 1] }; 00058 DevAddr[ai] = strtoul(hex, &non_numeric_ptr, 16); 00059 free(non_numeric_ptr); 00060 } 00061 const std::vector<uint8_t> loriot_dev_addr (DevAddr, DevAddr + sizeof(DevAddr) / sizeof(DevAddr[0]) ); 00062 00063 logInfo("setting network address"); 00064 if ((ret = dot->setNetworkAddress(loriot_dev_addr)) != mDot::MDOT_OK) { 00065 logError("failed to set network address %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00066 } 00067 00068 logInfo("setting network session key"); 00069 if ((ret = dot->setNetworkSessionKey(loriot_nwk_skey)) != mDot::MDOT_OK) { 00070 logError("failed to set network session key %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00071 } 00072 00073 logInfo("setting app session key"); 00074 if ((ret = dot->setDataSessionKey(loriot_app_skey)) != mDot::MDOT_OK) { 00075 logError("failed to set app session key %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00076 } 00077 } 00078 00079 static void rise() { 00080 counter++; 00081 from_interrupt = true; 00082 } 00083 00084 void send_data(void) { 00085 int32_t ret; 00086 00087 std::vector<uint8_t> data; 00088 data.push_back(++counter); 00089 00090 logInfo("sending data 0x%02x", counter); 00091 if ((ret = dot->send(data)) != mDot::MDOT_OK) { 00092 logError("failed to send %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00093 00094 uint32_t sleep_time = std::max((uint32_t)10000, (uint32_t)dot->getNextTxMs()) / 1000; 00095 logError("if no free channel, please wait %d seconds", sleep_time); 00096 } else { 00097 logInfo("successfully sent data to gateway"); 00098 } 00099 } 00100 00101 int main() { 00102 int32_t ret; 00103 printf("Entering main()\r\n"); 00104 00105 btn.rise(&rise); 00106 00107 // get a mDot handle 00108 dot = mDot::getInstance(); 00109 00110 dot->setLogLevel(mts::MTSLog::DEBUG_LEVEL); 00111 00112 // print library version information 00113 logInfo("version: %s", dot->getId().c_str()); 00114 00115 //******************************************* 00116 // configuration 00117 //******************************************* 00118 // reset to default config so we know what state we're in 00119 dot->resetConfig(); 00120 00121 logInfo("frequencyBand: %d", dot->getFrequencyBand()); 00122 00123 // set up the mDot with our network information: frequency sub band, network name, and network password 00124 // these can all be saved in NVM so they don't need to be set every time - see mDot::saveConfig() 00125 00126 // set join mode to MANUAL so the mDot doesn't have to rejoin after sleeping 00127 logInfo("setting join mode to MANUAL"); 00128 if ((ret = dot->setJoinMode(mDot::MANUAL)) != mDot::MDOT_OK) { 00129 logError("failed to set join mode %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00130 } 00131 00132 logInfo("setting public network"); 00133 if ((ret = dot->setPublicNetwork(true)) != mDot::MDOT_OK) { 00134 logError("failed to set public network %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00135 } 00136 00137 logInfo("setting tx power to 20"); 00138 if ((ret = dot->setTxPower(20)) != mDot::MDOT_OK) { 00139 logError("failed to set tx power %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00140 } 00141 00142 // set up the network keys 00143 parseKeys(); 00144 00145 // a higher spreading factor allows for longer range but lower throughput 00146 // in the 915 (US) frequency band, spreading factors 7 - 10 are available 00147 // in the 868 (EU) frequency band, spreading factors 7 - 12 are available 00148 logInfo("setting TX spreading factor"); 00149 if ((ret = dot->setTxDataRate(mDot::SF_7)) != mDot::MDOT_OK) { 00150 logError("failed to set TX datarate %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00151 } 00152 00153 // request receive confirmation of packets from the gateway 00154 logInfo("disabling ACKs"); 00155 if ((ret = dot->setAck(0)) != mDot::MDOT_OK) { 00156 logError("failed to disable ACKs %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00157 } 00158 00159 logInfo("enabling ADR"); 00160 if ((ret = dot->setAdr(0)) != mDot::MDOT_OK) { 00161 logError("failed to enable ADR %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00162 } 00163 00164 // save this configuration to the mDot's NVM 00165 logInfo("saving config"); 00166 if (! dot->saveConfig()) { 00167 logError("failed to save configuration"); 00168 } 00169 //******************************************* 00170 // end of configuration 00171 //******************************************* 00172 00173 while (true) { 00174 // when wake from interrupt (need to set this yourself), we don't send 00175 // as duty cycle was not adhere'd yet... 00176 if (!from_interrupt) { 00177 send_data(); 00178 } 00179 from_interrupt = false; 00180 00181 // get the next transmission frame (in whole seconds) 00182 uint32_t sleep_time = ceil(static_cast<float>(dot->getNextTxMs()) / 1000.0f); 00183 logInfo("going to sleep for %d seconds", sleep_time); 00184 00185 // go to deepsleep and wake up automatically sleep_time seconds later 00186 // third argument (false) means SLEEP instead of DEEP_SLEEP mode, 00187 // keeps registers & allows wake on Interrupts 00188 dot->sleep(sleep_time, mDot::RTC_ALARM, false); 00189 } 00190 00191 // also an idea: go to deepsleep and wake up on rising edge of WKUP pin (PA0/XBEE_CTS/XBEE_DIO7) 00192 // dot->sleep(0, mDot::INTERRUPT, true); 00193 }
Generated on Mon Jul 25 2022 04:24:50 by
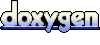