
jamie
Fork of PGO6_VoteController_template by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #define APP_VERSION 0.6f 00002 #define MQTT_VERSION 3 00003 #define BROKER_NAME "broker.hivemq.com" 00004 #define BROKER_PORT 1883 00005 00006 #include "debounce_button.h" 00007 #include "EthernetInterface.h" 00008 #include "MQTTNetwork.h" 00009 #include "MQTTmbed.h" 00010 #include "MQTTClient.h" 00011 00012 char* topic; 00013 00014 /** 00015 TODO 00016 ---- 00017 - Check if the button has been pressed. If so, print the amount of clicks to a serial terminal. 00018 - Make an MQTT-service which: 00019 - starts up a network using EthernetInterface. Make sure the development board requests its address via DHCP. 00020 - makes a client and connects it to the broker using a client ID and credentials (username & password). 00021 - sends messages at the same topic as the smartphone app from PGO 2. Feel free to choose which Quality of Service 00022 you are going to use. Make a separate function which handles the sending procedure. Therefore, this function 00023 can be called each time we want to send a certain message. 00024 - When the button is pressed once, we send an upvote. When pressed twice, a downvote is sent. By pressing 4 times, 00025 the program disconnects from the broker and terminates. 00026 00027 Extra 00028 ----- 00029 - Subscribe to the topic on which the song data is published. Display this received message on the serial terminal. 00030 - Test this controller in the complete system of PGO 2. Use these controllers instead of the smartphones. 00031 00032 Tips & tricks 00033 ------------- 00034 - To generate an interrupt on the press of a button, use: 00035 InterruptIn button(USER_BUTTON); 00036 ... 00037 button.fall(callback(someFunction)); 00038 - Before implementing MQTT, test the multiclick feature first. 00039 - Have a look at the MQTT-library for Mbed and the HelloMQTT-example. 00040 - To have a uniform message sending procedure, use the following function usage: 00041 sendMessage(&client, topic, buf, qos, retained, duplicate) 00042 */ 00043 int main(int argc, char* argv[]) 00044 { 00045 DigitalOut led1(LED1); 00046 for(int i=0;i<5;i++){ 00047 led1 = 1; 00048 wait(0.5); 00049 led1 = 0; 00050 wait(0.5); 00051 } 00052 00053 InterruptIn button(USER_BUTTON); 00054 while(1){ 00055 button.fall(callback(button1_onpressed_cb)); 00056 } 00057 return 0; 00058 } 00059 00060 00061
Generated on Wed Jul 13 2022 07:35:51 by
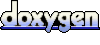