
jamie
Fork of PGO6_VoteController_template by
Embed:
(wiki syntax)
Show/hide line numbers
debounce_button.cpp
00001 #include "debounce_button.h" 00002 00003 DigitalIn button(USER_BUTTON); 00004 DigitalOut led1(LED1); 00005 DigitalOut led2(LED2); 00006 volatile bool button1_enabled = true; 00007 volatile bool button1_buzy = false; 00008 Timeout someTimeout; 00009 Timeout timer; 00010 int counter = 0; 00011 /** 00012 Some tips and tricks: 00013 - To use the built-in LED: 00014 DigitalOut led1(LED1); 00015 ... 00016 led1 = 1; 00017 - To delay the call of a function: 00018 Timeout someTimeout; 00019 ... 00020 someTimeout.attach(callback(&someFunction), 0.5) with 0.5 as 500 milliseconds 00021 - The variables that are used in interrupt callbacks have to be volatile, 00022 because these variables can change at any time. Therefore, the compiler is not 00023 going to make optimisations. 00024 */ 00025 00026 /** 00027 TODO 00028 ---- 00029 This function: 00030 - stores the amount of clicks in a variable which is read by the main loop. 00031 - resets the click counter which is used inside this file. 00032 - lowers a flag which tells the main loop that the user stopped pressing the button 00033 such that it can proceed its program. 00034 - turns the built-in LED off. Therefore, the user gets informed that the program stopped counting the clicks. 00035 */ 00036 00037 void replay(){ 00038 //replay the multiclick 00039 for(int i=0;i<counter;i++){ 00040 led2 = 1; 00041 wait(0.2); 00042 led2 =0; 00043 wait(0.2); 00044 } 00045 } 00046 00047 // This function enables the button again, such that unwanted clicks of the bouncing button get ignored. 00048 void button1_enabled_cb(void) 00049 { 00050 button1_enabled = true; 00051 led1 = 0; 00052 replay(); 00053 } 00054 00055 /** 00056 TODO 00057 ---- 00058 This function: 00059 - turns the built-in LED on, so the user gets informed that the program has started with counting clicks 00060 - disables the button such that the debouncer is active 00061 - enables the button again after a certain amount of time 00062 (use interrupts with "button1_enabled_cb()" as callback. 00063 - counts the amount of clicks within a period of 1 second 00064 - informs the main loop that the button has been pressed 00065 - informs the main loop that the user is clicking the button. 00066 Therefore, this main loop cannot continue its procedure until the clicks within 1 second have been counted. 00067 */ 00068 00069 void button1_busy_enable(){ 00070 button1_buzy = false; 00071 } 00072 void button1_onpressed_cb(void) 00073 { 00074 //button is pressed first time, turn led on 00075 if(button1_enabled){ 00076 counter = 0; 00077 // turns the built-in LED on, so the user gets informed that the program has started with counting clicks 00078 led1 = 1; 00079 //disables the button such that the debouncer is active 00080 button1_enabled = false; 00081 button1_buzy = true; 00082 timer.attach(callback(&button1_busy_enable),0.1); 00083 // enables the button again after a certain amount of time (use interrupts with "button1_enabled_cb()" as callback. 00084 someTimeout.attach(callback(&button1_enabled_cb), 5); 00085 }else if(!button1_buzy){ 00086 counter++; 00087 button1_buzy = true; 00088 timer.attach(callback(&button1_busy_enable),0.1); 00089 } 00090 00091 }
Generated on Wed Jul 13 2022 07:35:51 by
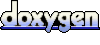