
FINAL VERSION
Embed:
(wiki syntax)
Show/hide line numbers
tests.h
00001 #ifndef TESTS_H 00002 #define TESTS_H 00003 00004 #include "Ball-test.h" 00005 #include "Brick-test.h" 00006 #include "Laser-test.h" 00007 00008 /** 00009 * @brief Run unit tests for relevant objects in the Breakout game 00010 * 00011 * @returns The number of failed tests 00012 */ 00013 int run_all_tests() 00014 { 00015 int n_tests_failed = 0; // initialised at 0 00016 00017 // Run the Ball_test_movement test 00018 printf("Testing Ball_test_movement...\n"); 00019 bool test_passed1 = Ball_test_movement(); 00020 00021 // Print out the result of this test 00022 if (test_passed1) { 00023 printf("...Passed!\n"); 00024 } 00025 else { 00026 printf("...FAILED!\n"); 00027 ++n_tests_failed; // Increment number of failures 00028 } 00029 00030 // Run the Ball_test_movement test 00031 printf("Testing Brick_test_movement...\n"); 00032 bool test_passed2 = Brick_test_movement(); 00033 00034 // Print out the result of this test 00035 if (test_passed2) { 00036 printf("...Passed!\n"); 00037 } 00038 else { 00039 printf("...FAILED!\n"); 00040 ++n_tests_failed; // Increment number of failures 00041 } 00042 00043 // Run the Ball_test_movement test 00044 printf("Testing Laser_test_movement...\n"); 00045 bool test_passed3 = Laser_test_movement(); 00046 00047 // Print out the result of this test 00048 if (test_passed3) { 00049 printf("...Passed!\n"); 00050 } 00051 else { 00052 printf("...FAILED!\n"); 00053 ++n_tests_failed; // Increment number of failures 00054 } 00055 00056 // Finish by printing a summary of the tests 00057 if (n_tests_failed > 0) { 00058 printf("%d tests FAILED!\n", n_tests_failed); 00059 } 00060 else { 00061 printf("All tests passed!\n"); 00062 } 00063 00064 return n_tests_failed; 00065 } 00066 00067 #endif
Generated on Thu Jul 14 2022 01:22:29 by
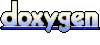