
FINAL VERSION
Embed:
(wiki syntax)
Show/hide line numbers
Laser.h
00001 #ifndef LASER_H 00002 #define LASER_H 00003 00004 #include "mbed.h" 00005 #include "N5110.h" 00006 #include "Gamepad.h" 00007 #include "Paddle.h" 00008 00009 /** Laser Class 00010 @author James Heavey, University of Leeds 00011 @brief Controls the Laser in the Breakout game 00012 @date May 2019 00013 */ 00014 class Laser 00015 { 00016 00017 public: 00018 00019 /** Constructor declaration */ 00020 Laser(); 00021 00022 /** Deconstructor declaration */ 00023 ~Laser(); 00024 00025 /** Initialises the laser off screen with a set velocity */ 00026 void init(); 00027 00028 /** Draws the Laser at at its current coordinates on the LCD 00029 * @param lcd @details a N5110 pointer 00030 */ 00031 void draw(N5110 &lcd); 00032 00033 /** Update the Laser's y coordinate based on its velocity */ 00034 void update(); 00035 00036 /** Sets the Laser's x coordinate 00037 * @param x @details set the variable _x to the new local x 00038 */ 00039 void set_posx(int x); 00040 00041 /** Sets the Laser's x coordinate 00042 * @param y @details set the variable _y to the new local y 00043 */ 00044 void set_posy(int y); 00045 00046 /** Retrieve the Laser's current x coordinate 00047 * @returns variable _x as an integer 00048 */ 00049 int get_x(); 00050 00051 /** Retrieve the Laser's current y coordinate 00052 * @returns variable _y as an integer 00053 */ 00054 int get_y(); 00055 00056 private: 00057 00058 int _speed_y; 00059 int _x; 00060 int _y; 00061 00062 }; 00063 #endif
Generated on Thu Jul 14 2022 01:22:29 by
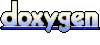