
FINAL VERSION
Embed:
(wiki syntax)
Show/hide line numbers
Brick.cpp
00001 #include "Brick.h" 00002 00003 Brick::Brick() 00004 { 00005 00006 } 00007 00008 Brick::~Brick() 00009 { 00010 00011 } 00012 00013 void Brick::init(int x,int y, int lives) // initialise the Brick object at the specified x, y coordinate and with the specified number of lives 00014 { 00015 _x = x; // x value on screen is fixed and must be defined at initialisation 00016 _y = y; // y value on screen is fixed and must be defined at initialisation 00017 _height = BRICK_HEIGHT; // set brick height and width defined in Brick.h 00018 _width = BRICK_WIDTH; 00019 _lives = lives; // lives set at initialisation, will be decremented upon collisions 00020 _base_lives = lives; // _base_lives variable used to reset the bricks to the number of lives specified at init 00021 00022 } 00023 00024 00025 void Brick::draw(N5110 &lcd) // draw the brick on the LCD 00026 { 00027 if (_x >= 0) { // only draw if on screen (more efficient?) 00028 if (_lives <= 2) { 00029 lcd.drawRect(_x,_y,_width,_height,FILL_TRANSPARENT); // if lives < 3 then draw a hollow brick 00030 } else if (_lives <= 4) { 00031 lcd.drawRect(_x,_y,_width,_height,FILL_BLACK); 00032 lcd.drawRect(_x + 3, _y + 1,_width-6,_height-2,FILL_WHITE); // if lives < 5 then fill up the brick a little bit (less hollow) 00033 } else { 00034 lcd.drawRect(_x,_y,_width,_height,FILL_BLACK); // if lives >= 5 then draw a fully black brick 00035 } 00036 } 00037 } 00038 00039 00040 bool Brick::hit() // decrement the number of lives by one (triggered by collisions), returns a bool 00041 { 00042 _lives = _lives - 1; // decrement lives 00043 if (_lives <= 0) { 00044 return true; // if lives <=0 brick is destroyed and will need to be moved off screen 00045 } else { 00046 return false; // else the brick is still alive and can remain where it is 00047 } 00048 } 00049 00050 00051 void Brick::reset_lives(int inc) // reset the lives after victory, takes inc 00052 { 00053 _lives = _base_lives + inc; // lives goes back to what it was at init plus 1 for every victory screen (inc = _multiplier in the breakout engine) 00054 } 00055 00056 int Brick::get_x() // return the brick's x coordinate (broken up into individual coordinates so it is easier to work with for list iterators) 00057 { 00058 return _x; 00059 } 00060 00061 00062 int Brick::get_y() // return the brick's y coordinate 00063 { 00064 return _y; 00065 } 00066 00067 00068 void Brick::set_posx(int x) // set the brick's x coordinate (used to move it off and on screen). y is unneccessary 00069 { 00070 _x = x; 00071 }
Generated on Thu Jul 14 2022 01:22:29 by
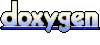