
FINAL VERSION
Embed:
(wiki syntax)
Show/hide line numbers
Ball.h
00001 #ifndef BALL_H 00002 #define BALL_H 00003 00004 #include "mbed.h" 00005 #include "N5110.h" 00006 #include "Gamepad.h" 00007 #include "Paddle.h" 00008 00009 #define BALL_SIZE 2 00010 #define BALL_SPEED 2 00011 00012 /** Ball Class 00013 @author James Heavey, University of Leeds 00014 @brief Controls the ball in the Breakout game 00015 @date May 2019 00016 */ 00017 00018 class Ball 00019 { 00020 00021 public: 00022 00023 /** Constructor declaration */ 00024 Ball(); 00025 00026 /** Destructor declaration */ 00027 ~Ball(); 00028 00029 /** Initialise Ball attributes 00030 * @param size @details initialises _size 00031 * @param speed @details initialises _speed 00032 * @param x @details initialises the _x coordinate over the centre of the paddle 00033 */ 00034 void init(int size,int speed,int x); 00035 00036 /** Draws the Ball at at its current coordinates on the LCD 00037 * @param lcd @details a N5110 pointer 00038 */ 00039 void draw(N5110 &lcd); 00040 00041 /** Update the Laser's x and y coordinates based on its velocity */ 00042 void update(); 00043 00044 /** Set the Ball's x and y velocities 00045 * @param v @details a vector of the x and y velocities 00046 */ 00047 void set_velocity(Vector2D v); 00048 00049 /** Set the Ball's x and y coordinates 00050 * @param p @details a vector of the x and y velocities 00051 */ 00052 void set_pos(Vector2D p); 00053 00054 /** Retrieves the Ball's x and y velocities 00055 * @returns a vector of the x and y velocities 00056 */ 00057 Vector2D get_velocity(); 00058 00059 /** Retrieves the Ball's x and y velocities 00060 * @returns a vector of the x and y velocities 00061 */ 00062 Vector2D get_pos(); 00063 00064 00065 00066 private: 00067 00068 Vector2D _velocity; 00069 int _size; 00070 int _x; 00071 int _y; 00072 }; 00073 #endif
Generated on Thu Jul 14 2022 01:22:29 by
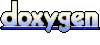