
FINAL VERSION
Embed:
(wiki syntax)
Show/hide line numbers
Ball.cpp
00001 #include "Ball.h" 00002 00003 Ball::Ball() 00004 { 00005 00006 } 00007 00008 Ball::~Ball() 00009 { 00010 00011 } 00012 00013 void Ball::init(int size,int speed, int x) 00014 { 00015 _size = size; // size of the ball 00016 00017 _x = x; // init position of the ball will be at the centre of the paddle 00018 _y = HEIGHT - _size/2 - 3; // fixed y init position, just above the paddle 00019 00020 srand(time(NULL)); 00021 int direction = rand() % 2; // randomise initial direction. 00022 00023 // 2 directions, both directed upwards (N) from the paddle 00024 if (direction == 0) { 00025 _velocity.x = speed; // direction = E 00026 _velocity.y = -speed; // direction = N 00027 } else { 00028 _velocity.x = -speed; // direction = W 00029 _velocity.y = -speed; // direction = N 00030 } 00031 } 00032 00033 00034 void Ball::draw(N5110 &lcd) // draw the ball at the specified location 00035 { 00036 lcd.drawRect(_x,_y,_size,_size,FILL_BLACK); 00037 } 00038 00039 00040 void Ball::update() // update the ball's position, based on its current velocity 00041 { 00042 _x += _velocity.x; 00043 _y += _velocity.y; 00044 } 00045 00046 00047 void Ball::set_velocity(Vector2D v) // set the velocity in its x/y directions (used for collision velocity correction) 00048 { 00049 _velocity.x = v.x; 00050 _velocity.y = v.y; 00051 } 00052 00053 00054 Vector2D Ball::get_velocity() // retrieve the ball's current velocities 00055 { 00056 Vector2D v = {_velocity.x,_velocity.y}; 00057 return v; 00058 } 00059 00060 00061 Vector2D Ball::get_pos() // retrieve the ball's current coordinates 00062 { 00063 Vector2D p = {_x,_y}; 00064 return p; 00065 } 00066 00067 00068 void Ball::set_pos(Vector2D p) // set the ball's coordinates (used for collision velocity correction) 00069 { 00070 _x = p.x; 00071 _y = p.y; 00072 }
Generated on Thu Jul 14 2022 01:22:29 by
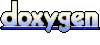