
FINAL VERSION
Embed:
(wiki syntax)
Show/hide line numbers
Ball-test.h
00001 #ifndef BALL_TEST_H 00002 #define BALL_TEST_H 00003 00004 /** 00005 * \brief Check that Ball object goes to correct position when moved 00006 * 00007 * \returns true if all the tests passed 00008 */ 00009 bool Ball_test_movement() 00010 { 00011 // Initialise Ball object with a size of 2, and speed of 2 00012 Ball _ball; 00013 _ball.init(2, 2); 00014 00015 // Set the position to 0, 0 00016 Vector2D initial_pos = {0, 0}; 00017 _ball.set_pos(initial_pos); 00018 00019 // Read the position 00020 Vector2D read_pos_1 = _ball.get_pos(); 00021 printf("%f, %f\n", read_pos_1.x, read_pos_1.y); 00022 00023 // Set the velocity to -2, 3 00024 Vector2D velocity = {-2, 3}; 00025 _ball.set_velocity(velocity); 00026 00027 // Update the position 00028 _ball.update(); 00029 00030 // Read the position 00031 Vector2D read_pos_2 = _ball.get_pos(); 00032 printf("%f, %f\n", read_pos_2.x, read_pos_2.y); 00033 00034 // Now check that both the positions are as expected 00035 bool success_flag = true; 00036 00037 // Fail the test if the initial position is wrong 00038 if (read_pos_1.x != 0 || read_pos_1.y != 0) { 00039 success_flag = false; 00040 } 00041 00042 // Fail the test if the final position is wrong 00043 if (read_pos_2.x != -2 || read_pos_2.y != 3) { 00044 success_flag = false; 00045 } 00046 00047 return success_flag; 00048 } 00049 #endif
Generated on Thu Jul 14 2022 01:22:29 by
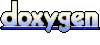