
IGGE Power board
Dependencies: mbed ADS1015 USBDevice MCP4725
main.cpp
00001 00002 #include "mbed.h" 00003 #include "Adafruit_ADS1015.h" 00004 //#include "USBSerial.h" 00005 00006 #include <iostream> 00007 #include <map> 00008 #include <string> 00009 #include <stdio.h> 00010 00011 #define SERIALOUT 00012 00013 //#define SERIAL_BAUD_RATE 9600 00014 00015 //library function setup 00016 I2C i2c(p28, p27); 00017 Adafruit_ADS1015 ads(&i2c); 00018 //USBSerial pc; // USB CDC serial port 00019 Ticker graph; 00020 00021 00022 //can stuff 00023 Ticker ticker; 00024 //CAN can1(p9, p10); 00025 CAN can2(p30, p29); 00026 char counter[8] = {1,1,1,1,1,1,1,1}; 00027 int test=0x0; 00028 char counter2=0; 00029 00030 00031 bool fromUsr[5] = {false,false,false,false,false}; //cpupwr, cpu rst, sensor, net, usrint 00032 00033 00034 00035 //leds 00036 DigitalOut led0(LED1); 00037 DigitalOut led1(LED2); 00038 DigitalOut led2(LED3); 00039 DigitalOut led3(LED4); 00040 00041 //current readings 00042 AnalogIn iBat(p15); //75a PROTO 00043 AnalogIn iTwelve(p17); //31a 00044 AnalogIn iFive(p18);//31a PROTO 00045 AnalogIn iMot(p16);//75a 00046 AnalogIn iCpu(p20);//31a 00047 AnalogIn iPeri(p19);//31a 00048 00049 //voltage scalers 00050 #define batScal 12.2 00051 #define motScal 12.2 00052 #define twelveScal 12.2 00053 #define fiveScal 12.2 00054 00055 //Relays 00056 DigitalOut relSens(p21); // PROTO 00057 DigitalOut relUsr(p24); 00058 DigitalOut relNet(p23); 00059 DigitalOut relCpuRst(p22); 00060 DigitalOut relCpuPower(p25); 00061 00062 00063 //30 minute graph arrays 00064 uint16_t powerArr [10]; //{iBat,vBat,iTwelve, vTwelve, iFive, vFive, iMot, vMot, iCpu, iPeri} 00065 uint16_t ibat,vbat,itwelve, vtwelve, ifive, vfive, imot, vmot, icpu, iperi= 0; 00066 00067 00068 00069 //volt 00070 uint16_t vBat, vTwelve,vFive,vMot ; 00071 00072 00073 00074 void sending_values(long int int1,long int int2,long int int3,long int int4, int ID ) 00075 { 00076 //val(); 00077 led1=1; 00078 counter[0]=int1 >> 8; 00079 counter[1]=int1 & 0xFF; 00080 counter[2]=int2 >> 8; 00081 counter[3]=int2 & 0xFF; 00082 counter[4]=int3 >> 8; 00083 counter[5]=int3 & 0xFF; 00084 counter[6]=int4 >> 8; 00085 counter[7]=int4 & 0xFF; 00086 led2=1; 00087 if(can2.write(CANMessage(ID, counter, 8))) { 00088 00089 // printf("Message sent: %x%x\r\n", counter[1],counter[0]); 00090 // printf("Should be: %d, %d, %d, %d\r\n", int1,int2,int3,int4);"); 00091 led1=0; 00092 } 00093 else 00094 printf("CAN ERROR"); 00095 00096 } 00097 00098 00099 00100 00101 00102 00103 void Can_main(long int val1,long int val2,long int val3,long int val4, int the_ID) 00104 { 00105 00106 std::map <int,string> CanIDMap; 00107 00108 CanIDMap[0]=" "; 00109 CanIDMap[1]="Message id 1: Batt Cur, Batt Volt, Twelve Cur, Twelve Volt"; 00110 CanIDMap[2]="Message id 2: Five Cur, Five Volt, Mot Cur, Mot Volt,"; 00111 CanIDMap[3]="Message id 3: Cpu Cur, Perifferal Cur, null, null...."; 00112 CanIDMap[4]="Message id 4: Network switch, usrint switch, sensor on switch, null"; 00113 CanIDMap[5]="Message id 5: cpupwr switch, cpu rst switch,null,null."; 00114 CanIDMap[6]=" "; 00115 CanIDMap[7]=" "; 00116 CanIDMap[8]=" "; 00117 CanIDMap[9]=" "; 00118 CanIDMap[10]=" "; 00119 CanIDMap[11]=" "; 00120 CanIDMap[12]="Zac is the Worst"; 00121 CanIDMap[13]="Auto"; 00122 CanIDMap[14]="Manual"; 00123 00124 00125 00126 00127 00128 //ticker.attach(&sending_values,1); 00129 CANMessage msg; 00130 int fixer1=0; 00131 int fixer2=0; 00132 int fixer3=0; 00133 int fixer4=0; 00134 00135 00136 if(can2.read(msg)) { 00137 bool tester= CanIDMap.find(msg.id) != CanIDMap.end(); 00138 if (tester) { 00139 //printf("Message received: %x %x \r\n", msg.data[0], msg.data[1]); 00140 00141 //printf("ID of message: %s \r\n", CanIDMap[msg.id]); 00142 00143 std::string str=CanIDMap[msg.id]; 00144 unsigned pos = str.find("_"); // position of "_" in str 00145 std::string str3 = str.substr (pos+1); // get from past"_" to the end 00146 // printf("Dealing with: %s \r\n",str3); 00147 fixer1=((msg.data[0]<<8) | (msg.data[1])); 00148 fixer2=((msg.data[2]<<8) | (msg.data[3])); 00149 fixer3=((msg.data[4]<<8) | (msg.data[5])); 00150 fixer4=((msg.data[6]<<8) | (msg.data[7])); 00151 if(msg.id==4) { // "Message id 4: Network switch, usrint switch, sensor on switch, null"; 00152 fromUsr[3]=fixer1; //fromUsr=cpupwr, cpu rst, sensor, net, usrint 00153 fromUsr[4]= fixer2; 00154 fromUsr[2]=fixer3; 00155 } 00156 00157 00158 else if(msg.id==5) { // "Message id 5: cpupwr switch, cpu rst switch,"; 00159 fromUsr[0]=fixer1; //fromUsr=cpupwr, cpu rst, sensor, net, usrint 00160 fromUsr[1]= fixer2; 00161 00162 00163 } 00164 00165 else{ 00166 led3=!led3; 00167 } 00168 led2 = !led2; 00169 00170 // printf("Resulting Answer back is: %d, %d, %d, %d\r\n\r\n\r\n",fixer1,fixer2,fixer3,fixer4 ); 00171 00172 } 00173 00174 else { 00175 00176 // printf("Can Message, not related to this controller\r\n\r\n"); 00177 00178 } 00179 00180 //wait(0.2); 00181 } 00182 00183 00184 00185 00186 00187 } 00188 00189 00190 00191 00192 void sendArray (void) 00193 { 00194 #ifdef SERIALOUT 00195 printf("___________________________\r\n"); 00196 printf("Sending messages....\r\n"); 00197 printf("Message id 1: Batt Cur = %d, Batt Volt = %d, Twelve Cur = %d, Twelve Volt = %d....",powerArr[0],powerArr[1],powerArr[2],powerArr[3]); 00198 sending_values(powerArr[0],powerArr[1],powerArr[2],powerArr[3],1); 00199 printf("....sent\r\n"); 00200 printf("Message id 2: Five Cur = %d, Five Volt = %d, Mot Cur = %d, Mot Volt = %d....",powerArr[4],powerArr[5],powerArr[6],powerArr[7]); 00201 sending_values(powerArr[4],powerArr[5],powerArr[6],powerArr[7],2); 00202 printf("....sent\r\n"); 00203 printf("Message id 3: Cpu Cur = %d, Perifferal Cur = %d, null, null....",powerArr[8],powerArr[9]); 00204 sending_values(powerArr[8],powerArr[9],0,0,3); 00205 printf("....sent\r\n"); 00206 printf("CANBUS TRANMISSION COMPLETE\r\n"); 00207 printf("~~~~~~~~~~~~~~~~~~~~~~~~~~~\r\n"); 00208 //relNet=!relNet; 00209 // relSens= !relSens; 00210 00211 00212 //relCpuPower=!relCpuPower; 00213 //relCpuRst =!relCpuRst; 00214 // relUsr=!relUsr; 00215 float neW =(powerArr[1]*.003); 00216 printf("actual vbat= %2.4f volt\r\n", neW); 00217 #endif 00218 #ifndef SERIALOUT 00219 sending_values(powerArr[0],powerArr[1],powerArr[2],powerArr[3],1); 00220 sending_values(powerArr[4],powerArr[5],powerArr[6],powerArr[7],2); 00221 sending_values(powerArr[8],powerArr[9],0,0,3); 00222 // relNet=!relNet; 00223 //relSens= !relSens; 00224 00225 //relCpuPower=!relCpuPower; 00226 //relCpuRst =!relCpuRst; 00227 // relUsr=!relUsr; 00228 #endif 00229 00230 } 00231 00232 00233 00234 void setup(void) 00235 { 00236 graph.attach(&sendArray, (1)); //update array every 10 seconds 00237 00238 can2.frequency(500000); 00239 //initialize relays 00240 relSens= 0; 00241 00242 relNet =0; 00243 relCpuPower=0; 00244 relCpuRst =0; 00245 relUsr=0; 00246 wait(3); 00247 sending_values(1,1,1,1,4); 00248 wait(.01); 00249 sending_values(0,0,0,0,4); 00250 00251 00252 00253 } 00254 00255 00256 00257 int main() 00258 { 00259 setup(); 00260 00261 00262 while (1) { 00263 Can_main(0,0,0,0,0); 00264 00265 00266 00267 powerArr[0]=ibat = (iBat.read_u16()); 00268 powerArr[1]=vBat = ads.readADC_SingleEnded(0); // read channel 0 PROTO 00269 00270 powerArr[2]=itwelve = (iTwelve.read_u16()); 00271 powerArr[3]=vtwelve = ads.readADC_SingleEnded(1); // read channel 1 00272 00273 powerArr[4]=ifive = (iFive.read_u16()); 00274 powerArr[5]=vfive = ads.readADC_SingleEnded(2); // read channel 0 00275 00276 powerArr[6]=imot = (iMot.read_u16()); 00277 powerArr[7]=vmot = ads.readADC_SingleEnded(3); // read channel 0 00278 00279 powerArr[8]=icpu = (iCpu.read_u16()); 00280 powerArr[9]=iperi = (iPeri.read_u16()); 00281 00282 //powerArr[] = {ibat,vbat,itwelve, vtwelve, ifive, vfive, imot, vmot, icpu, iperi}; 00283 00284 relCpuPower=fromUsr[0]; 00285 relCpuRst =fromUsr[1]; 00286 relSens= fromUsr[2]; 00287 relNet =fromUsr[3]; 00288 relUsr=fromUsr[4]; 00289 00290 00291 //cpupwr, cpu rst, sensor, net, usrint 00292 00293 led0=!led0; 00294 00295 } 00296 } 00297 00298 00299 00300 00301 00302 00303 00304 00305 00306 00307 00308 00309 00310 00311 00312 00313 00314 00315 00316
Generated on Sun Jul 24 2022 03:10:04 by
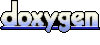