ADC Sensor LoRa Demo
Dependencies: libmDot mbed-rtos mbed-src
main.cpp
00001 /* 00002 * ADC Sensor Demo 00003 * 00004 * Analog inputs are read and pushed to the LoRa gateway. 00005 * 00006 * Analog sensors should be wired to mDot pins 16 and 17. 00007 * 00008 */ 00009 00010 #include "mbed.h" 00011 #include "mDot.h" 00012 #include <string> 00013 #include <vector> 00014 00015 /* 00016 * LoRa settings 00017 * 00018 * For Conduit AEP settings can be found at: 00019 * 00020 * https://<ip-address>/lora_network.html 00021 * 00022 * For Conduit mlinux: 00023 * 00024 * /var/config/lora/lora-network-server.conf 00025 * 00026 */ 00027 static std::string config_network_name = "testtest"; 00028 static std::string config_network_pass = "memememe"; 00029 static uint8_t config_frequency_sub_band = 5; 00030 00031 enum { 00032 MSG_TYPE_ADC0, 00033 MSG_TYPE_ADC1, 00034 }; 00035 00036 AnalogIn adc0(PA_1); // PIN16 on mDot 00037 AnalogIn adc1(PA_4); // PIN17 on mDot 00038 00039 Serial debug(USBTX, USBRX); 00040 00041 int main() { 00042 int32_t rc; 00043 00044 debug.baud(115200); 00045 00046 mDot *mdot = mDot::getInstance(); 00047 00048 mdot->resetConfig(); 00049 00050 printf("mDot version: %s\r\n", mdot->getId().c_str()); 00051 00052 // Activity Status on UDK2 00053 mdot->setActivityLedPin(LED1); 00054 mdot->setActivityLedEnable(true); 00055 00056 if ((rc = mdot->setFrequencySubBand(config_frequency_sub_band)) != mDot::MDOT_OK) { 00057 printf("failed to set frequency sub-band: %d\r\n", rc); 00058 return 1; 00059 } 00060 00061 if ((rc = mdot->setNetworkName(config_network_name)) != mDot::MDOT_OK) { 00062 printf("failed to set network name: %d\r\n", rc); 00063 return 1; 00064 } 00065 00066 if ((rc = mdot->setNetworkPassphrase(config_network_pass)) != mDot::MDOT_OK) { 00067 printf("failed to set network pass phrase: %d\r\n", rc); 00068 return 1; 00069 } 00070 00071 while ((rc = mdot->joinNetwork()) != mDot::MDOT_OK) { 00072 printf("failed to join network: %d\r\n", rc); 00073 if (mdot->getFrequencyBand() == mDot::FB_868){ 00074 rc = mdot->getNextTxMs(); 00075 } else { 00076 rc = 0; 00077 } 00078 00079 wait(1); 00080 } 00081 00082 while (true) { 00083 std::vector<uint8_t> lora_pl; 00084 00085 // Read analog inputs 00086 uint16_t adc0_value = adc0.read_u16(); 00087 uint16_t adc1_value = adc1.read_u16(); 00088 00089 // Pack ADC0 TLV 00090 lora_pl.clear(); 00091 lora_pl.push_back(MSG_TYPE_ADC0); 00092 lora_pl.push_back(2); 00093 lora_pl.push_back(((char *) &adc0_value)[1]); 00094 lora_pl.push_back(((char *) &adc0_value)[0]); 00095 00096 // Pack ADC1 TLV 00097 lora_pl.push_back(MSG_TYPE_ADC1); 00098 lora_pl.push_back(2); 00099 lora_pl.push_back(((char *) &adc1_value)[1]); 00100 lora_pl.push_back(((char *) &adc1_value)[0]); 00101 00102 // Send message 00103 while ((rc = mdot->send(lora_pl)) != mDot::MDOT_OK) { 00104 printf("failed to send message: %d\r\n", rc); 00105 wait(1); 00106 } 00107 00108 wait(5); 00109 } 00110 }
Generated on Sat Jul 23 2022 10:20:24 by
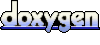