
Creating a CoxBox with the mbed
Dependencies: mbed C12832 SMARTGPU2
main.cpp
00001 #include "mbed.h" 00002 #include "C12832.h" 00003 #include "SMARTGPU2.h" 00004 #include "HallEffect.h" 00005 #include "TimerCB.h" 00006 00007 AnalogIn Ain(p17); 00008 AnalogOut Aout(p18); 00009 Ticker s20khz_tick; 00010 Ticker s1hz_tick; 00011 Ticker s30hz_tick; 00012 C12832 lcdX(p5, p7, p6, p8, p11); 00013 SMARTGPU2 lcd(TXPIN, RXPIN, RESETPIN); 00014 InterruptIn interrupt(p9); 00015 00016 //DigitalIn joyDown(p12); 00017 //DigitalIn joyRight(p16); 00018 InterruptIn startStop(p12); //joystick down 00019 InterruptIn reset(p16); //joystick right 00020 00021 void s20khz_task(void); 00022 void s1hz_task(void); 00023 void screenRefresh(void); 00024 void hallISR(void); 00025 void startStopTimer(void); 00026 void resetTimer(void); 00027 00028 float data_in, data_out; 00029 HallEffect hall; 00030 TimerCB timerCB; 00031 00032 //cache data for fixing LCD flicker 00033 int lastCount; 00034 int lastSPM; 00035 int lastMin; 00036 int lastSec; 00037 int lastMS; 00038 00039 void lcdInit() { 00040 lcd.reset(); 00041 lcd.start(); 00042 lcd.erase(); 00043 lastCount = -1; 00044 lastSPM = -1; 00045 lastMin = -1; 00046 lastSec = -1; 00047 lastMS = -1; 00048 } 00049 00050 void drawOut() { 00051 NUMBEROFBYTES bytes; 00052 //config strings 00053 lcd.setTextSize(FONT8); 00054 //high baud rate actually slows the system down 00055 // lcd.baudChange(BAUD7); //set a fast baud! for fast drawing 00056 00057 int spm = hall.getSPM(); 00058 if(spm != lastSPM){ 00059 lastSPM = spm; 00060 //draw black box over where rating is to clear it for redrawing 00061 lcd.drawRectangle(50,50,240,120,BLACK,FILL); 00062 //stroke rating using snprintf 00063 char spmBuffer[3]; 00064 snprintf(spmBuffer, 3, "%02d", spm); 00065 lcd.string(50,50,MAX_X_LANDSCAPE,MAX_Y_LANDSCAPE,spmBuffer,&bytes); 00066 } 00067 00068 int count = hall.getCount(); 00069 if(count != lastCount){ 00070 lastCount = count; 00071 //draw black box over where count is to clear it for redrawing 00072 lcd.drawRectangle(300,50,MAX_X_LANDSCAPE,120,BLACK,FILL); 00073 //stroke count using snprintf 00074 char strokesBuffer[4]; 00075 snprintf(strokesBuffer, 4, "%03d", count); 00076 lcd.string(300,50,MAX_X_LANDSCAPE,MAX_Y_LANDSCAPE,strokesBuffer,&bytes); 00077 } 00078 00079 /* 00080 TODO: make intelligent to only update (draw box): 00081 minutes if minutes changed 00082 seconds if sectonds changed 00083 ms if ms changed 00084 00085 Adapt to number of ones present in higher places 00086 */ 00087 00088 int tenthSec = timerCB.getMS()/100; 00089 if(tenthSec != lastMS){ 00090 lastMS = tenthSec; 00091 //draw black box over where the time is to clear it for redrawing 00092 lcd.drawRectangle(50,200,MAX_X_LANDSCAPE,MAX_Y_LANDSCAPE,BLACK,FILL); 00093 //time using snprintf 00094 char timeBuffer[8]; 00095 snprintf(timeBuffer, 8, "%02d:%02d.%d",timerCB.getMinutes(),timerCB.getSeconds(),tenthSec); 00096 lcd.string(50,200,MAX_X_LANDSCAPE,MAX_Y_LANDSCAPE,timeBuffer,&bytes); 00097 } 00098 00099 //print labels 00100 lcd.setTextSize(FONT4); 00101 lcd.string(50,170,MAX_X_LANDSCAPE,MAX_Y_LANDSCAPE,"Time",&bytes); 00102 lcd.string(300,20,MAX_X_LANDSCAPE,MAX_Y_LANDSCAPE,"Count",&bytes); 00103 lcd.string(50,20,MAX_X_LANDSCAPE,MAX_Y_LANDSCAPE,"SPM",&bytes); 00104 } 00105 00106 int main(){ 00107 // lcd.printf("Audio In"); 00108 //initialize big LCD 00109 lcdInit(); 00110 00111 s20khz_tick.attach_us(&s20khz_task,50); 00112 //refresh app board lcd at .5hz 00113 // s1hz_tick.attach(&s1hz_task,.5); 00114 //refresh screen at 30hz 00115 // s30hz_tick.attach(&screenRefresh,.5); 00116 00117 interrupt.rise(&hallISR); 00118 startStop.rise(&startStopTimer); 00119 reset.rise(&resetTimer); 00120 while(true){ 00121 drawOut(); 00122 } 00123 } 00124 00125 void s20khz_task(void) { 00126 data_in = Ain; 00127 data_out = data_in; 00128 Aout = data_out; 00129 } 00130 00131 void s1hz_task(void) { 00132 lcdX.cls(); 00133 lcdX.locate(0,0); 00134 lcdX.printf("StrokeCount: %d\r\nStroke Rating: %d\n", hall.getCount(), hall.getSPM()); 00135 lcdX.printf("time: %02d:%02d:%02d",timerCB.getMinutes(),timerCB.getSeconds(),(timerCB.getMS()/10)); 00136 } 00137 00138 void screenRefresh(void) { 00139 drawOut(); 00140 } 00141 00142 void hallISR() { 00143 hall.recordStroke(); 00144 if(!timerCB.isRunning()){ 00145 timerCB.start(); 00146 } 00147 } 00148 00149 void startStopTimer() { 00150 if(timerCB.isRunning()){ 00151 timerCB.stop(); 00152 } 00153 else { 00154 timerCB.start(); 00155 } 00156 } 00157 00158 void resetTimer() { 00159 timerCB.reset(); 00160 hall.resetCount(); 00161 }
Generated on Thu Jul 14 2022 01:14:36 by
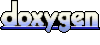