
Creating a CoxBox with the mbed
Dependencies: mbed C12832 SMARTGPU2
TimerCB.cpp
00001 #include "mbed.h" 00002 #include "C12832.h" 00003 #include "TimerCB.h" 00004 00005 TimerCB::TimerCB(){ 00006 running = false; 00007 } 00008 00009 int TimerCB::getMinutes(){ 00010 int secs = (int)timer.read(); 00011 int min = secs/60; 00012 return min; 00013 } 00014 00015 int TimerCB::getSeconds(){ 00016 int secs = (int)timer.read(); 00017 secs = secs%60; 00018 return secs; 00019 } 00020 00021 int TimerCB::getMS(){ 00022 int millis = timer.read_ms(); 00023 millis = millis%1000; 00024 return millis; 00025 } 00026 00027 void TimerCB::start(){ 00028 timer.start(); 00029 running = true; 00030 } 00031 00032 void TimerCB::stop(){ 00033 timer.stop(); 00034 running = false; 00035 } 00036 00037 void TimerCB::reset(){ 00038 timer.reset(); 00039 timer.stop(); 00040 running = false; 00041 } 00042 00043 bool TimerCB::isRunning(){ 00044 return running; 00045 }
Generated on Thu Jul 14 2022 01:14:36 by
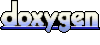