Library for handling ILI9163 - based LCD displays.
Embed:
(wiki syntax)
Show/hide line numbers
ili9163lcd.h
Go to the documentation of this file.
00001 /** 00002 * @file ili9163lcd.h 00003 * @brief ILI9163 128x128 LCD Driver (Header file) 00004 * 00005 * This code has been ported from the ili9163lcd library for mbed 00006 * made by Jun Morita. 00007 * Source form <http://files.noccylabs.info/lib430/liblcd/ili9163lcd_8c.html> 00008 * 00009 * This code has been ported from the ili9163lcd library for avr made 00010 * by Simon Inns, to run on a msp430. 00011 * 00012 * This program is free software: you can redistribute it and/or modify 00013 * it under the terms of the GNU General Public License as published by 00014 * the Free Software Foundation, either version 3 of the License, or 00015 * (at your option) any later version. 00016 * 00017 * This program is distributed in the hope that it will be useful, 00018 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 * GNU General Public License for more details. 00021 * 00022 * You should have received a copy of the GNU General Public License 00023 * along with this program. If not, see <http://www.gnu.org/licenses/>. 00024 * 00025 * @author Jun Morita (iccraft) 00026 * @author Simon Inns <simon.inns@gmail.com> 00027 * @author Christopher Vagnetoft (NoccyLabs) 00028 * @copyright (C) 2012 Simon Inns 00029 * @copyright parts (C) 2012 NoccyLabs 00030 */ 00031 00032 #ifndef ILI9163LCD_H_ 00033 #define ILI9163LCD_H_ 00034 00035 #include "mbed.h" 00036 #include "font5x8.h" 00037 00038 // Screen orientation defines: 00039 // 0 = Ribbon at top 00040 // 1 = Ribbon at left 00041 // 2 = Ribbon at right 00042 // 3 = Ribbon at bottom 00043 #define LCD_ORIENTATION0 0 00044 #define LCD_ORIENTATION1 96 00045 #define LCD_ORIENTATION2 160 00046 #define LCD_ORIENTATION3 192 00047 00048 // Each colour takes up 5 bits 00049 // Green is shifted 6 bits to the left 00050 // Blue is shifted 11 bits to the left 00051 // some RGB color definitions BLUE GREEN RED 00052 #define Black 0x0000 /* 0, 0, 0 */ 00053 #define Maroon 0x000F /* 0, 0,15 */ 00054 #define DarkGreen 0x03C0 /* 0,15, 0 */ 00055 #define Navy 0x7800 /* 15, 0, 0 */ 00056 #define Red 0x001F /* 0, 0,31 */ 00057 #define Green 0x07C0 /* 0,31, 0 */ 00058 #define Blue 0xF800 /* 31, 0, 0 */ 00059 #define Yellow 0x07DF /* 0,31,31 */ 00060 #define Magenta 0xF81F /* 31, 0,31 */ 00061 #define Cyan 0xFFC0 /* 31,31, 0 */ 00062 #define White 0xFFFF /* 31,31,31 */ 00063 00064 class ILI9163 { 00065 public: 00066 // Definitions for control lines (port C) 00067 static const uint8_t LCD_WR =(1 << 2); 00068 static const uint8_t LCD_RS =(1 << 4); 00069 static const uint8_t LCD_RD =(1 << 5); 00070 static const uint8_t LCD_CS =(1 << 6); 00071 static const uint8_t LCD_RESET =(1 << 7); 00072 // ILI9163 LCD Controller Commands 00073 static const uint8_t NOP = 0x00; 00074 static const uint8_t SOFT_RESET = 0x01; 00075 static const uint8_t GET_RED_CHANNEL = 0x06; 00076 static const uint8_t GET_GREEN_CHANNEL = 0x07; 00077 static const uint8_t GET_BLUE_CHANNEL = 0x08; 00078 static const uint8_t GET_PIXEL_FORMAT = 0x0C; 00079 static const uint8_t GET_POWER_MODE = 0x0A; 00080 static const uint8_t GET_ADDRESS_MODE = 0x0B; 00081 static const uint8_t GET_DISPLAY_MODE = 0x0D; 00082 static const uint8_t GET_SIGNAL_MODE = 0x0E; 00083 static const uint8_t GET_DIAGNOSTIC_RESULT = 0x0F; 00084 static const uint8_t ENTER_SLEEP_MODE = 0x10; 00085 static const uint8_t EXIT_SLEEP_MODE = 0x11; 00086 static const uint8_t ENTER_PARTIAL_MODE = 0x12; 00087 static const uint8_t ENTER_NORMAL_MODE = 0x13; 00088 static const uint8_t EXIT_INVERT_MODE = 0x20; 00089 static const uint8_t ENTER_INVERT_MODE = 0x21; 00090 static const uint8_t SET_GAMMA_CURVE = 0x26; 00091 static const uint8_t SET_DISPLAY_OFF = 0x28; 00092 static const uint8_t SET_DISPLAY_ON = 0x29; 00093 static const uint8_t SET_COLUMN_ADDRESS = 0x2A; 00094 static const uint8_t SET_PAGE_ADDRESS = 0x2B; 00095 static const uint8_t WRITE_MEMORY_START = 0x2C; 00096 static const uint8_t WRITE_LUT = 0x2D; 00097 static const uint8_t READ_MEMORY_START = 0x2E; 00098 static const uint8_t SET_PARTIAL_AREA = 0x30; 00099 static const uint8_t SET_SCROLL_AREA = 0x33; 00100 static const uint8_t SET_TEAR_OFF = 0x34; 00101 static const uint8_t SET_TEAR_ON = 0x35; 00102 static const uint8_t SET_ADDRESS_MODE = 0x36; 00103 static const uint8_t SET_SCROLL_START = 0x37; 00104 static const uint8_t EXIT_IDLE_MODE = 0x38; 00105 static const uint8_t ENTER_IDLE_MODE = 0x39; 00106 static const uint8_t SET_PIXEL_FORMAT = 0x3A; 00107 static const uint8_t WRITE_MEMORY_CONTINUE = 0x3C; 00108 static const uint8_t READ_MEMORY_CONTINUE = 0x3E; 00109 static const uint8_t SET_TEAR_SCANLINE = 0x44; 00110 static const uint8_t GET_SCANLINE = 0x45; 00111 static const uint8_t READ_ID1 = 0xDA; 00112 static const uint8_t READ_ID2 = 0xDB; 00113 static const uint8_t READ_ID3 = 0xDC; 00114 static const uint8_t FRAME_RATE_CONTROL1 = 0xB1; 00115 static const uint8_t FRAME_RATE_CONTROL2 = 0xB2; 00116 static const uint8_t FRAME_RATE_CONTROL3 = 0xB3; 00117 static const uint8_t DISPLAY_INVERSION = 0xB4; 00118 static const uint8_t SOURCE_DRIVER_DIRECTION = 0xB7; 00119 static const uint8_t GATE_DRIVER_DIRECTION = 0xB8; 00120 static const uint8_t POWER_CONTROL1 = 0xC0; 00121 static const uint8_t POWER_CONTROL2 = 0xC1; 00122 static const uint8_t POWER_CONTROL3 = 0xC2; 00123 static const uint8_t POWER_CONTROL4 = 0xC3; 00124 static const uint8_t POWER_CONTROL5 = 0xC4; 00125 static const uint8_t VCOM_CONTROL1 = 0xC5; 00126 static const uint8_t VCOM_CONTROL2 = 0xC6; 00127 static const uint8_t VCOM_OFFSET_CONTROL = 0xC7; 00128 static const uint8_t WRITE_ID4_VALUE = 0xD3; 00129 static const uint8_t NV_MEMORY_FUNCTION1 = 0xD7; 00130 static const uint8_t NV_MEMORY_FUNCTION2 = 0xDE; 00131 static const uint8_t POSITIVE_GAMMA_CORRECT = 0xE0; 00132 static const uint8_t NEGATIVE_GAMMA_CORRECT = 0xE1; 00133 static const uint8_t GAM_R_SEL = 0xF2; 00134 00135 // Create the ILI9163 object 00136 // 00137 // @param D13 SCK 00138 // @param D11 SDA 00139 // @param D10 A0 00140 // @param D9 RESET pin connected to RESET of display 00141 // @param D8 CS pin connected to CS of display 00142 // @param D12 LED (optional) connected to LED pin (for controlling backlight) 00143 // 00144 // ILI9163 lcd(D13,D11,D10,D9,D8,D12); 00145 // 00146 ILI9163(PinName SCK, PinName SDA, PinName A0, PinName RESET, PinName CS, PinName LED = NC); 00147 00148 SPI SPI_; 00149 DigitalOut A0_; 00150 DigitalOut RESET_; 00151 DigitalOut CS_; 00152 DigitalOut LED_; 00153 00154 // font array 00155 unsigned char* font; 00156 uint8_t font_bp_char; // Bytes per character 00157 uint8_t font_hor; // Horizontal size 00158 uint8_t font_vert; // Vertical size 00159 uint8_t font_bp_line; // Bytes per line 00160 00161 // LCD function prototypes 00162 void reset(void); 00163 void writeCommand(uint8_t address); 00164 void writeParameter(uint8_t parameter); 00165 void writeData(uint16_t data); 00166 void init(uint8_t orientation); 00167 00168 //Theoretically, it is possible to use PWM to change the display brightness 00169 //However, I found that it can interfere with data transfers, so I just use backlight on/off 00170 inline void backlightOn() { LED_ = 1; }; 00171 inline void backlightOff() { LED_ = 0; }; 00172 inline void backlightToggle() { LED_ = !LED_; }; 00173 00174 // Translates a 3 byte RGB value into a 2 byte value for the LCD (values should be 0-31) 00175 static inline uint16_t colourFromRGB5(uint8_t r, uint8_t g, uint8_t b) 00176 { return (b << 11) | (g << 6) | (r); } 00177 00178 static inline uint16_t colourFromRGB8(uint8_t r, uint8_t g, uint8_t b) 00179 { return colourFromRGB5(r >> 3, g >> 3, b >> 3); } 00180 00181 void clearDisplay(uint16_t colour); 00182 void plot(uint8_t x, uint8_t y, uint16_t colour); 00183 void line(int16_t x0, int16_t y0, int16_t x1, int16_t y1, uint16_t colour); 00184 void rectangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, uint16_t colour); 00185 void filledRectangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, uint16_t colour); 00186 void circle(int16_t xCentre, int16_t yCentre, int16_t radius, uint16_t colour); 00187 00188 // select the font to use 00189 // 00190 // @param f pointer to font array 00191 // 00192 // font array can be created with GLCD Font Creator from http://www.mikroe.com 00193 // you have to add 4 parameter at the beginning of the font array to use it: 00194 // - the number of bytes per char 00195 // - the vertical size in pixel 00196 // - the horizontal size in pixel 00197 // - the number of bytes per vertical line 00198 // you also have to change the array to char[] 00199 // 00200 void setFont(unsigned char* f); 00201 inline uint8_t lcdTextX(uint8_t x) { return x*font_hor; } 00202 inline uint8_t lcdTextY(uint8_t y) { return y*font_vert; } 00203 00204 void putCh(unsigned char character, uint8_t x, uint8_t y, uint16_t fgColour, uint16_t bgColour); 00205 void putS(const char *string, uint8_t x, uint8_t y, uint16_t fgColour, uint16_t bgColour); 00206 00207 }; // end class 00208 00209 #endif /* ILI9163LCD_H_ */
Generated on Fri Aug 12 2022 03:45:49 by
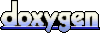