library for stepping motor
Embed:
(wiki syntax)
Show/hide line numbers
Suteppa.h
00001 #ifndef SUTEPPA_H 00002 #define SUTEPPA_H 00003 00004 #include "mbed.h" 00005 #ifndef M_PI 00006 #define M_PI 3.14159265359 00007 #endif 00008 00009 class Suteppa 00010 { 00011 public: 00012 Suteppa(); 00013 void init(unsigned long allStep, void (*stepper)(int)); 00014 void setSpeed(unsigned long speed); 00015 00016 void setDefaultSmooth(unsigned long step, unsigned long initSpeed); 00017 00018 void beginSmooth(unsigned long step, unsigned long initSpeed); 00019 void beginSmooth(); 00020 00021 void endSmooth(){_smooth = false;}; 00022 00023 long getStep(){return _step;}; 00024 long getStepAbsolute(){return _step%_allStep;}; 00025 00026 unsigned long getSpeed(){return _speed;}; 00027 00028 void rotate(int mode, long step, bool sync); 00029 void rotate(int mode, long step); 00030 00031 void setHome(); 00032 bool tick(); 00033 00034 static const int RELATIVE = 0; 00035 static const int ABSOLUTE = 1; 00036 static const int ABSOLUTE_SKIP = 2; 00037 private: 00038 void _delay(unsigned long time); 00039 00040 void _rotateAbsolute(long step, bool skip, bool sync); 00041 void _rotateRelative(long step, bool sync); 00042 00043 void (*_stepper)(int); 00044 void (*_turner)(int); 00045 00046 bool _smooth; 00047 00048 long _step; 00049 00050 unsigned long _allStep; 00051 unsigned long _speed; 00052 unsigned long _initDiff; 00053 unsigned long _smoothStep; 00054 unsigned long _initSpeed; 00055 00056 unsigned long _defaultSmoothStep; 00057 unsigned long _defaultInitSpeed; 00058 00059 int _r_direction; 00060 unsigned long _r_step; 00061 unsigned long _r_smoothStep; 00062 bool _r_smooth; 00063 float _r_max; 00064 unsigned int _r_i; 00065 unsigned long _r_time; 00066 unsigned long _r_interval; 00067 00068 float sigmoid(float x); 00069 Timer timer; 00070 }; 00071 00072 #endif
Generated on Sun Jul 17 2022 05:06:56 by
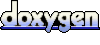