
demo of GPS receive using interrupt
Dependencies: GPS_INT MODSERIAL mbed
main.cpp
00001 // -*- coding: utf-8 -*- 00002 /** 00003 @file main.cpp 00004 @brief Sample for "GPS_INT" library 00005 00006 @author D.Nakayama 00007 @version 1.0 00008 @date 2018-07-12 D.Nakayama Written for C++/mbed. 00009 00010 00011 @see 00012 Copyright (C) 2018 D.Nakayama. 00013 Released under the MIT license. 00014 http://opensource.org/licenses/mit-license.php 00015 using device Nucleo-F401RE and GMS7-CR6 00016 */ 00017 00018 #include "mbed.h" 00019 #include "GPS_INT.h" 00020 #include "MODSERIAL.h" 00021 00022 MODSERIAL pc(USBTX, USBRX); // tx, rx 00023 GPS_INT gps(D8, D2); // tx, rx 00024 00025 int main() { 00026 printf("hello gps!\n"); 00027 while(1) { 00028 if(gps.location_is_update()){ 00029 printf("UTC :%04d/%02d/%02d %02d:%02d:%02d\n",gps.t.tm_year + 1900, gps.t.tm_mon + 1, gps.t.tm_mday, gps.t.tm_hour, gps.t.tm_min, gps.t.tm_sec); 00030 printf("longitude :%f\n",gps.lon); 00031 printf("latitude :%f\n",gps.lat); 00032 printf("PDOP :%.1f\n",gps.PDOP); 00033 printf("HDOP :%.1f\n",gps.HDOP); 00034 printf("VDOP :%.1f\n",gps.VDOP); 00035 printf("lock :%d\n",gps.lock); 00036 printf("n_sat :%d\n",gps.n_sat); 00037 printf("h_see :%.1f\n",gps.h_see); 00038 printf("h_geo :%.1f\n",gps.h_geo); 00039 printf("\n"); 00040 } 00041 } 00042 }
Generated on Sat Jul 23 2022 04:44:46 by
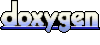