library to access typical i2c device
Embed:
(wiki syntax)
Show/hide line numbers
i2c_general_io.h
Go to the documentation of this file.
00001 // -*- coding: utf-8 -*- 00002 /** 00003 @file i2c_general_io.h 00004 @brief This is a library for accessing registers of a typical i2c sensor to set or read measurement value. 00005 00006 @author D.Nakayama 00007 @version 1.0 00008 @date 2018-07-08 D.Nakayama Written for C++/mbed. 00009 00010 00011 @see 00012 Copyright (C) 2018 D.Nakayama. 00013 Released under the MIT license. 00014 http://opensource.org/licenses/mit-license.php 00015 00016 */ 00017 00018 #ifndef INCLUDED_i2c_genela_io_h_ 00019 #define INCLUDED_i2c_genela_io_h_ 00020 #include "mbed.h" 00021 00022 /** 00023 @class GEN_I2C 00024 @brief Class for accessing a typical sensor with i2c 00025 */ 00026 class GEN_I2C{ 00027 00028 public: 00029 00030 /** 00031 @brief Create a new i2c sensor. 00032 @param sda SDA pin name (Defined in PinName.h) 00033 @param sck SCK pin name (Defined in PinName.h) 00034 */ 00035 GEN_I2C(PinName sda, PinName sck); 00036 /** 00037 @brief Create a new i2c sensor. 00038 @param &i2c_obj i2c name 00039 */ 00040 GEN_I2C(I2C &i2c_obj); 00041 00042 /** 00043 @brief Disable the i2c sensor. 00044 @param No parameters. 00045 */ 00046 virtual ~GEN_I2C(); 00047 00048 00049 //multi bytes func 00050 /** 00051 @brief multible read register. 00052 @param[in] Device_add Device i2c address(8bit) 00053 @param[in] reg_add register address(8bit) 00054 @param[out] *data read data array 00055 @param[in] n Number of register reads 00056 @return int read result(define mbed(i2c)) 00057 */ 00058 virtual int read_reg(char Device_add, char reg_add, char *data, int n); 00059 00060 //single byte func 00061 /** 00062 @brief single read register. 00063 @param[in] Device_add Device i2c address(8bit) 00064 @param[in] reg_add register address(8bit) 00065 @return char read data 00066 */ 00067 virtual char read_reg(char Device_add, char reg_add); 00068 00069 /** 00070 @brief single write register. 00071 @param[in] Device_add Device i2c address(8bit) 00072 @param[in] reg_add register address(8bit) 00073 @param[in] data write data 00074 @return int write result(define mbed(i2c)) 00075 */ 00076 virtual int write_reg(char Device_add, char reg_add, char data); 00077 00078 private: 00079 I2C *i2c_p; 00080 I2C &i2c; 00081 00082 }; 00083 00084 #endif
Generated on Fri Jul 29 2022 03:00:59 by
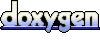