
Simple square wave generator using MAX32630FTHR and a potentiometer.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /****************************************************************************** 00002 * MIT License 00003 * 00004 * Copyright (c) 2017 Justin J. Jordan 00005 * 00006 * Permission is hereby granted, free of charge, to any person obtaining a copy 00007 * of this software and associated documentation files (the "Software"), to deal 00008 * in the Software without restriction, including without limitation the rights 00009 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00010 * copies of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 00013 * The above copyright notice and this permission notice shall be included in all 00014 * copies or substantial portions of the Software. 00015 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00017 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00018 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00019 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00020 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00022 * SOFTWARE. 00023 ******************************************************************************/ 00024 00025 00026 //Demo uses pot connected between 3.3 and GND with wiper on AIN_0. 00027 //SQWV is generated on P4_0 00028 00029 00030 #include "mbed.h" 00031 #include "max32630fthr.h" 00032 #include "adc.h" 00033 00034 00035 int main() 00036 { 00037 MAX32630FTHR pegasus(MAX32630FTHR::VIO_3V3); 00038 00039 MBED_ASSERT(ADC_Init() == E_NO_ERROR); 00040 00041 //Init pwm signal 00042 PwmOut pwmOut(P4_0); 00043 pwmOut.write(0.5F); 00044 00045 uint16_t rawADCdata; 00046 float volts = 0.0F; 00047 float period = 0.0F; 00048 00049 pwmOut.period(period); 00050 00051 printf("\033[H"); //home 00052 printf("\033[0J"); //erase from cursor to end of screen 00053 00054 //loop 00055 while(1) 00056 { 00057 //Do ADC conversion 00058 ADC_StartConvert(ADC_CH_0_DIV_5, 1, 1); 00059 ADC_GetData(&rawADCdata); 00060 00061 //Get ADC value in volts 00062 volts = ((6.0F * rawADCdata) / 1023.0F); 00063 00064 //convert to period in seconds for pwm 00065 period = (1.0F / ((300.0F * volts) + 10.0F)); 00066 00067 //Update sqwv period 00068 pwmOut.period(period); 00069 pwmOut.write(0.5F); 00070 00071 //Display data 00072 printf("Raw Data = 0x%04x\r\n", rawADCdata); 00073 printf("Volts = %3.2f\r\n", volts); 00074 printf("Period = %5.4f\r\n", period); 00075 printf("\033[H"); //home 00076 00077 wait(0.1F); 00078 } 00079 }
Generated on Wed Jul 20 2022 17:53:23 by
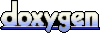