Fork for fixes
Embed:
(wiki syntax)
Show/hide line numbers
os2_stoip4.c
00001 /* 00002 * Copyright (c) 2014-2018 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "mbed_version.h" 00017 00018 #if MBED_MAJOR_VERSION == 2 00019 00020 #include <string.h> 00021 #include <stdlib.h> 00022 #include <stdint.h> 00023 #include "common_functions.h" 00024 #include "ip4string.h" 00025 00026 /** 00027 * Convert numeric IPv4 address string to a binary. 00028 * \param ip4addr IPv4 address in string format. 00029 * \param len Length of IPv4 string, maximum of 16.. 00030 * \param dest buffer for address. MUST be 4 bytes. 00031 * \return boolean set to true if conversion succeded, false if it didn't 00032 */ 00033 bool stoip4(const char *ip4addr, size_t len, void *dest) 00034 { 00035 uint8_t *addr = dest; 00036 00037 if (len > 16) { // Too long, not possible 00038 return false; 00039 } 00040 00041 uint_fast8_t stringLength = 0, byteCount = 0; 00042 00043 //Iterate over each component of the IP. The exit condition is in the middle of the loop 00044 while (true) { 00045 00046 //No valid character (IPv4 addresses don't have implicit 0, that is x.y..z being read as x.y.0.z) 00047 if (stringLength == len || ip4addr[stringLength] < '0' || ip4addr[stringLength] > '9') { 00048 return false; 00049 } 00050 00051 //For each component, we convert it to the raw value 00052 uint_fast16_t byte = 0; 00053 while (stringLength < len && ip4addr[stringLength] >= '0' && ip4addr[stringLength] <= '9') { 00054 byte *= 10; 00055 byte += ip4addr[stringLength++] - '0'; 00056 00057 //We go over the maximum value for an IPv4 component 00058 if (byte > 0xff) { 00059 return false; 00060 } 00061 } 00062 00063 //Append the component 00064 addr[byteCount++] = (uint8_t) byte; 00065 00066 //If we're at the end, we leave the loop. It's the only way to reach the `true` output 00067 if (byteCount == 4) { 00068 break; 00069 } 00070 00071 //If the next character is invalid, we return false 00072 if (stringLength == len || ip4addr[stringLength++] != '.') { 00073 return false; 00074 } 00075 } 00076 00077 return stringLength == len || ip4addr[stringLength] == '\0'; 00078 } 00079 #endif
Generated on Fri Jul 15 2022 22:55:10 by
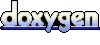