Fork for fixes
Embed:
(wiki syntax)
Show/hide line numbers
UipEthernet.h
00001 /* 00002 UipEthernet.h - Arduino implementation of a UIP wrapper class. 00003 Copyright (c) 2013 Norbert Truchsess <norbert.truchsess@t-online.de> 00004 All rights reserved. 00005 00006 Modified (ported to mbed) by Zoltan Hudak <hudakz@inbox.com> 00007 00008 This program is free software: you can redistribute it and/or modify 00009 it under the terms of the GNU General Public License as published by 00010 the Free Software Foundation, either version 3 of the License, or 00011 (at your option) any later version. 00012 00013 This program is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 GNU General Public License for more details. 00017 00018 You should have received a copy of the GNU General Public License 00019 along with this program. If not, see <http://www.gnu.org/licenses/>. 00020 */ 00021 #ifndef UIPETHERNET_h 00022 #define UIPETHERNET_h 00023 00024 #define UIPETHERNET_DEBUG 00025 //#define UIPETHERNET_DEBUG_CHKSUM 00026 #define UIPETHERNET_DEBUG_UDP 00027 #define UIPETHERNET_DEBUG_CLIENT 00028 #define UIP_LOGGING 1 00029 #include "mbed.h" 00030 #include "DhcpClient.h" 00031 #include "IpAddress.h" 00032 #include "utility/Enc28j60Eth.h" 00033 #include "TcpClient.h" 00034 #include "TcpServer.h" 00035 #include "UdpSocket.h" 00036 00037 extern "C" 00038 { 00039 #include "utility/uip_timer.h" 00040 #include "utility/uip.h" 00041 #include "utility/util.h" 00042 } 00043 00044 class UipEthernet 00045 { 00046 public: 00047 static UipEthernet* ethernet; 00048 static IpAddress dnsServerAddress; 00049 Enc28j60Eth enc28j60Eth; 00050 00051 UipEthernet (const uint8_t mac[6], PinName mosi, PinName miso, PinName sck, PinName cs); 00052 00053 int connect(unsigned long timeout = 60); 00054 void disconnect(); 00055 void set_network(uint8_t octet1, uint8_t octet2, uint8_t octet3, uint8_t octet4); 00056 void set_network(IpAddress ip); 00057 void set_network(IpAddress ip, IpAddress dns); 00058 void set_network(IpAddress ip, IpAddress dns, IpAddress gateway); 00059 void set_network(IpAddress ip, IpAddress dns, IpAddress gateway, IpAddress subnet); 00060 void set_network(const char *ip_address, const char *netmask, const char *gateway); 00061 void tick(); 00062 IpAddress localIP(); 00063 IpAddress subnetMask(); 00064 IpAddress gatewayIP(); 00065 IpAddress dnsServerIP(); 00066 const char* get_ip_address(); 00067 void get_ip_address(SocketAddress* addr); 00068 const char* get_netmask(); 00069 void get_netmask(SocketAddress* addr); 00070 const char* get_gateway(); 00071 void get_gateway(SocketAddress* addr); 00072 const char* get_mqttFromDhcp(); 00073 static uint16_t chksum(uint16_t sum, const uint8_t* data, uint16_t len); 00074 static uint16_t ipchksum(); 00075 bool stoip4(const char *ip4addr, size_t len, void *dest); 00076 private: 00077 uint8_t *const _mac; 00078 IpAddress _ip; 00079 IpAddress _dns; 00080 IpAddress _gateway; 00081 IpAddress _subnet; 00082 static memhandle inPacket; 00083 static memhandle uipPacket; 00084 static uint8_t uipHeaderLen; 00085 static uint8_t packetState; 00086 DhcpClient dhcpClient; 00087 Timer periodicTimer; 00088 void init(const uint8_t* mac); 00089 bool network_send(); 00090 friend class TcpServer; 00091 friend class TcpClient; 00092 friend class UdpSocket; 00093 #if UIP_UDP 00094 uint16_t upper_layer_chksum(uint8_t proto); 00095 #endif 00096 friend uint16_t uip_ipchksum(); 00097 friend uint16_t uip_tcpchksum(); 00098 friend uint16_t uip_udpchksum(); 00099 friend void uipclient_appcall(); 00100 friend void uipudp_appcall(); 00101 00102 #if UIP_CONF_IPV6 00103 uint16_t uip_icmp6chksum (); 00104 #endif 00105 }; 00106 00107 #endif
Generated on Fri Jul 15 2022 22:55:10 by
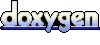